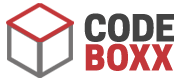

Javascript Text To Speech (Simple Examples)
Table of contents, download & notes, example code download, sorry for the ads....
But someone has to pay the bills, and sponsors are paying for it. I insist on not turning Code Boxx into a "paid scripts" business, and I don't "block people with Adblock". Every little bit of support helps.
Buy Me A Coffee Code Boxx eBooks
JAVASCRIPT TEXT TO SPEECH
Tutorial video, 1) simple text to speech, 1a) the html, 1b) the javascript, 1c) the demo, 2) choosing a voice, 2a) the html, 2b) the javascript, 2c) the demo, 3) more controls – volume, pitch, rate, 3a) the html, 3b) the javascript, 3c) the demo, compatibility checks, links & references.
Thank you for reading, and we have come to the end. I hope that it has helped you to better understand, and if you want to share anything with this guide, please feel free to comment below. Good luck and happy coding!
Leave a Comment Cancel Reply
- About AssemblyAI
JavaScript Text-to-Speech - The Easy Way
Learn how to build a simple JavaScript Text-to-Speech application using JavaScript's Web Speech API in this step-by-step beginner's guide.

Contributor
When building an app, you may want to implement a Text-to-Speech feature for accessibility, convenience, or some other reason. In this tutorial, we will learn how to build a very simple JavaScript Text-to-Speech application using JavaScript's built-in Web Speech API .
For your convenience, we have provided the code for this tutorial application ready for you to fork and play around with over at Replit , or ready for you to clone from Github . You can also view a live version of the app here .
Step 1 - Setting Up The App
First, we set up a very basic application using a simple HTML file called index.html and a JavaScript file called script.js .
We'll also use a CSS file called style.css to add some margins and to center things, but it’s entirely up to you if you want to include this styling file.
The HTML file index.html defines our application's structure which we will add functionality to with the JavaScript file. We add an <h1> element which acts as a title for the application, an <input> field in which we will enter the text we want spoken, and a <button> which we will use to submit this input text. We finally wrap all of these objects inside of a <form> . Remember, the input and the button have no functionality yet - we'll add that in later using JavaScript.
Inside of the <head> element, which contains metadata for our HTML file, we import style.css . This tells our application to style itself according to the contents of style.css . At the bottom of the <body> element, we import our script.js file. This tells our application the name of the JavaScript file that stores the functionality for the application.
Now that we have finished the index.html file, we can move on to creating the script.js JavaScript file.
Since we imported the script.js file to our index.html file above, we can test its functionality by simply sending an alert .
To add an alert to our code, we add the line of code below to our script.js file. Make sure to save the file and refresh your browser, you should now see a little window popping up with the text "It works!".
If everything went ok, you should be left with something like this:

Step 2 - Checking Browser Compatibility
To create our JavaScript Text-to-Speech application, we are going to utilize JavaScript's built-in Web Speech API. Since this API isn’t compatible with all browsers, we'll need to check for compatibility. We can perform this check in one of two ways.
The first way is by checking our operating system and version on caniuse.com .
The second way is by performing the check right inside of our code, which we can do with a simple conditional statement:
This is a shorthand if/else statement, and is equivalent to the following:
If you now run the app and check your browser console, you should see one of those messages. You can also choose to pass this information on to the user by rendering an HTML element.
Step 3 - Testing JavaScript Text-to-Speech
Next up, let’s write some static code to test if we can make the browser speak to us.
Add the following code to the script.js file.
Code Breakdown
Let’s look at a code breakdown to understand what's going on:
- With const synth = window.speechSynthesis we declare the synth variable to be an instance of the SpeechSynthesis object, which is the entry to point to using JavaScript's Web Speech API. The speak method of this object is what ultimately converts text into speech.
- let ourText = “Hey there what’s up!!!!” defines the ourText variable which holds the string of text that we want to be uttered.
- const utterThis = new SpeechSynthesisUtterance(ourText) defines the utterThis variable to be a SpeechSynthesisUtterance object, into which we pass ourText .
- Putting it all together, we call synth.speak(utterThis) , which utters the string inside ourText .
Save the code and refresh the browser window in which your app runs in order to hear a voice saying “ Hey there what’s up!!!! ”.
Step 4 - Making Our App Dynamic
Our code currently provides us with a good understanding of how the Text-to-Speech aspect of our application works under the hood, but the app at this point only converts the static text which we defined with ourText into speech. We want to be able to dynamically change what text is being converted to speech when using the application. Let’s do that now utilizing a <form> .
- First, we add the const textInputField = document.querySelector("#text-input") variable, which allows us to access the value of the <input> tag that we have defined in the index.html file in our JavaScript code. We select the <input> field by its id: #text-input .
- Secondly, we add the const form = document.querySelector("#form") variable, which selects our form by its id #form so we can later submit the <form> using the onsubmit function.
- We initialize ourText as an empty string instead of a static sentence.
- We wrap our browser compatibility logic in a function called checkBrowserCompatibility and then immediately call this function.
Finally, we create an onsubmit handler that executes when we submit our form. This handler does several things:
- event.preventDefault() prevents the browser from reloading after submitting the form.
- ourText = textInputField.value sets our ourText string to whatever we enter in the "input" field of our application.
- utterThis.text = ourText sets the text to be uttered to the value of ourText .
- synth.speak(utterThis) utters our text string.
- textInputField.value resets the value of our input field to an empty string after submitting the form.
Step 5 - Testing Our JavaScript Text-to-Speech App
To test our JavaScript Text-to-Speech application, simply enter some text in the input field and hit “Submit” in order to hear the text converted to speech.
Additional Features
There are a lot of properties that can be modified when working with the Web Speech API. For instance:
You can try playing around with these properties to tailor the application to your needs.
This simple example provides an outline of how to use the Web Speech API for JavaScript Text-to-Speech .
While Text-to-Speech is useful for accessibility, convenience, and other purposes, there are a lot of use-cases in which the opposite functionality, i.e. Speech-to-Text, is useful. We have built a couple of example projects using AssemblyAI’s Speech-to-Text API that you can check out for those who want to learn more.
Some of them are:
- React Speech Recognition with React Hooks
- How To Convert Voice To Text Using JavaScript
Popular posts
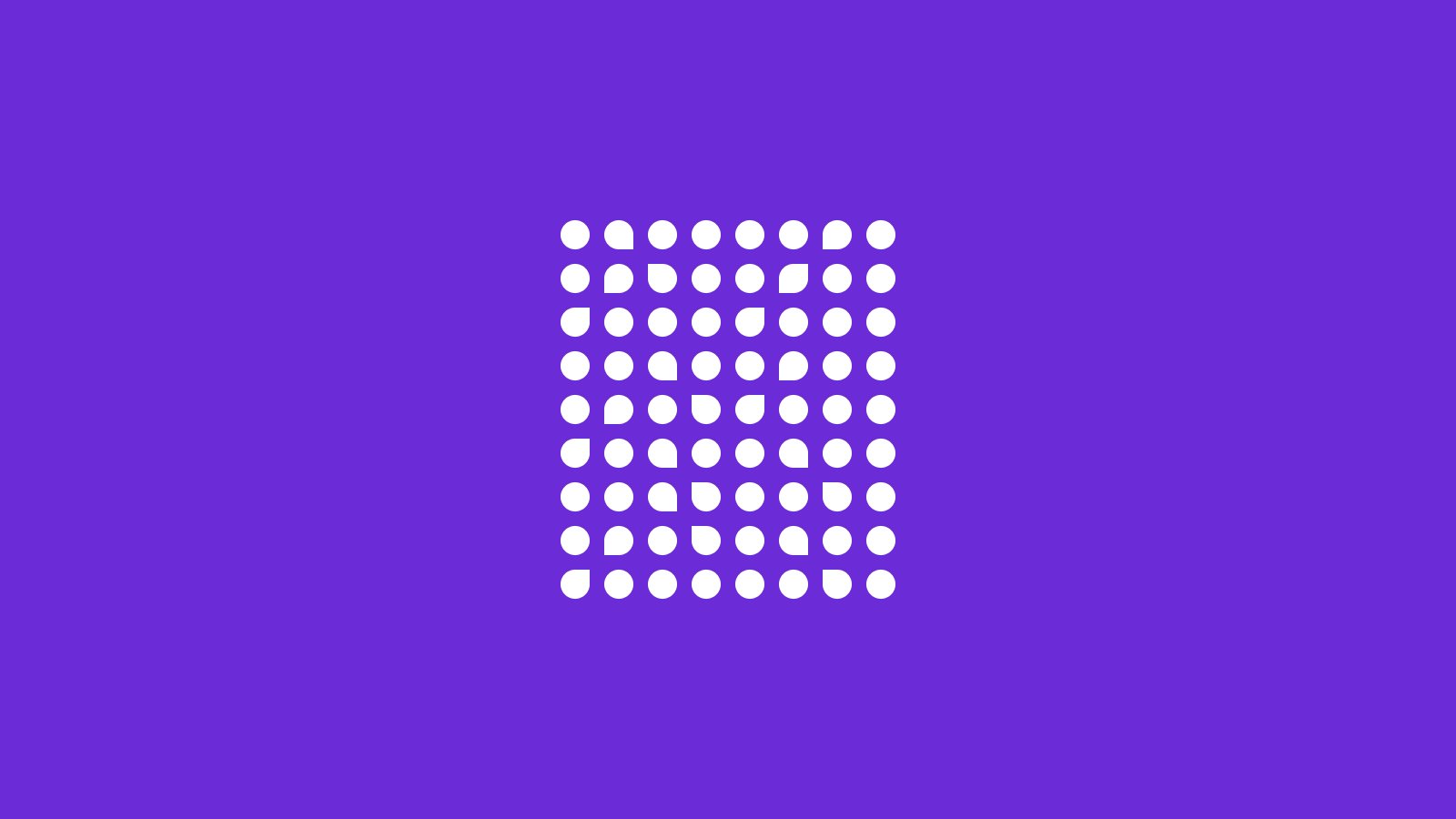
AI trends in 2024: Graph Neural Networks

Developer Educator at AssemblyAI
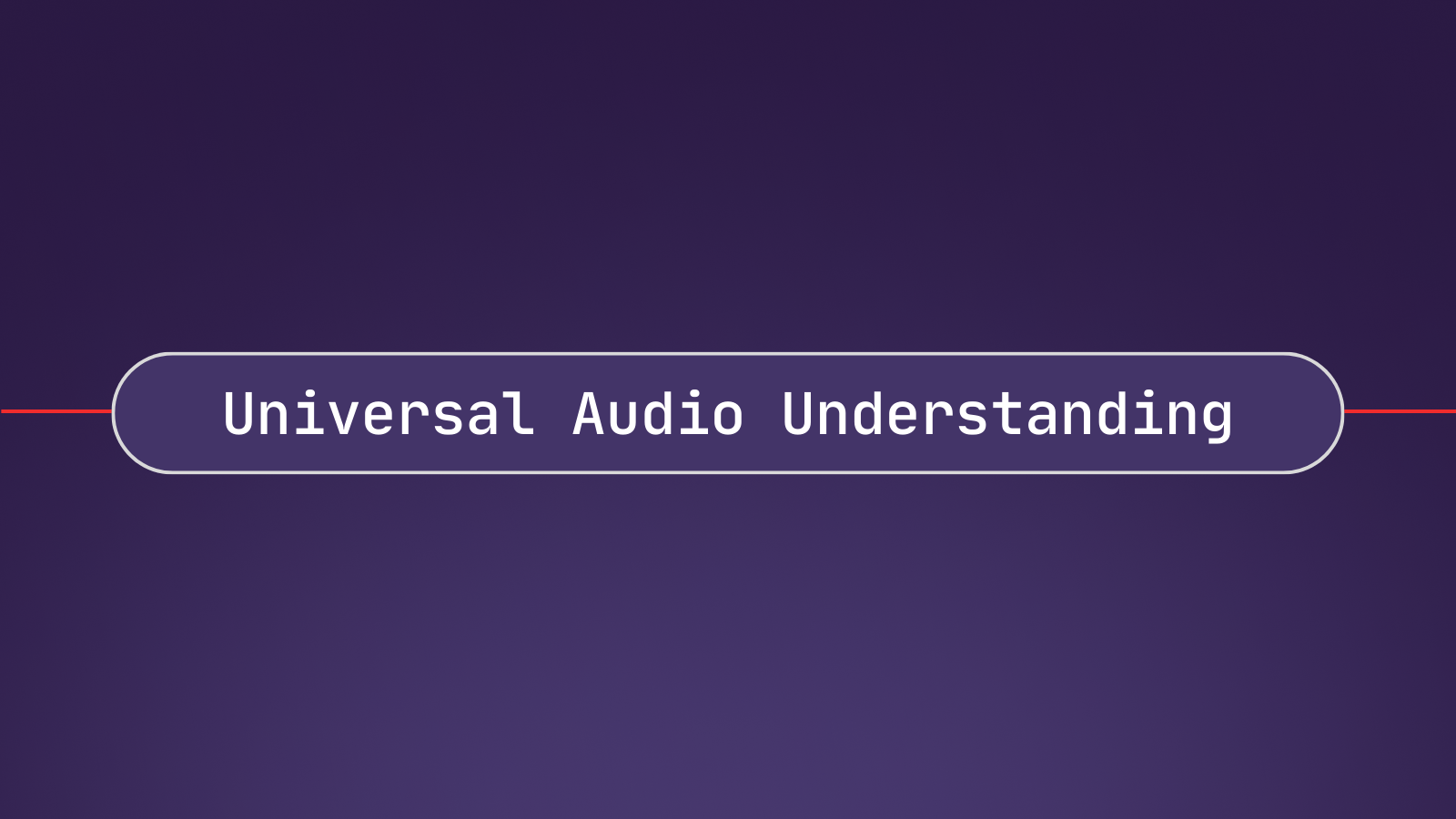
AI for Universal Audio Understanding: Qwen-Audio Explained

Combining Speech Recognition and Diarization in one model
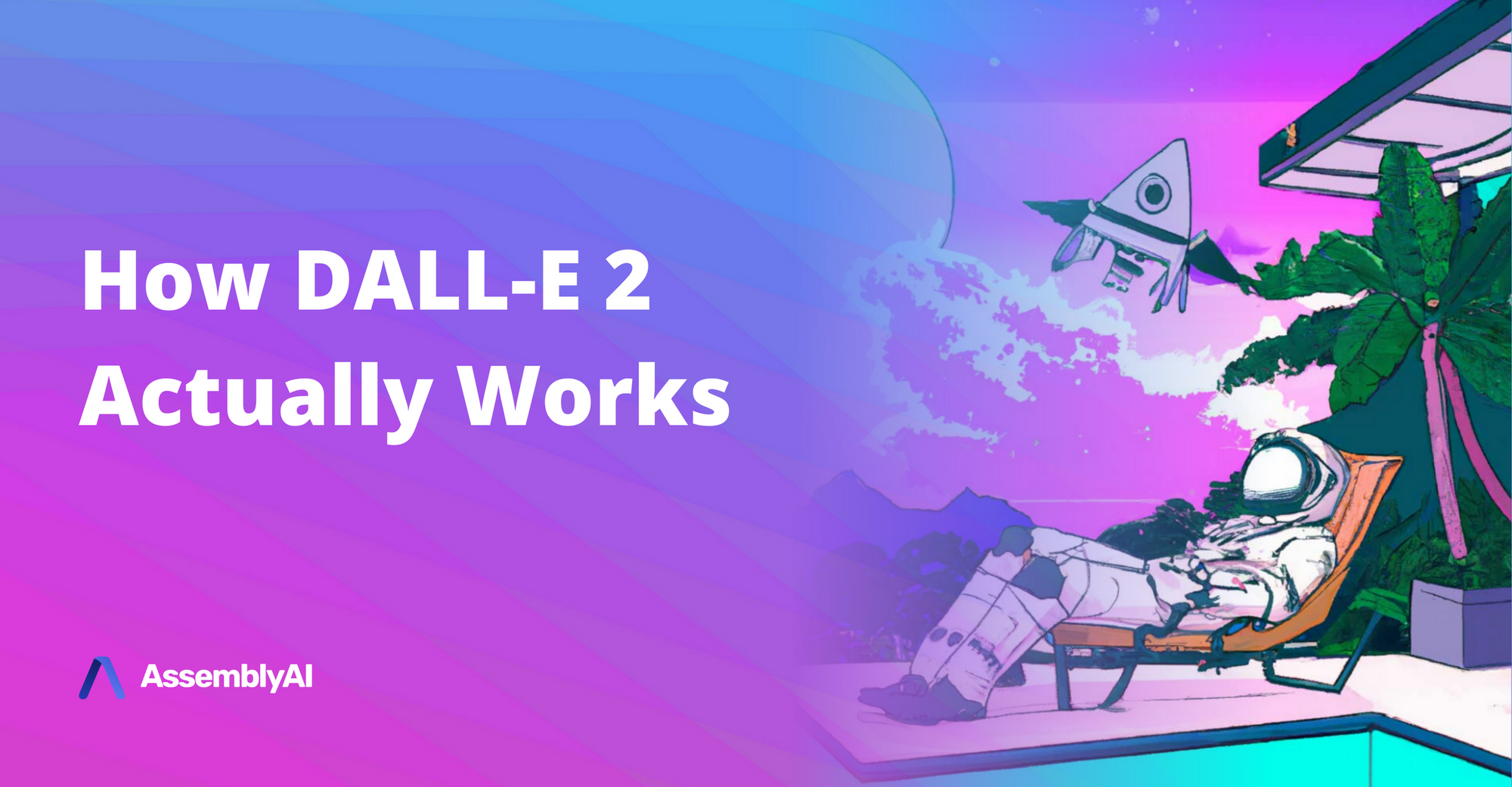
How DALL-E 2 Actually Works
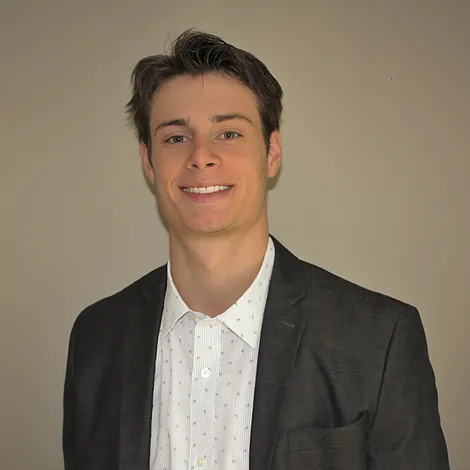
Javascript Text To Speech: A Guide To Converting TTS With Javascript
Want to add a unique feature to your website? Learn how to integrate Javascript text-to-speech capabilities with this helpful guide.
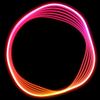
Unreal Speech
Javascript text to speech technology provides a unique digital experience by converting written content into spoken words. By leveraging Javascript text to speech, developers gain access to an array of features that can enhance user interaction and engagement. When applying text to speech technology, developers can implement accessibility features like screen readers, voice commands, and more. Through this technology, developers can bring an innovative and interactive dimension to their digital applications. Embrace the possibilities of Javascript text to speech and explore the endless potential it holds for your projects.
Table of Contents
Introduction to javascript text to speech (tts), understanding javascript tts apis, getting started with javascript text to speech, customizing the speech to your preferences, best practices for javascript tts development, try unreal speech for free today — affordably and scalably convert text into natural-sounding speech with our text-to-speech api.
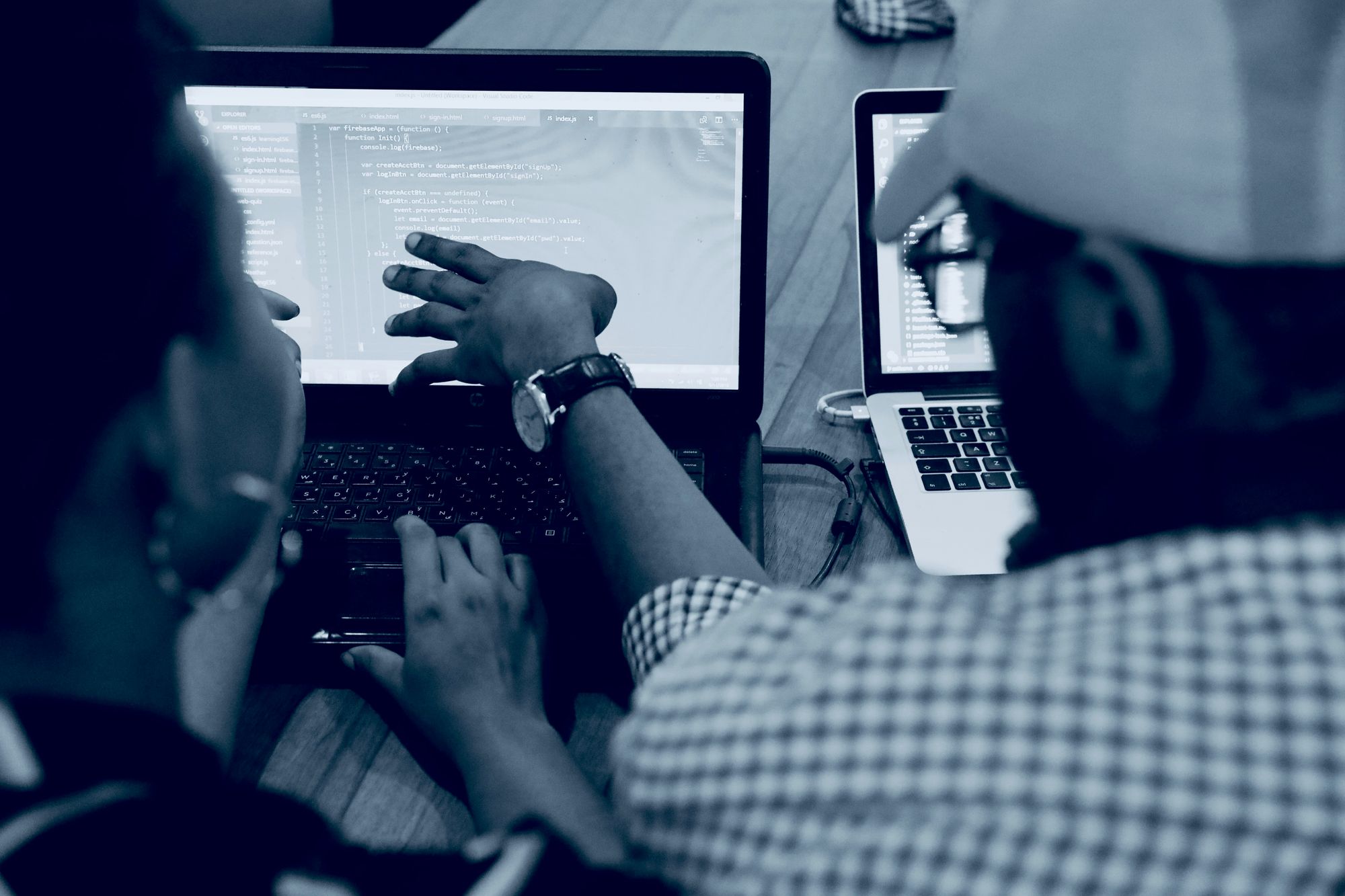
Text-to-Speech (TTS) technology enables computers to convert written text into spoken words. In the context of JavaScript, TTS allows developers to integrate speech synthesis capabilities directly into web applications. With TTS, users can interact with websites and applications using voice commands and receive audible feedback.
Importance of TTS in Web Development
TTS plays a crucial role in enhancing the accessibility and usability of web content. It enables users with visual impairments or reading difficulties to access online information more effectively by listening to the content instead of reading it. TTS can provide a more engaging and interactive user experience, especially in applications that require hands-free operation or communication with users in diverse environments. Integrating TTS into web development projects can improve inclusivity, usability, and overall user satisfaction.
Cutting-Edge and Cost-Effective Text-to-Speech Solution
Unreal Speech offers a low-cost, highly scalable text-to-speech API with natural-sounding AI voices, which is the cheapest and most high-quality solution in the market . Unreal Speech can cut your text-to-speech costs by up to 90%. Get human-like AI voices with their super-fast, low-latency API, with the option for per-word timestamps. The simple, easy-to-use API allows you to give your LLM a voice with ease and offer this functionality at scale. If you are looking for cheap, scalable, realistic TTS to incorporate into your products, try our text-to-speech AP I for free today. Convert text into natural-sounding speech at an affordable and scalable price.
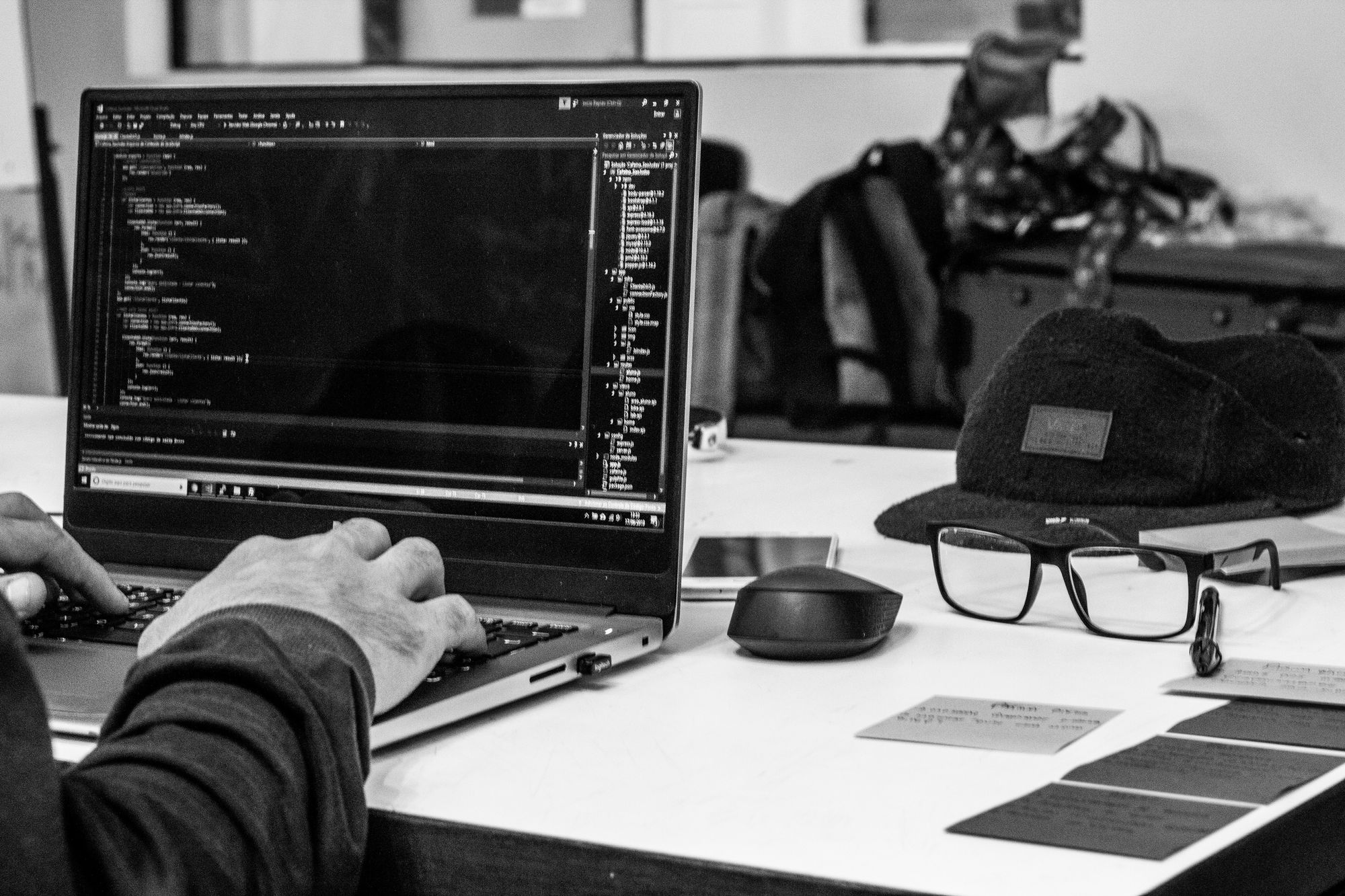
Web Speech API Overview
The Web Speech API provides a straightforward way to incorporate text-to-speech functionality into web applications using JavaScript. With just a few lines of code, developers can enable their browsers to convert text into speech. This API opens up a plethora of opportunities for enhancing user experiences and accessibility on the web.
TTS Functionality with Web Speech API
The Web Speech API offers developers various methods and functions to synthesize speech from plain text. Developers can further control speech synthesis parameters like voice selection and rate and handle related events effectively. While the Web Speech API's functionality largely depends on the voices available in the browser or the user's operating system, it provides a quick and cost-effective solution for integrating TTS capabilities into web applications.
Browser Compatibility and Support
Although the Web Speech API is supported by many modern web browsers such as Chrome, Firefox, and Safari, developers should be mindful of discrepancies in implementation across different platforms. Ensuring consistent behavior and fallback options is crucial for a seamless user experience. Testing browser compatibility and having fallback options ready can help maintain accessibility and functionality across various devices and platforms.
Affordable and Scalable Text-to-Speech Solution
If you are looking for cheap, scalable, realistic TTS to incorporate into your products, try our text-to-speech API for free today. Convert text into natural-sounding speech at an affordable and scalable price.
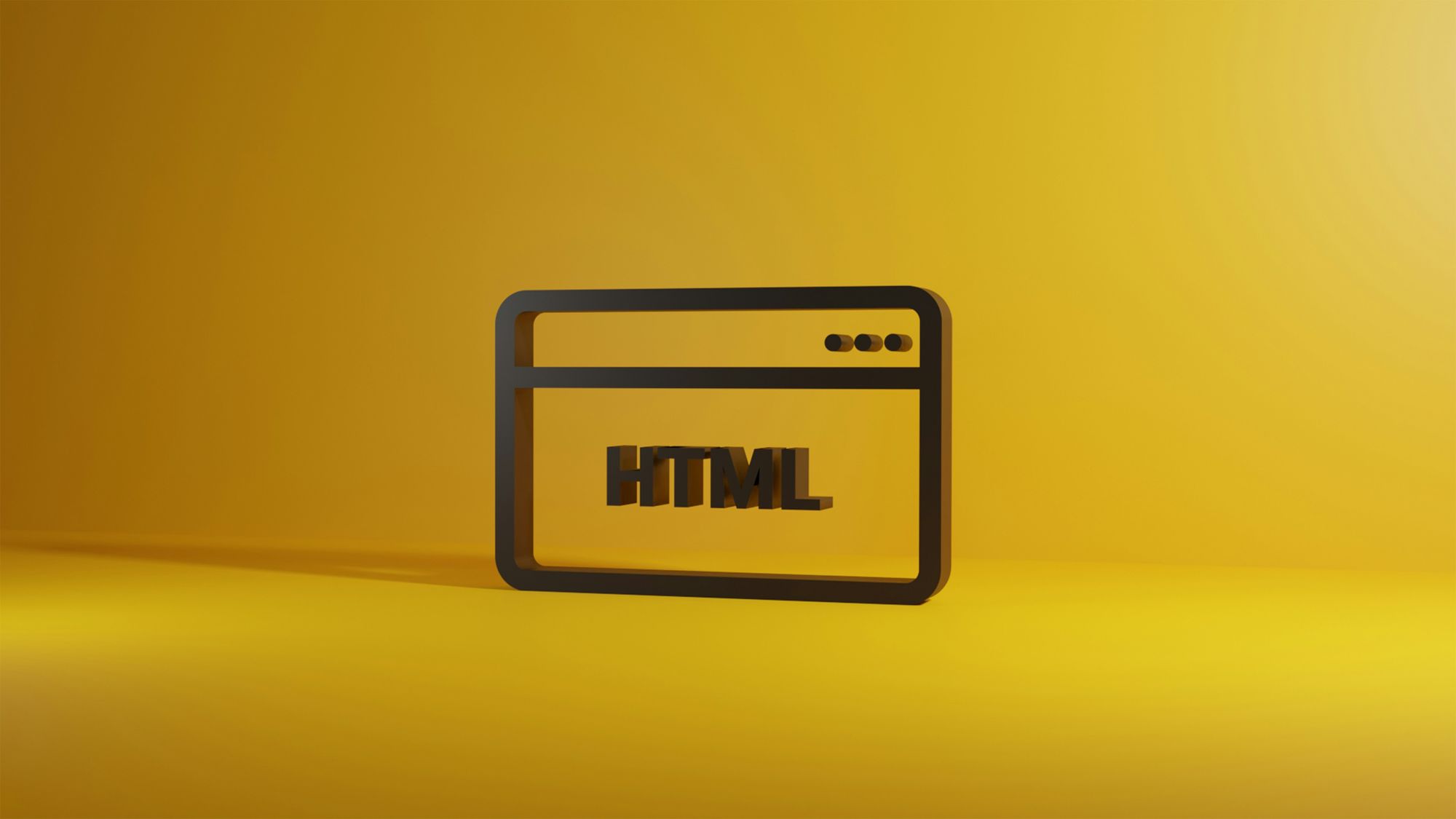
To utilize the Web Speech API for text-to-speech functionality in your JavaScript project, you need to include the necessary scripts in your HTML file. This typically involves referencing the SpeechSynthesis interface provided by the Web Speech API.
The speechSynthesis Interface
Begin by creating an HTML file and linking your JavaScript file using the script src tag. In your JavaScript file, initialize the speech synthesis object and set up an event listener for when the voices are ready. ```javascript const synth = window.speechSynthesis; // Wait for voices to be loaded synth.onvoiceschanged = () => { const voices = synth.getVoices(); // Do something with the available voices }; ``` Once the voices are loaded, you can access them using the synth.getVoices() method. This will return a list of available voices that you can use for speech synthesis. You can loop through the voices using forEach and display them in your HTML. ```javascript const voiceSelect = document.getElementById(‘voice-select’); voices.forEach((voice) => { const option = document.createElement(‘option’); option.textContent = ${voice.name} (${voice.lang}); option.setAttribute(‘value’, voice.lang); voiceSelect.appendChild(option); }); ``` Next, you can create a function to synthesize speech from the selected voice. This function takes the text input from a textarea element and uses the selected voice to generate speech. ```javascript const speak = () => { const text = document.getElementById(‘text-input’).value; const voice = voices[voiceSelect.selectedIndex]; const utterance = new SpeechSynthesisUtterance(text); utterance.voice = voice; synth.speak(utterance); }; ``` Add an event listener to the button or form submit to trigger the speak function. ```javascript const button = document.getElementById(‘speak-button’); button.addEventListener(‘click’, speak);
/ ``` With these few lines of code, you can convert text to speech in real-time. If you are looking for cheap, scalable, realistic TTS to incorporate into your products, try our text-to-speech API for free today. Convert text into natural-sounding speech at an affordable and scalable price.
To customize the speech rate, pitch, and volume with the Javascript text to speech, you can set properties on the SpeechSynthesisUtterance object. For the rate, a decimal value between 0.1 (slowest) and 10 (fastest) can be set, with 1 being the default value. The pitch, on the other hand, is a decimal value between 0 (lowest) and 2 (highest; almost audible only for felines), with 1 being the default value. For a decent speech quality , you can customize these values with an example shown below:
- utterance.rate = 0.8;
- utterance.pitch = 1;
- utterance.volume = 1;
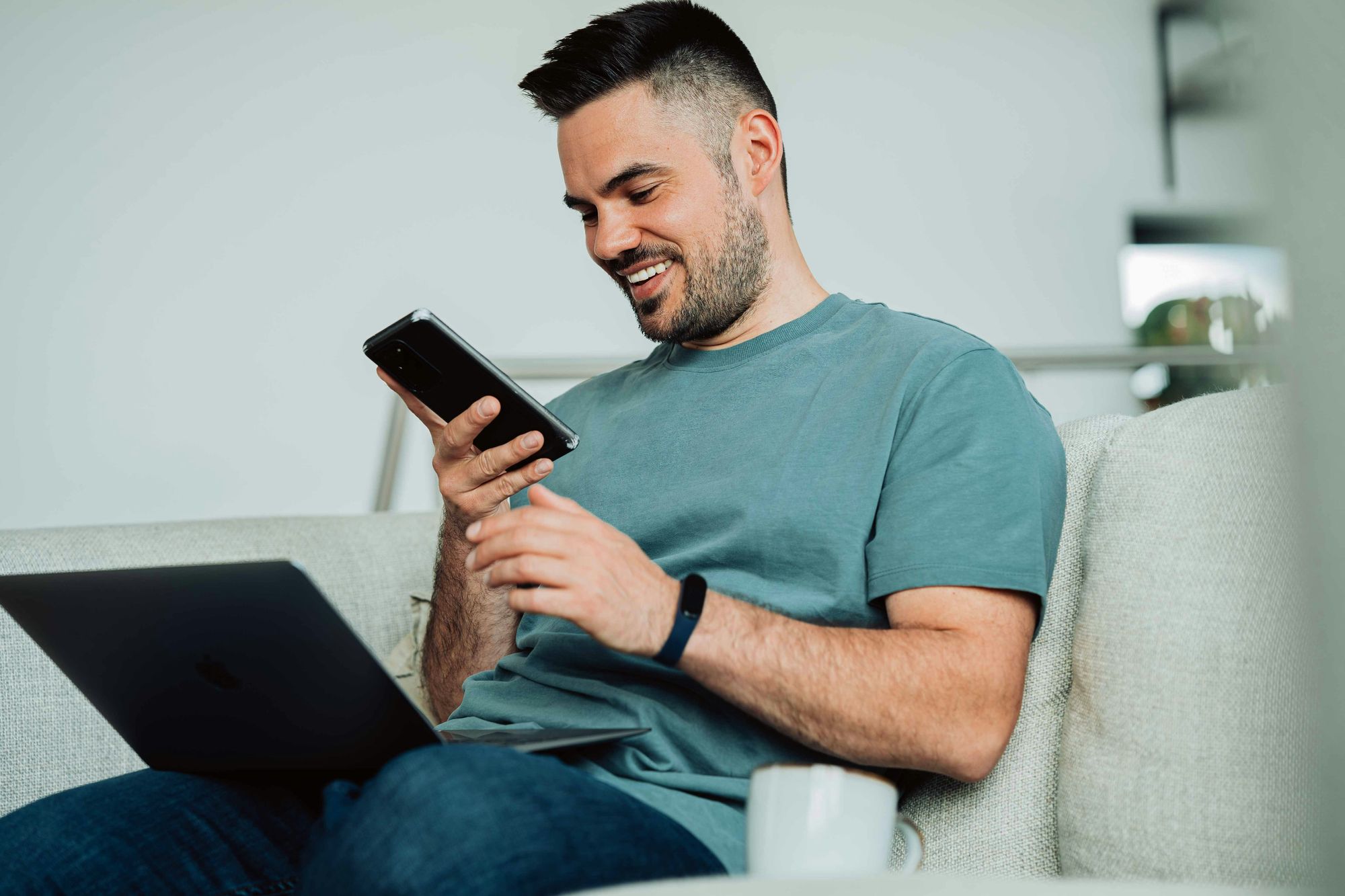
Efficient Algorithms for Text-to-Speech Processing
When developing a JavaScript TTS system, I always make sure to implement efficient algorithms that can handle large volumes of text quickly and accurately. By using efficient algorithms, I can drastically reduce latency and resource consumption, resulting in a smoother and more responsive user experience.
Caching Frequently Used Data for Improved Performance
Caching frequently accessed or processed text data is vital for optimizing the performance of my JavaScript TTS applications. By storing commonly used text data in a cache, I can avoid repetitive computations during speech synthesis, thereby reducing the overhead and enhancing the overall efficiency of the system.
Testing Across Multiple Browsers for Compatibility
One of the key best practices I follow for JavaScript TTS development is to ensure that my implementation works seamlessly across various web browsers and versions. By validating my JavaScript TTS code across multiple browsers, I can identify and address any compatibility issues, guaranteeing a consistent user experience across different platforms.
Handling Browser-Specific Behavior with Fallbacks
To account for differences in browser behavior and feature support, I always incorporate fallback mechanisms or polyfills in my JavaScript TTS applications. By doing so, I can ensure that my TTS system functions correctly even in browsers that lack full support for the Web Speech API, thereby enhancing the overall reliability and robustness of the application.
Comprehensive Testing for Enhanced TTS Functionality
Thorough testing is a crucial aspect of developing a successful JavaScript TTS system. I test my TTS functionality across a wide range of scenarios, including varying text inputs, speech settings, and user interactions. Through comprehensive testing, I can identify and address any potential issues or limitations, ensuring that my JavaScript TTS implementation delivers a seamless and flawless user experience.
Unreal Speech is a game-changer in the world of text-to-speech API solutions. By using Unreal Speech, you can experience a drastic reduction in the costs associated with text-to-speech, potentially up to a whopping 90%. A unique aspect of Unreal Speech is its capability to provide you with a highly scalable text-to-speech API. Thus, whether your project is small or large-scale, Unreal Speech has your back. And the cherry on top? The quality of the speech generated by this tool is top-notch, with AI voices that sound very natural. These voices are so close to human-like that you might have to remind yourself that it's actually a machine speaking!
Precision and Ease of Use with Per-Word Timestamps
Unreal Speech also boasts a feature that is incredibly beneficial for many projects: per-word timestamps. Developers like me often need such precision when it comes to generating speech from text. Thus, having per-word timestamps as a feature is not only convenient but also a necessity. Speaking of convenience, Unreal Speech is designed with a simple API that is easy to use. In Javascript Text-to-Speech, I can vouch for the fact that a simple interface is a blessing when working on projects that involve generating speech.
Unreal Speech's Fast Operation
Another amazing feature of Unreal Speech is the speed at which it operates. With super low latency, you can expect the tool to work fast, getting you your results in no time. This is a huge plus point, particularly when working on time-sensitive projects. Therefore, if you’re looking for an affordable and scalable text-to-speech solution that offers high-quality output and is easy to integrate into your products, Unreal Speech is the way to go. Give it a try today!
How to Convert Text to Speech using HTML, CSS and JavaScript
By Faraz - September 28, 2022
Learn how to implement text to speech in JavaScript using Speech Synthesis API. Follow our step-by-step guide and add this exciting feature to your website!
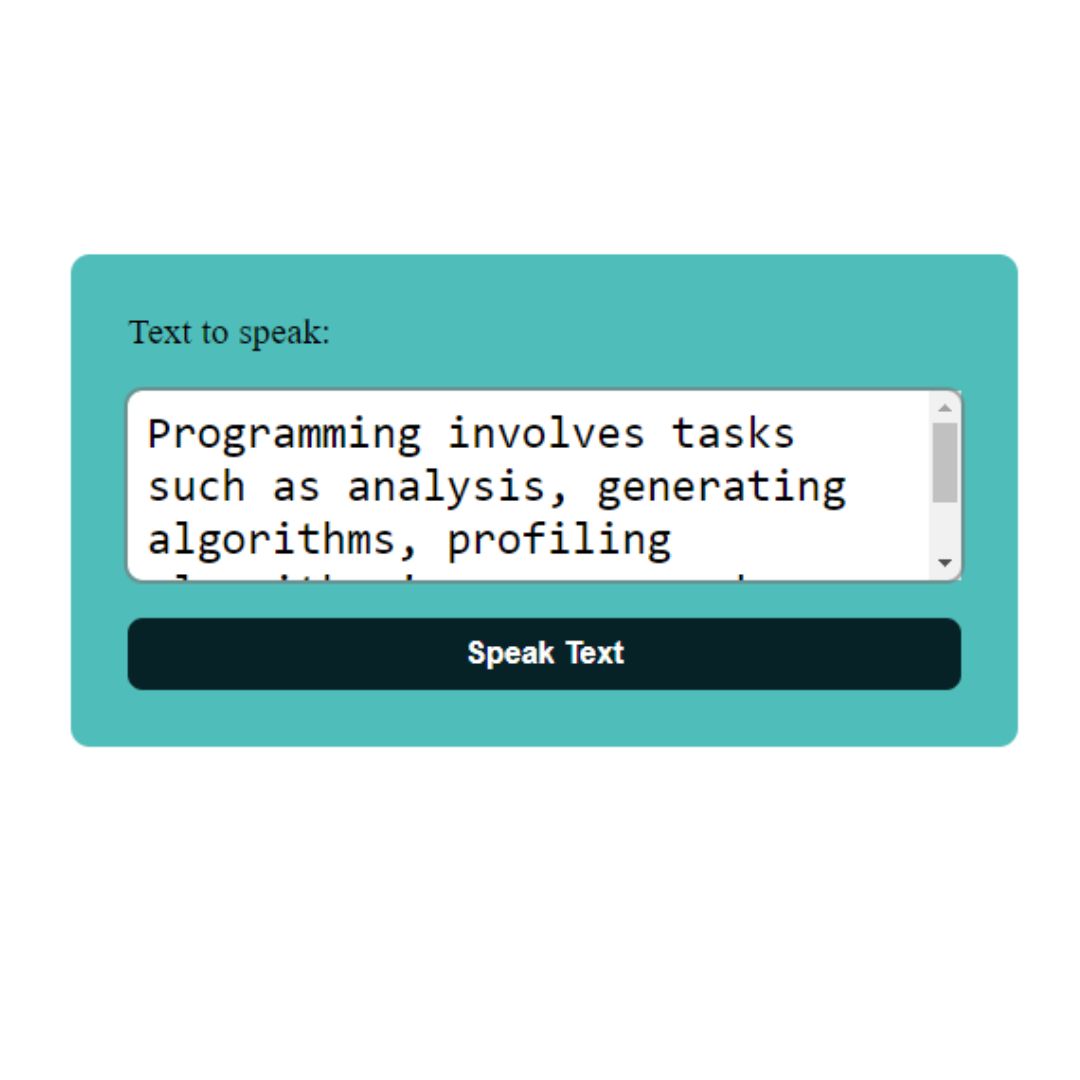
Table of Contents
- Project Introduction
- JavaScript Code
Welcome to our guide on how to convert text to speech in JavaScript using the Speech Synthesis API. Text-to-speech technology has become increasingly popular, and implementing it on your website can improve the user experience and accessibility for users with hearing impairments or learning disabilities. In this guide, we will cover everything you need to know to add this exciting feature to your website. We'll start with an introduction to text-to-speech and its importance, followed by an overview of the Speech Synthesis API and its features and limitations.
The Speech Synthesis API is a web browser API that enables developers to generate speech from the text on a webpage. The API provides a range of settings that can be used to customize the speech output, such as voice, pitch, rate, and volume.
One of the key features of the Speech Synthesis API is that it is easy to use and requires only a few lines of code to get started. It is also supported by most modern web browsers, making it a reliable choice for adding text to speech functionality to a website.
However, there are some limitations to the Speech Synthesis API. For example, the available voices and languages depend on the user's operating system and browser. Additionally, the quality of the speech output can vary depending on the voice used, and some voices may not sound natural or be easy to understand.
Despite these limitations, the Speech Synthesis API remains a powerful tool for adding text to speech functionality to a website, and it can greatly enhance the user experience for many users.
When creating an application, you may want to enable text-to-speech features for accessibility, convenience, or some other reason. In this tutorial, we'll learn how to create a very simple JavaScript text-to-speech application using JavaScript's built-in Web Speech API.
Watch full tutorial on my YouTube Channel: Watch Here .
Let's start making an amazing text-to-speech converter Using HTML, CSS, and JavaScript step by step.
Join My Telegram Channel to Download the Project: Click Here
Prerequisites:
Before starting this tutorial, you should have a basic understanding of HTML, CSS, and JavaScript. Additionally, you will need a code editor such as Visual Studio Code or Sublime Text to write and save your code.
Source Code
Step 1 (HTML Code):
To get started, we will first need to create a basic HTML file. In this file, we will include the main structure for our text to speech converter.
The first line of the code, , declares the document type as HTML. This line is required for all HTML documents.
The html tag wraps around the entire document, and the lang attribute specifies the language of the document, in this case, English.
The head section contains meta information about the document, including the character encoding, which is set to UTF-8, and the viewport, which defines how the website should be displayed on different devices. It also includes a title tag which sets the title of the document, which appears on the tab of the browser.
In the head section, there is a link to an external stylesheet, which is used to define the visual styling of the document, and a script tag that includes an external JavaScript file. The type attribute in the script tag is set to "module" to indicate that the script uses ES6 modules.
The body section is where the actual content of the web page is contained. In this case, it consists of a div element with a class of "container" that wraps around a label and a textarea element. The label tag is used to associate a label with an input element, and the textarea is where the user can enter the text they want to be spoken. There is also a button element with an id attribute of "speak" that triggers the text-to-speech functionality when clicked.
After creating the files just paste the following below codes into your file. Make sure to save your HTML document with a .html extension, so that it can be properly viewed in a web browser.
This is the basic structure of our text to speech converter using HTML, and now we can move on to styling it using CSS.
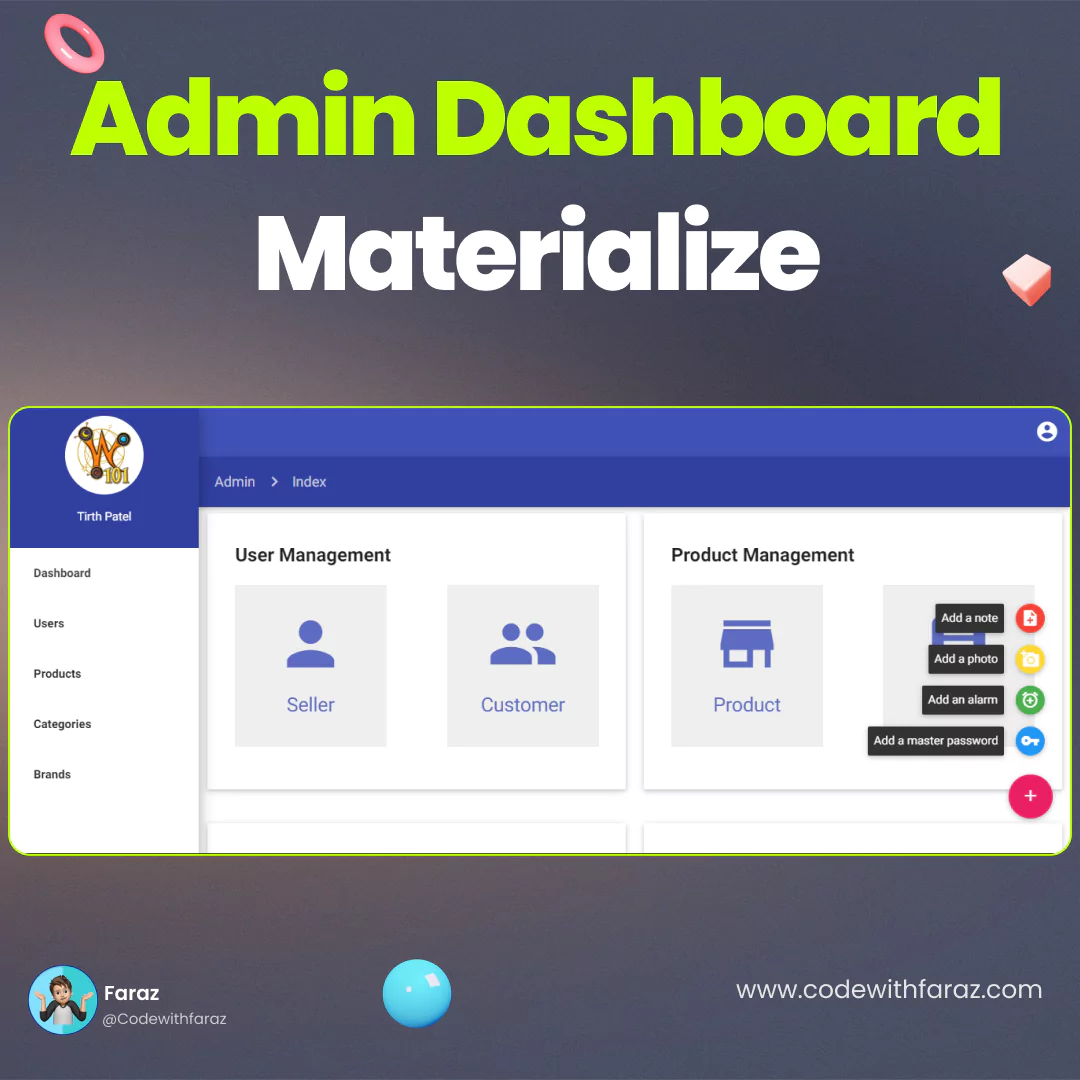
Step 2 (CSS Code):
Once the basic HTML structure of the text to speech converter is in place, the next step is to add styling to the text to speech converter using CSS.
Next, we will create our CSS file. In this file, we will use some basic CSS rules to create our text to speech converter.
The first section, *{} , is a universal selector that applies to all elements on the webpage. It sets the box-sizing property to border-box, which includes any padding and border in the element's total width and height, and sets the margin of all elements to 0, removing any default margin.
The body selector sets the display property to flex, which creates a flexible container for the page's content. The justify-content and align-items properties are set to center, which horizontally and vertically centers the content in the container. The min-height property is set to 100vh, which makes the container take up the entire viewport height.
The .container selector applies styles to a specific div element with a class of "container". It sets the background-color to #4FBDBA, which is a light blue color. The display property is set to grid, which allows the container to be laid out in a grid structure. The gap property sets the gap between grid items to 20 pixels. The width is set to 500 pixels, and the max-width property uses a calc() function to set the maximum width to be the width of the viewport minus 40 pixels. The padding property sets the padding inside the container, while the border-radius property sets the rounded corners of the container. The font-size property sets the size of the text inside the container.
The #text selector applies styles to a specific textarea element with an id of "text". It sets the display property to block, which makes the element take up the entire width of its container. The height is set to 100 pixels, while the border-radius property sets the rounded corners of the element. The font-size property sets the size of the text inside the element, while the border property sets the border around the element. The resize property is set to none, which disables resizing of the element by the user. The padding property sets the padding inside the element, while the outline property sets a visible border around the element when it is in focus.
The button selector applies styles to all button elements. It sets the padding inside the button, while the background property sets the background color of the button. The color property sets the color of the text inside the button, while the border-radius property sets the rounded corners of the button. The cursor property sets the type of cursor that appears when the button is hovered over. The border property sets the border around the button, while the font-size and font-weight properties set the size and weight of the text inside the button.
This will give our text to speech converter an upgraded presentation. Create a CSS file with the name of styles.css and paste the given codes into your CSS file. Remember that you must create a file with the .css extension.
Step 3 (JavaScript Code):
Finally, we need to create a speechSynthesis function in JavaScript.
The code uses the Document Object Model (DOM) to select and manipulate HTML elements, and it uses the Web Speech API to synthesize speech from text.
The first line of the code uses the getElementById method to select an HTML element with an id of "text". The textEL variable is then assigned to this element.
The second line of the code uses the getElementById method to select an HTML element with an id of "speak". The speakEL variable is then assigned to this element.
The third line of the code uses the addEventListener method to add a click event listener to the speakEL element. The speakText function is passed as the event handler for this listener. This means that when the "speak" button is clicked, the speakText function will be executed.
The speakText function first calls the cancel method on the window.speechSynthesis object. This stops any speech that is currently being synthesized.
The function then retrieves the text that has been entered into the textEL element by accessing its value property. This text is then used to create a new SpeechSynthesisUtterance object, which is a type of speech request.
The speak method of the window.speechSynthesis object is then called with the utterance object as a parameter. This causes the Web Speech API to synthesize speech from the text in the utterance object and speak it through the device's speakers.
Create a JavaScript file with the name of script.js and paste the given codes into your JavaScript file and make sure it's linked properly to your HTML document, so that the scripts are executed on the page. Remember, you’ve to create a file with .js extension.
Final Output:
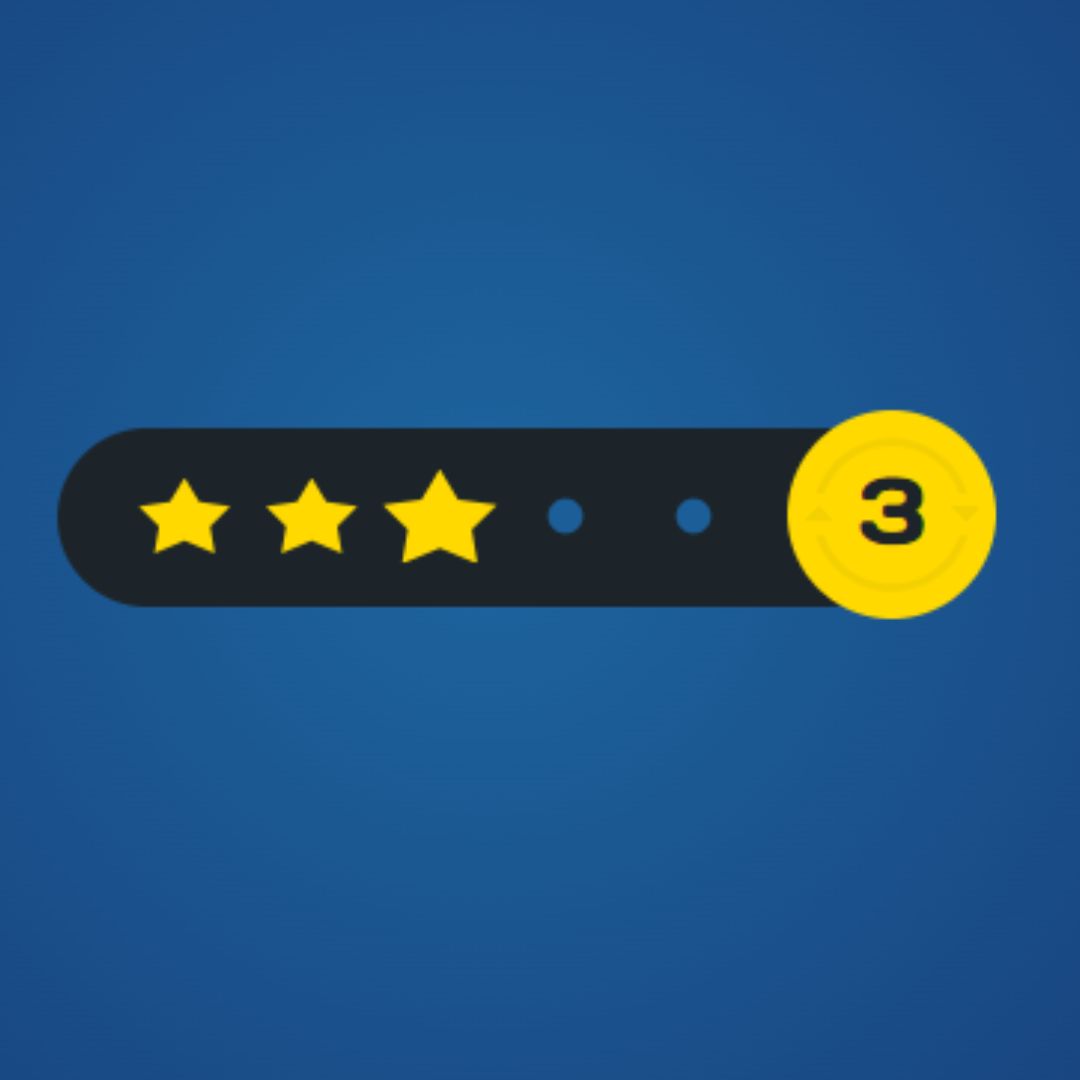
Conclusion:
In conclusion, adding text to speech functionality to a website can greatly improve the user experience for users with hearing impairments, learning disabilities, or anyone who prefers to listen to content instead of reading it. With the Speech Synthesis API, implementing this feature is simple and straightforward, making it accessible to developers of all skill levels.
By following the steps outlined in this guide, you can add text to speech functionality to your website and customize it to fit your users' needs. Remember to test your implementation thoroughly to ensure that it works correctly and provides a high-quality user experience.
Overall, the Speech Synthesis API is a powerful tool that can greatly enhance the accessibility and usability of your website. We hope that this guide has been helpful and that you can use these tips to create a more inclusive and accessible online experience for your users.
That’s a wrap!
I hope you enjoyed this post. Now, with these examples, you can create your own amazing page.
Did you like it? Let me know in the comments below 🔥 and you can support me by buying me a coffee.
And don’t forget to sign up to our email newsletter so you can get useful content like this sent right to your inbox!
Thanks! Faraz 😊
Subscribe to my Newsletter
Get the latest posts delivered right to your inbox, latest post.
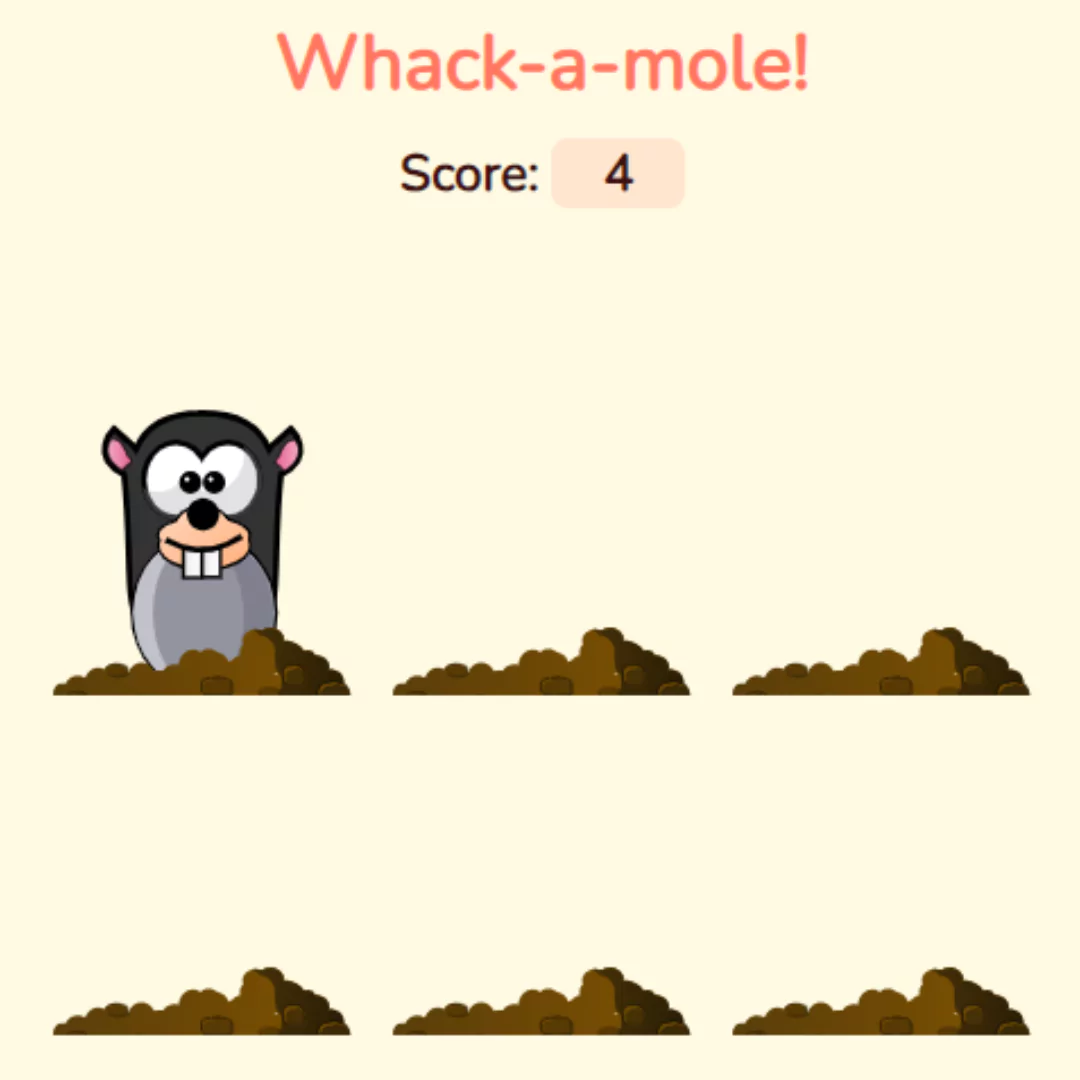
Create a Whack-a-Mole Game with HTML, CSS, and JavaScript | Step-by-Step Guide
Learn how to create a Whack-a-Mole game using HTML, CSS, and JavaScript. Follow this step-by-step guide to build, style, and add logic to your game. Get the complete source code and tips for enhancements.
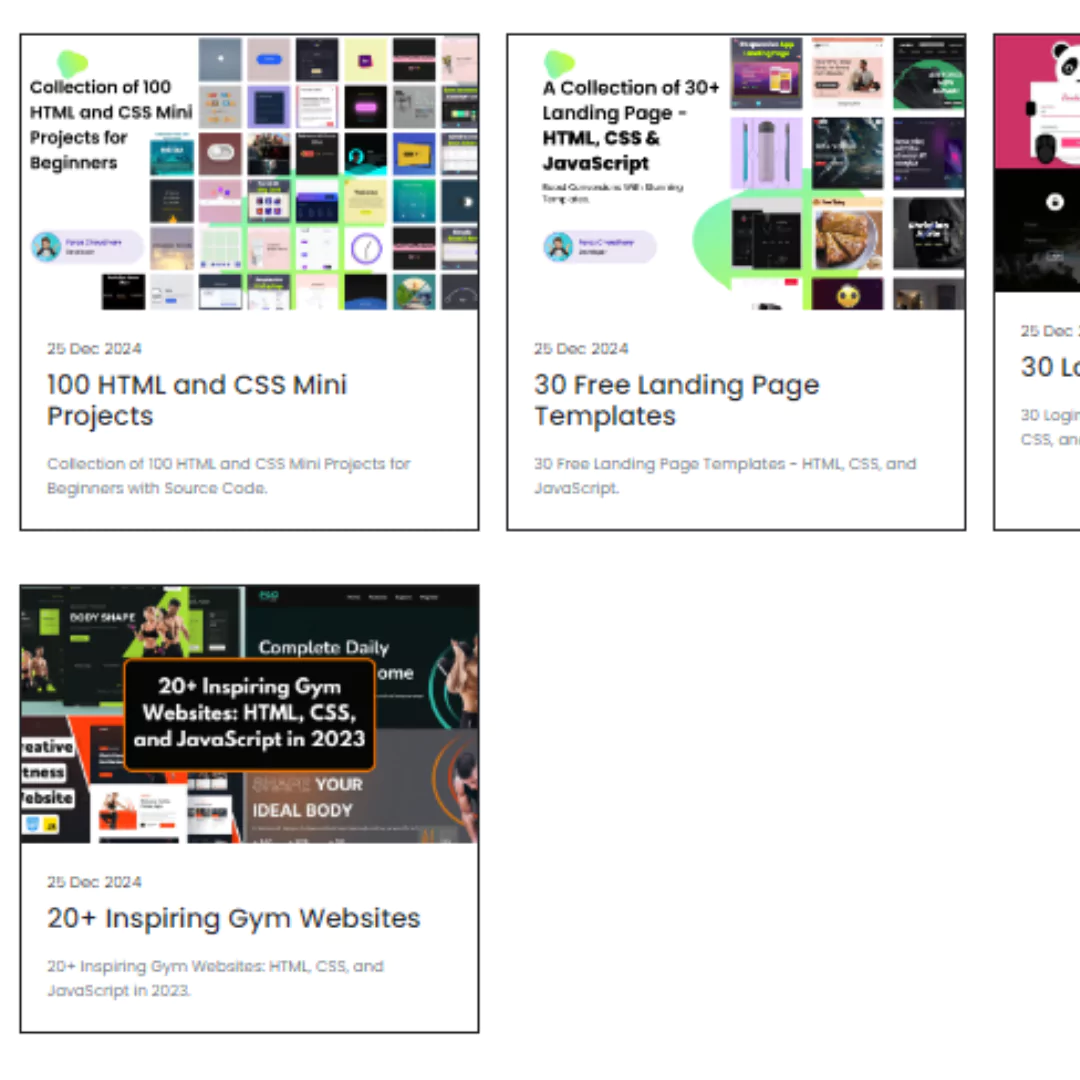
How to Create Grid Card Layout with HTML and Bootstrap | Step-by-Step Guide
June 11, 2024

Create Bootstrap Breadcrumb Navigation | Tutorial
June 10, 2024
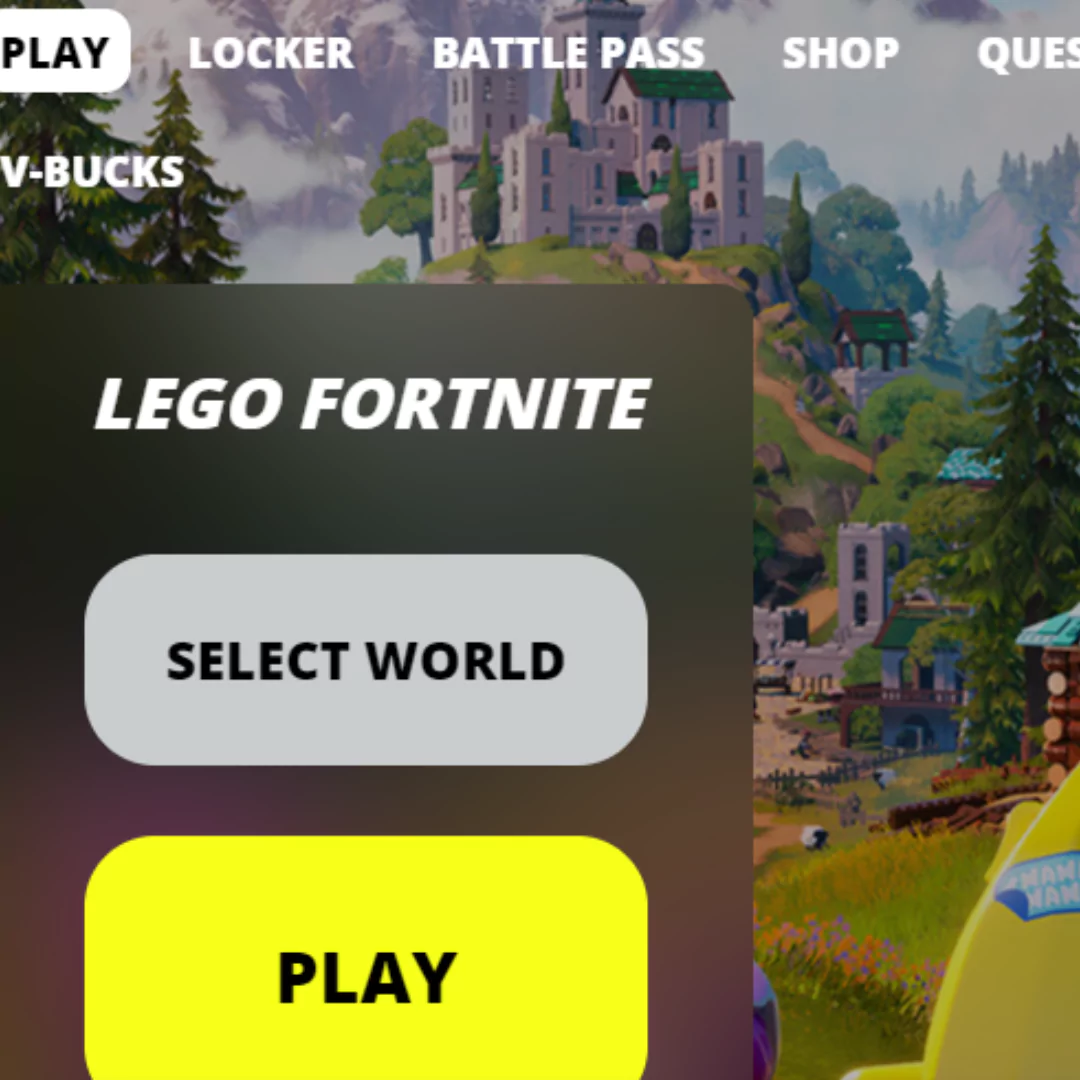
Create Fortnite Buttons Using HTML and CSS - Step-by-Step Guide
June 05, 2024
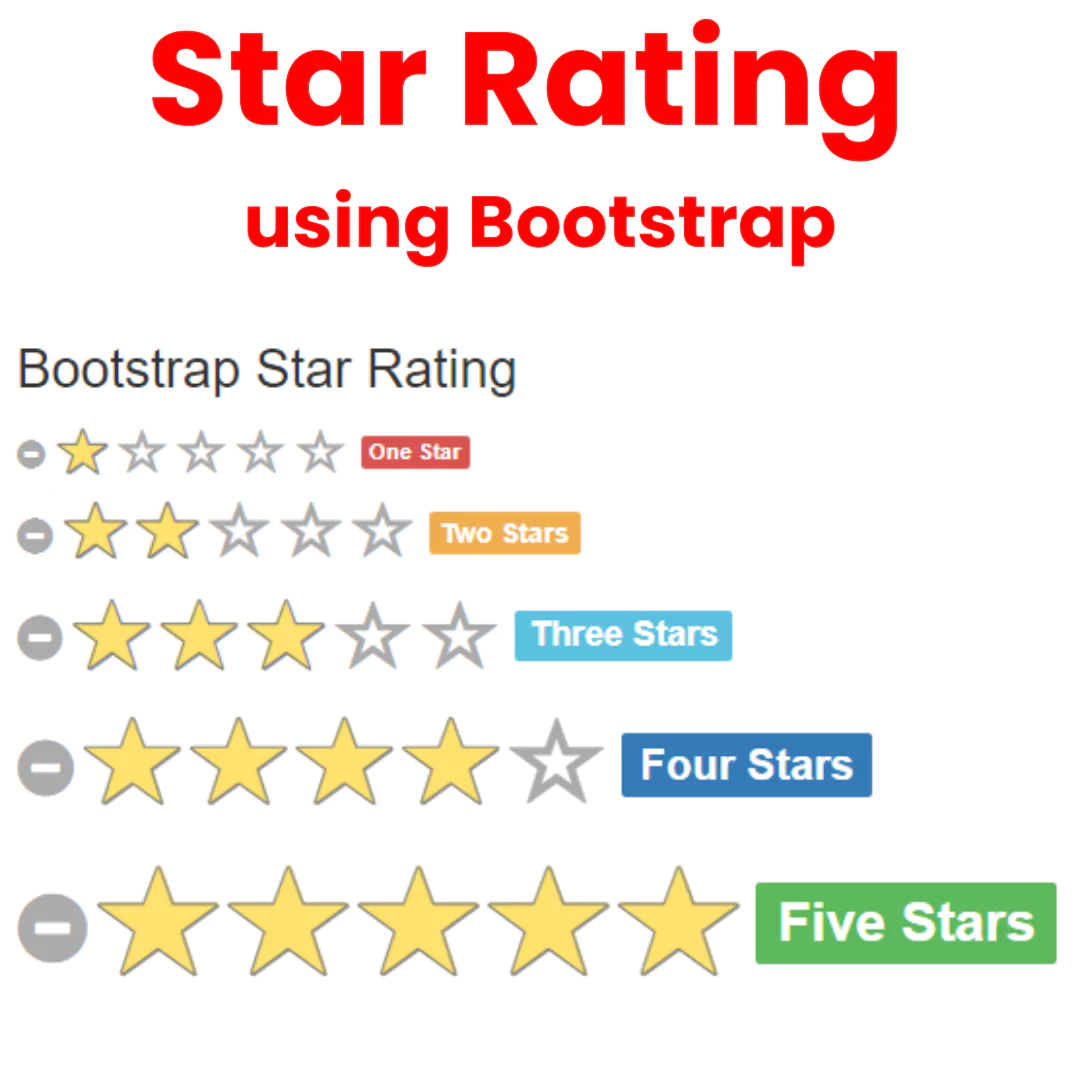
Create Star Rating using HTML and Bootstrap (Source Code) - Easy Guide
June 03, 2024
Learn how to create Fortnite-style buttons using HTML and CSS. This step-by-step guide includes source code and customization tips.
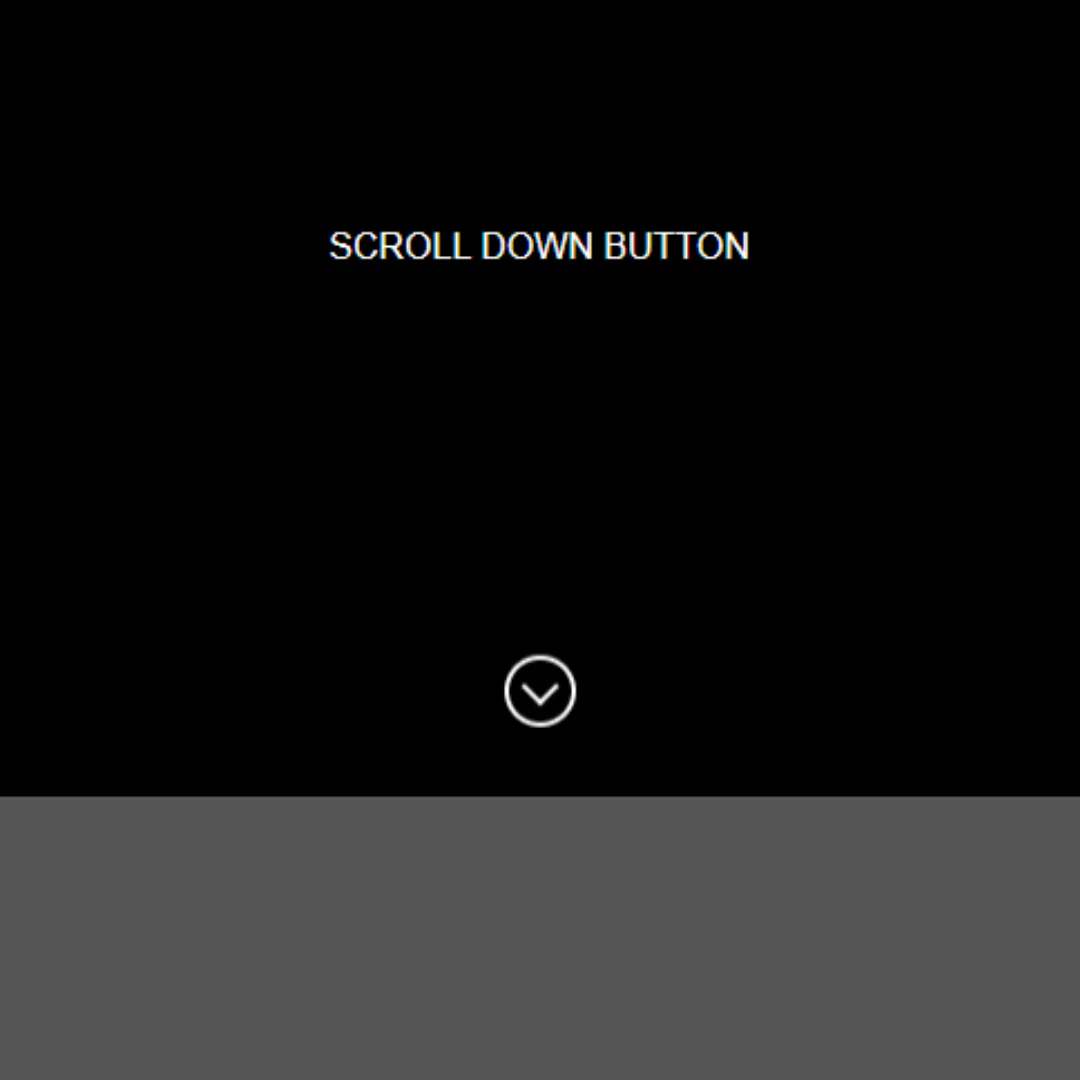
How to Create a Scroll Down Button: HTML, CSS, JavaScript Tutorial
March 17, 2024
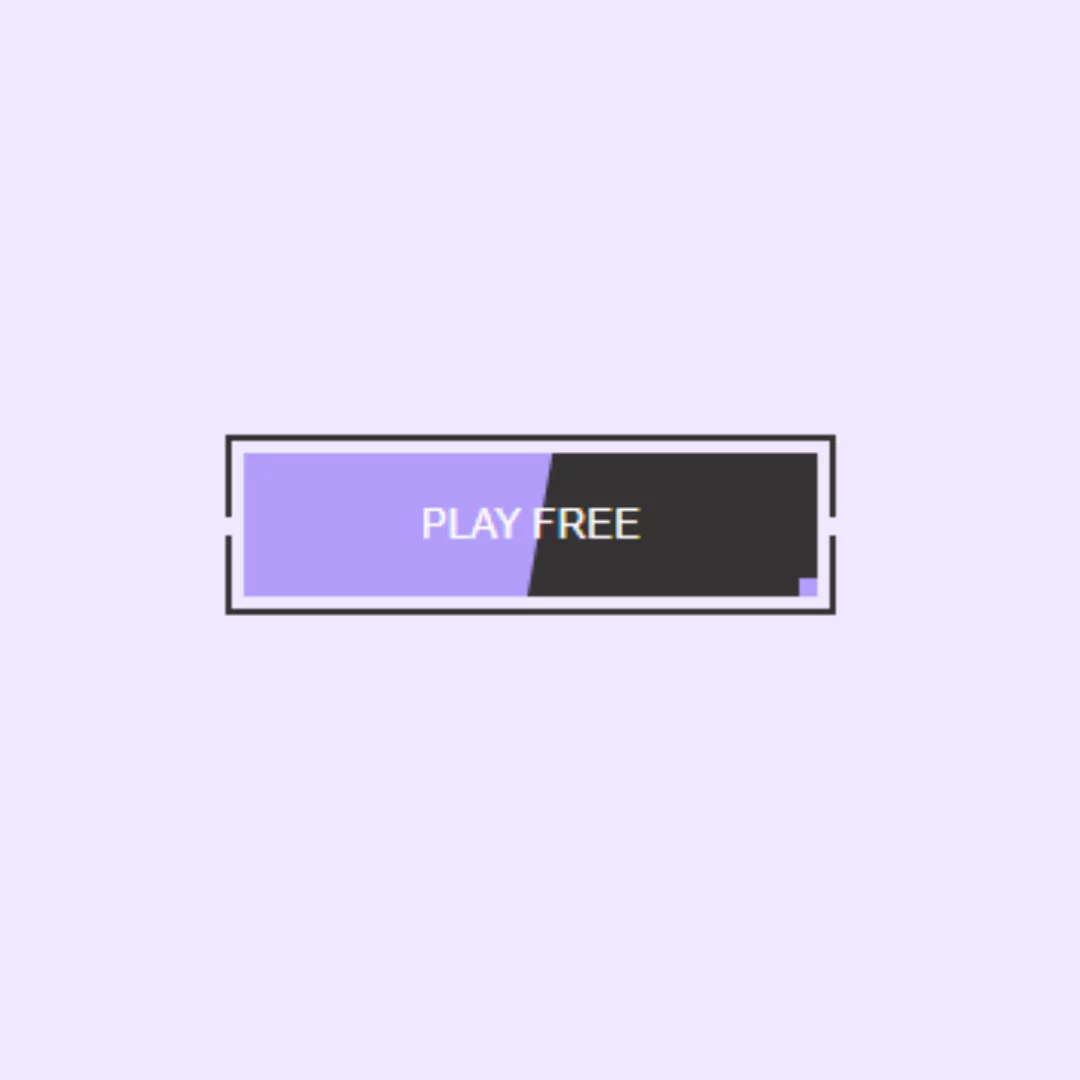
How to Create a Trending Animated Button Using HTML and CSS
March 15, 2024
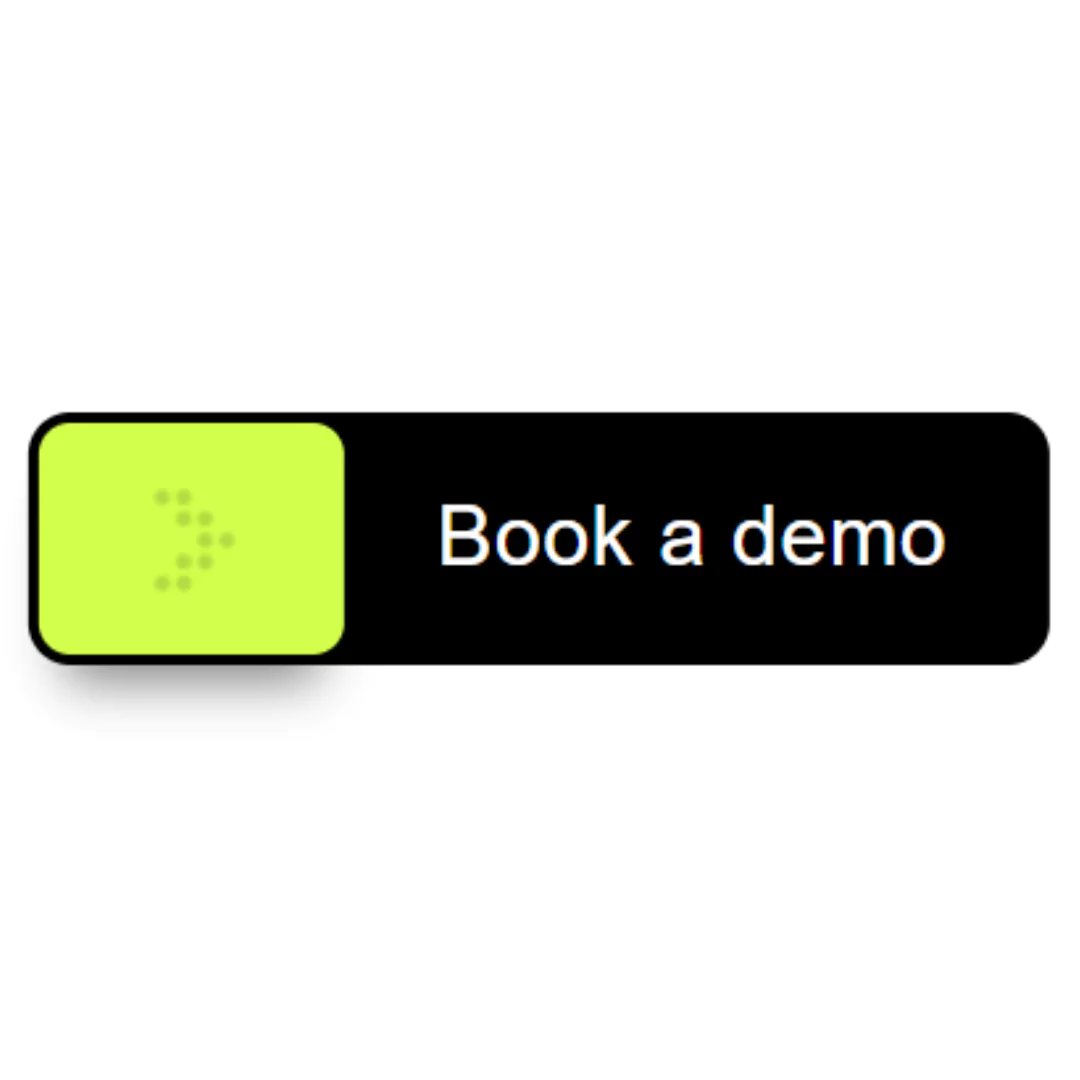
Create Interactive Booking Button with mask-image using HTML and CSS (Source Code)
March 10, 2024
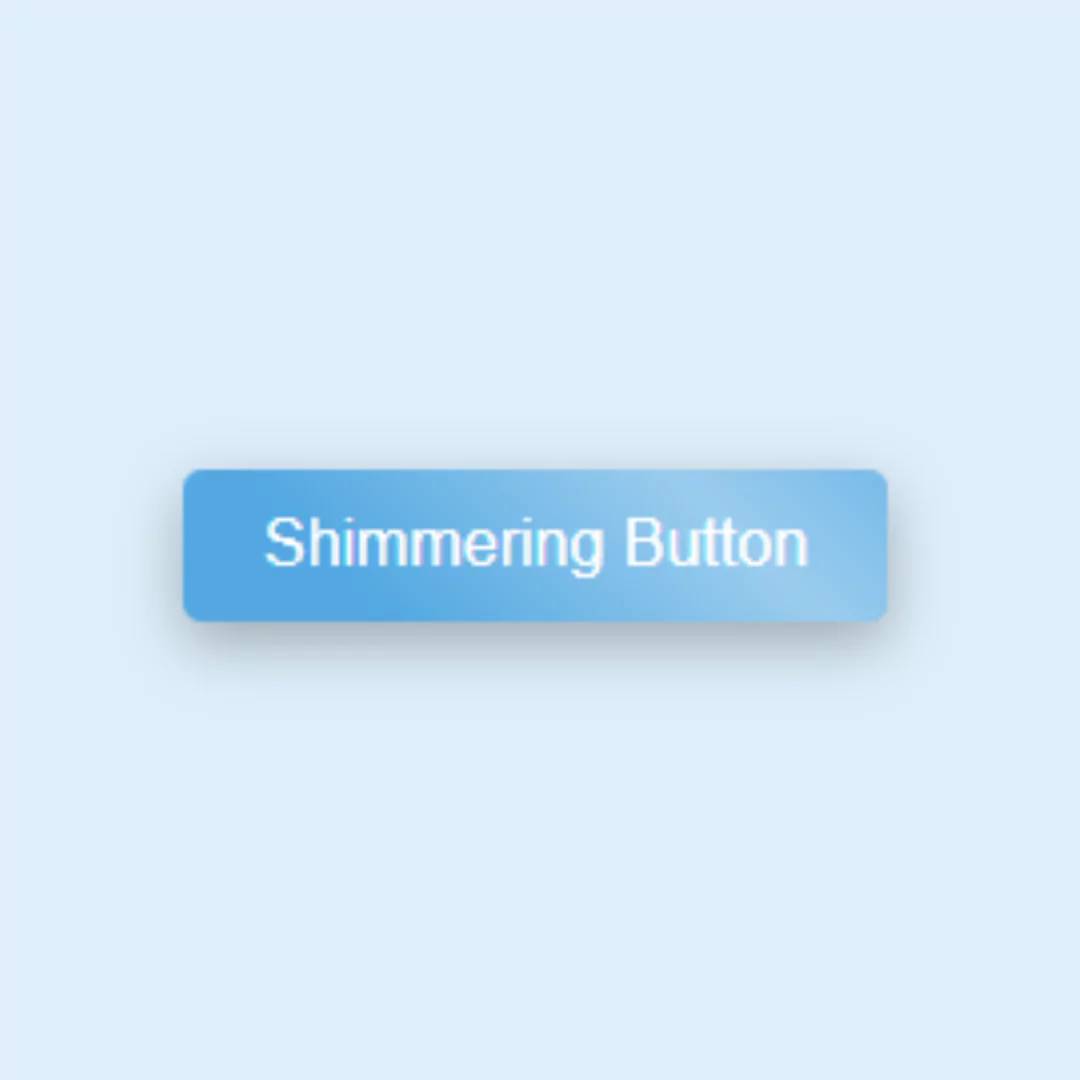
Create Shimmering Effect Button: HTML & CSS Tutorial (Source Code)
March 07, 2024
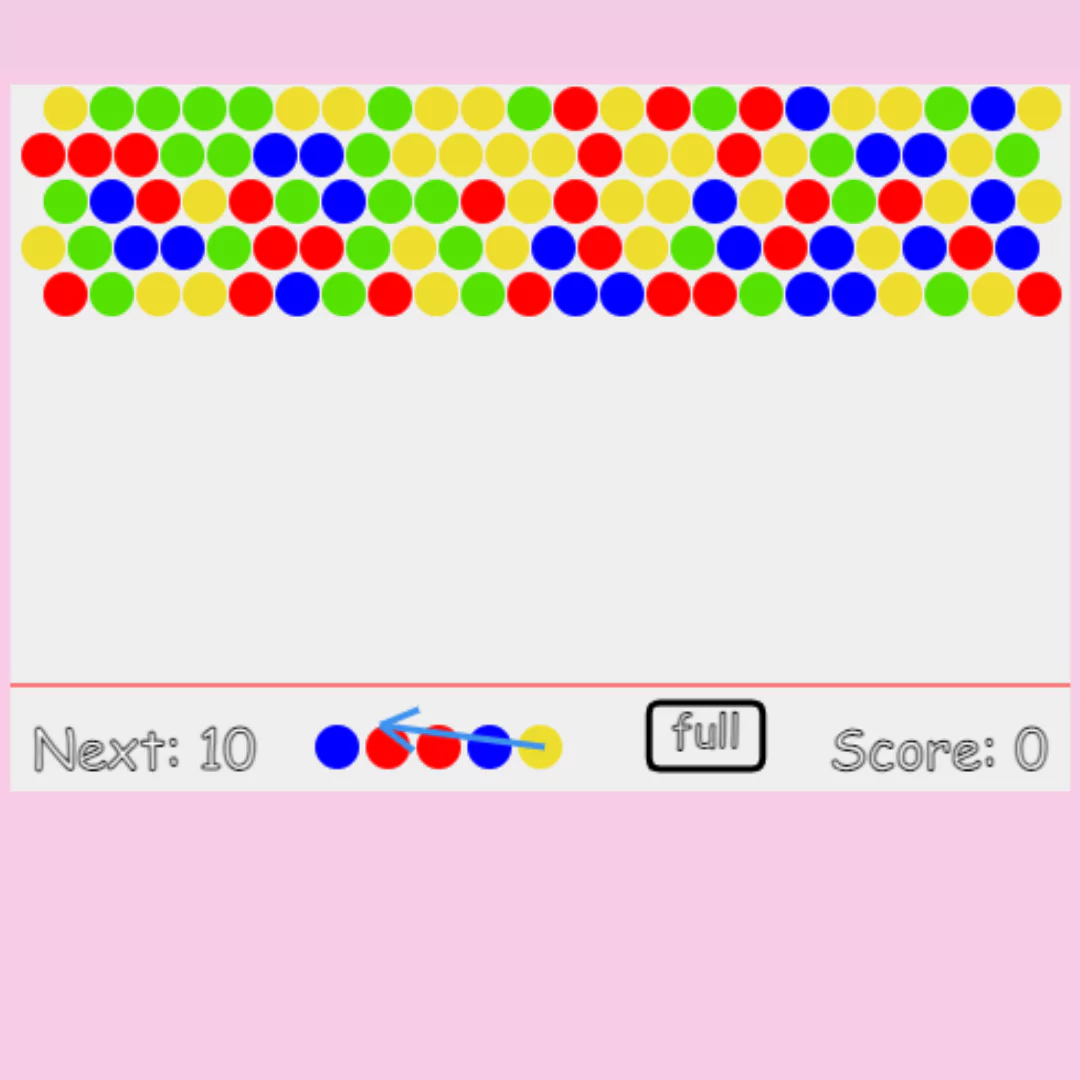
Create Your Own Bubble Shooter Game with HTML and JavaScript
May 01, 2024
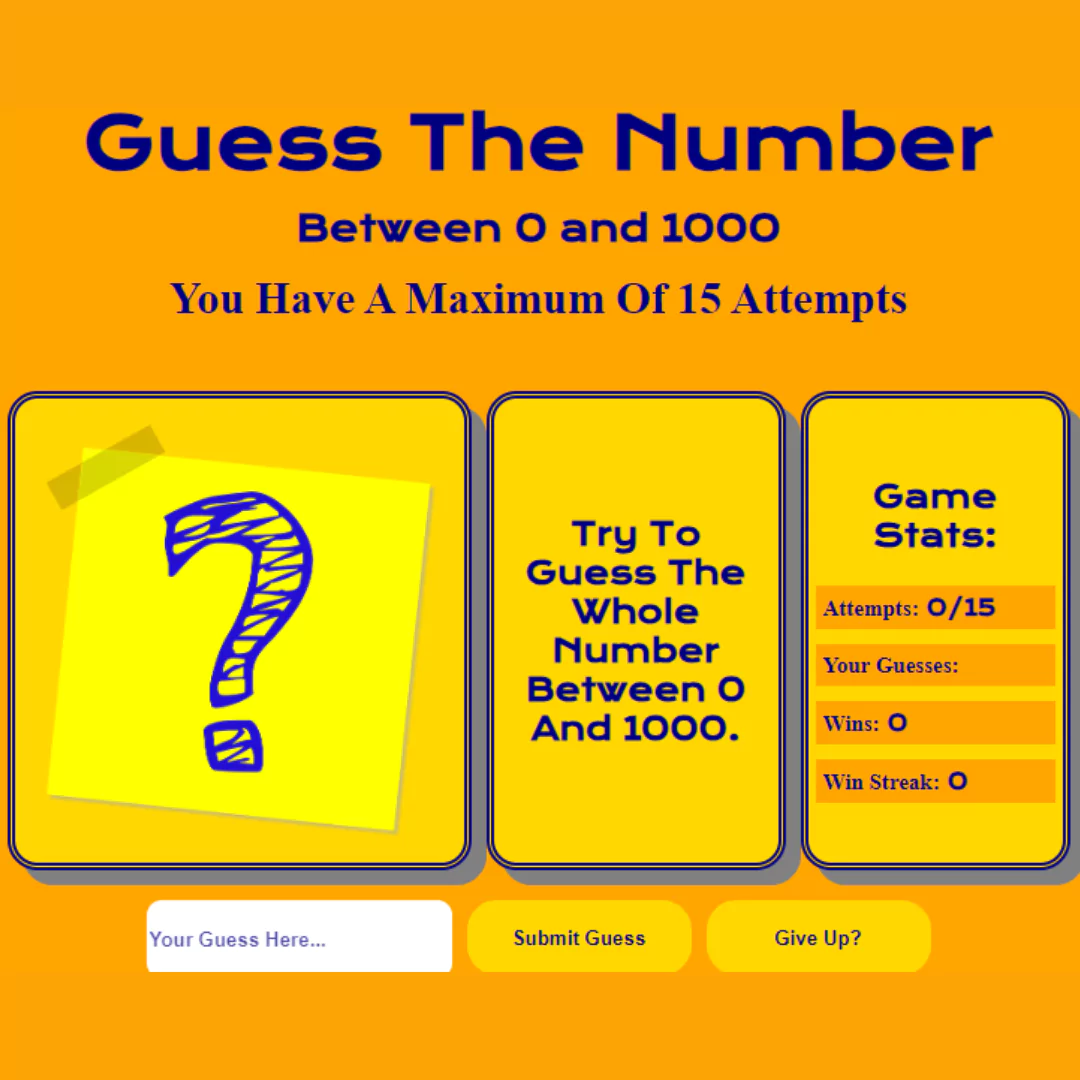
Build a Number Guessing Game using HTML, CSS, and JavaScript | Source Code
April 01, 2024
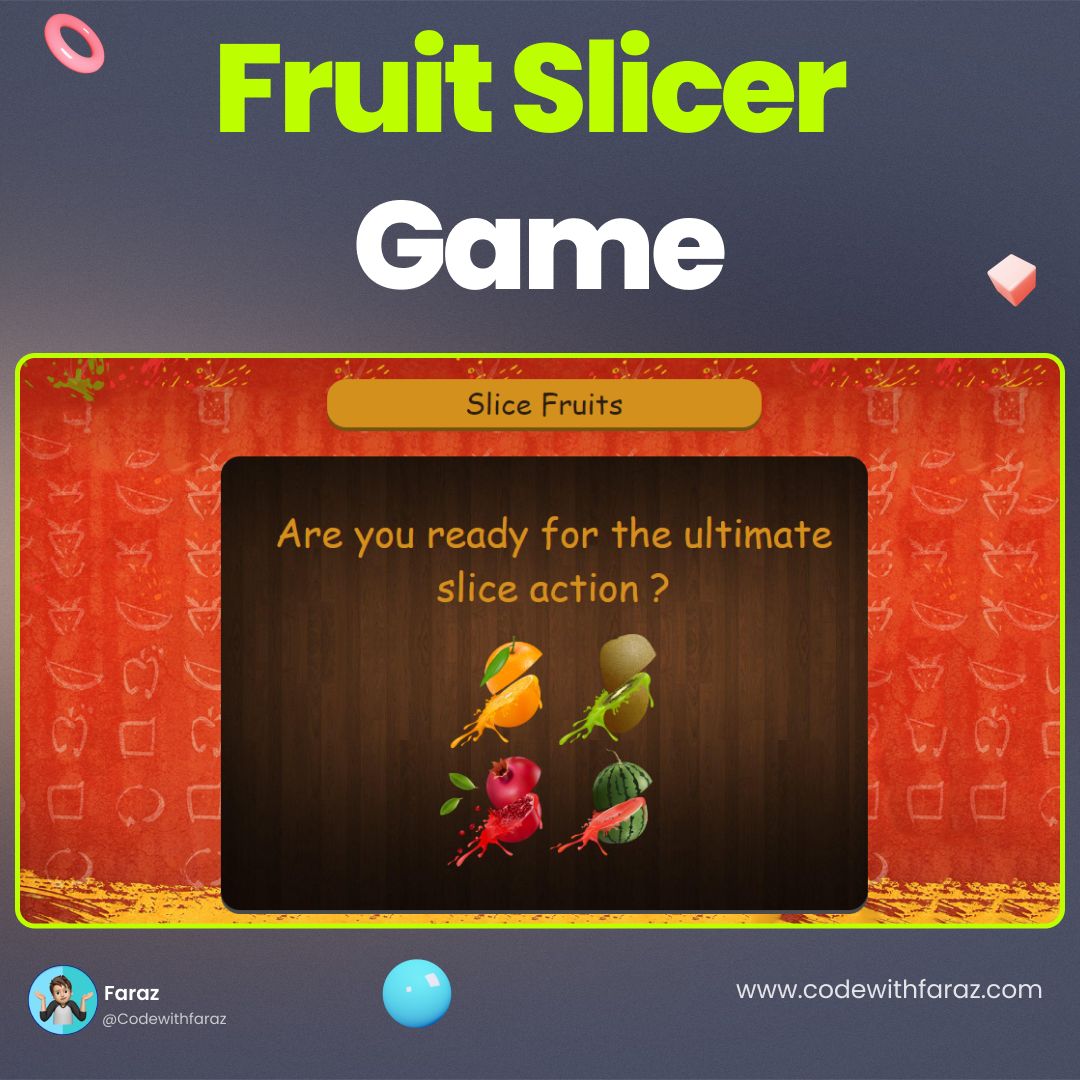
Building a Fruit Slicer Game with HTML, CSS, and JavaScript (Source Code)
December 25, 2023
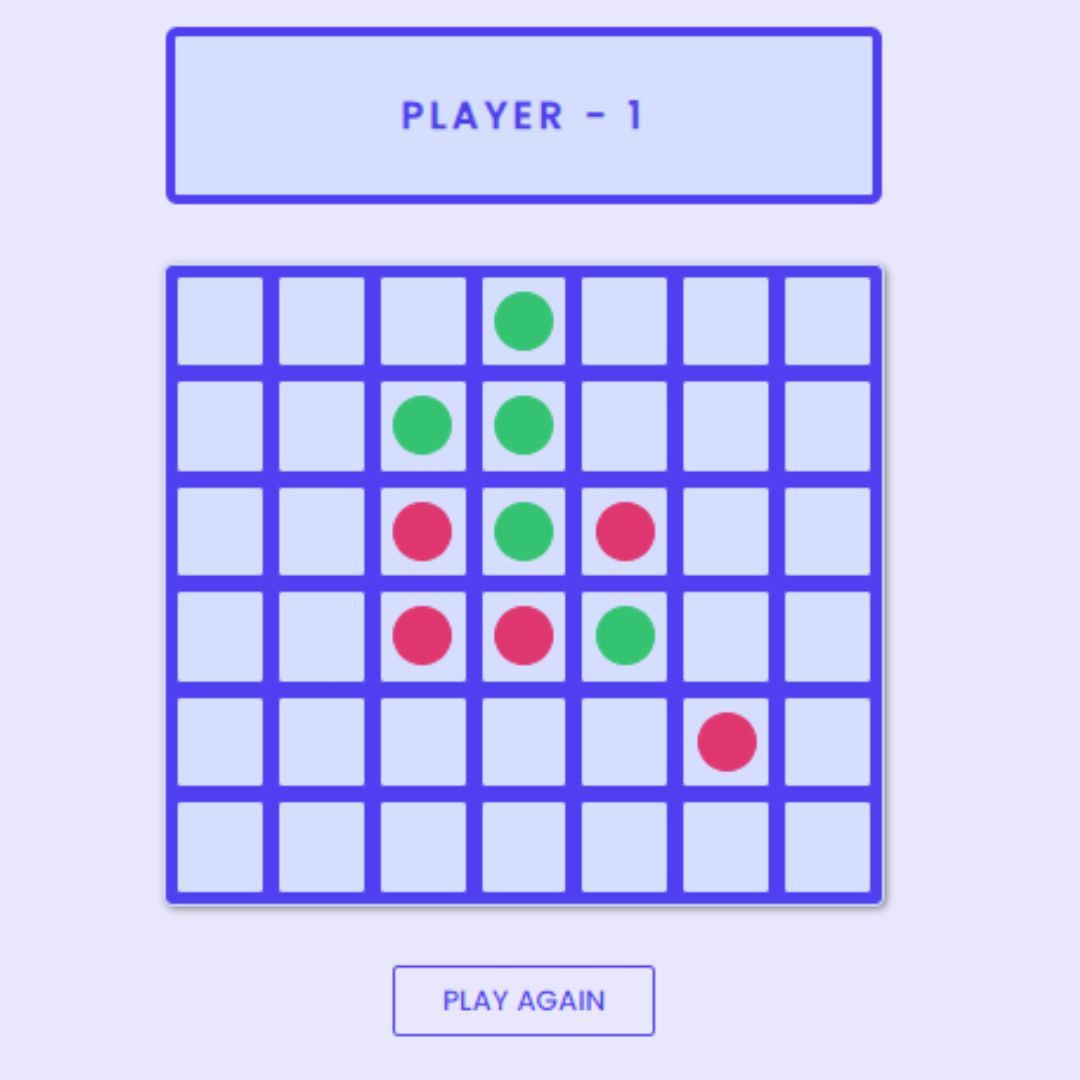
Create Connect Four Game Using HTML, CSS, and JavaScript (Source Code)
December 07, 2023
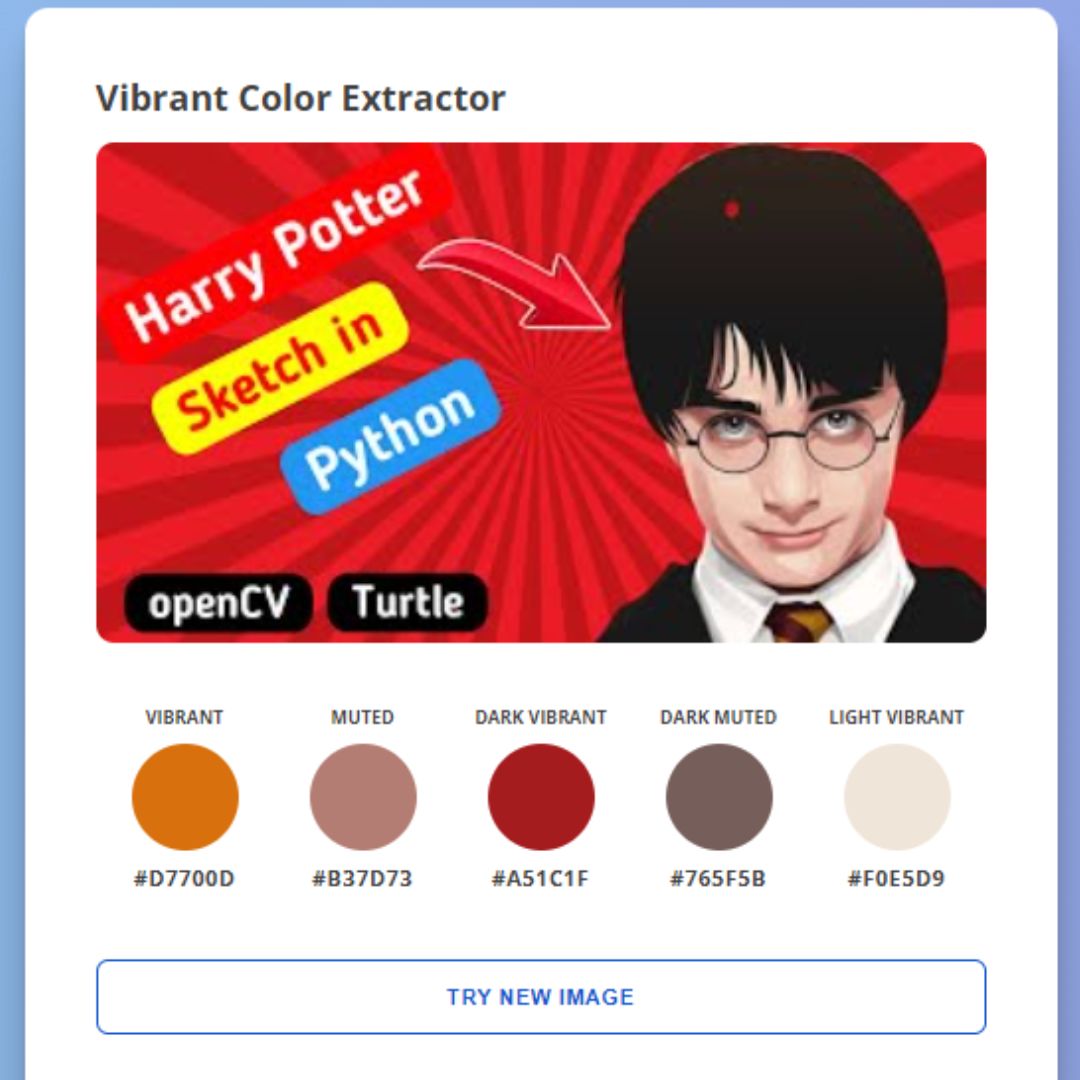
Create Image Color Extractor Tool using HTML, CSS, JavaScript, and Vibrant.js
Master the art of color picking with Vibrant.js. This tutorial guides you through building a custom color extractor tool using HTML, CSS, and JavaScript.
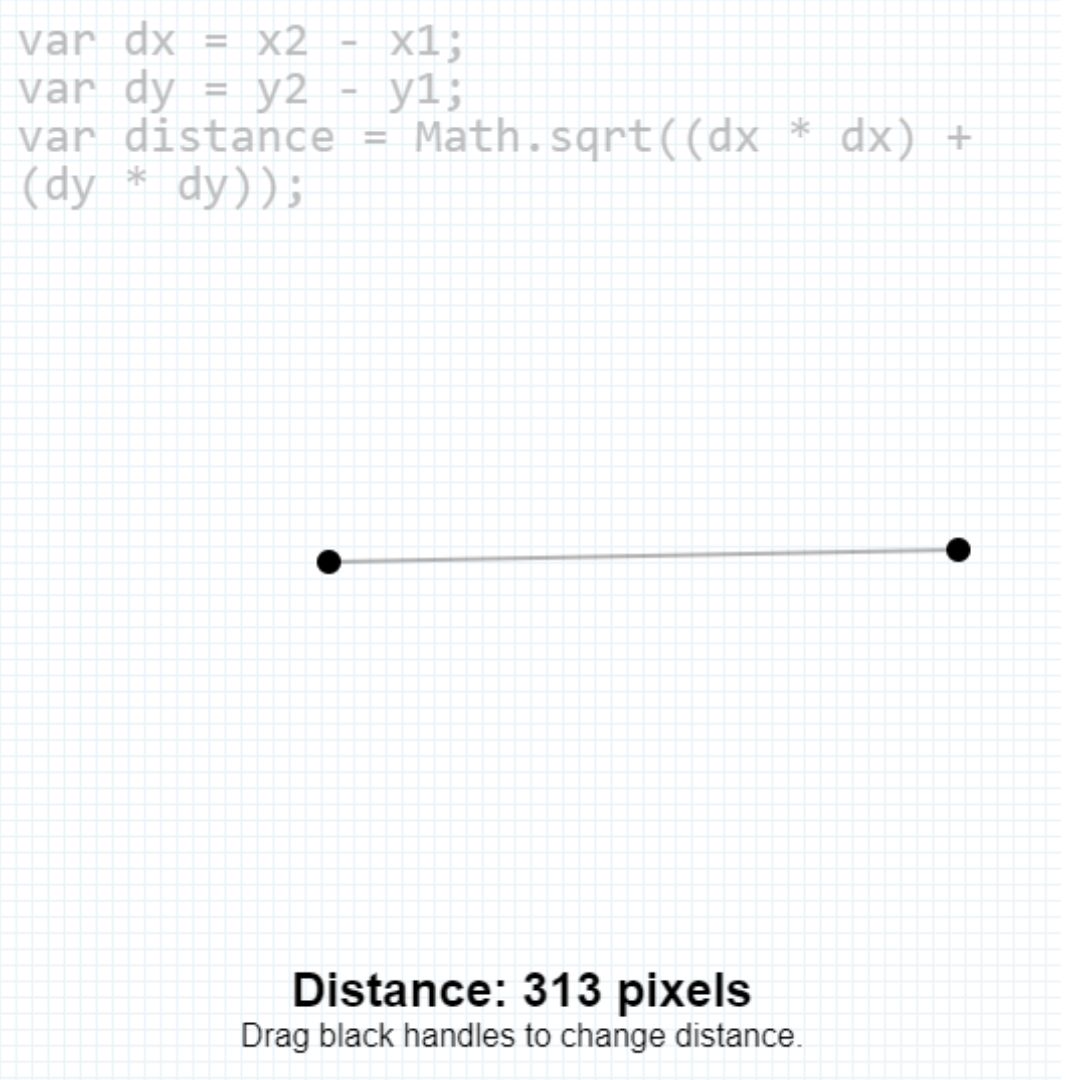
Build a Responsive Screen Distance Measure with HTML, CSS, and JavaScript
January 04, 2024
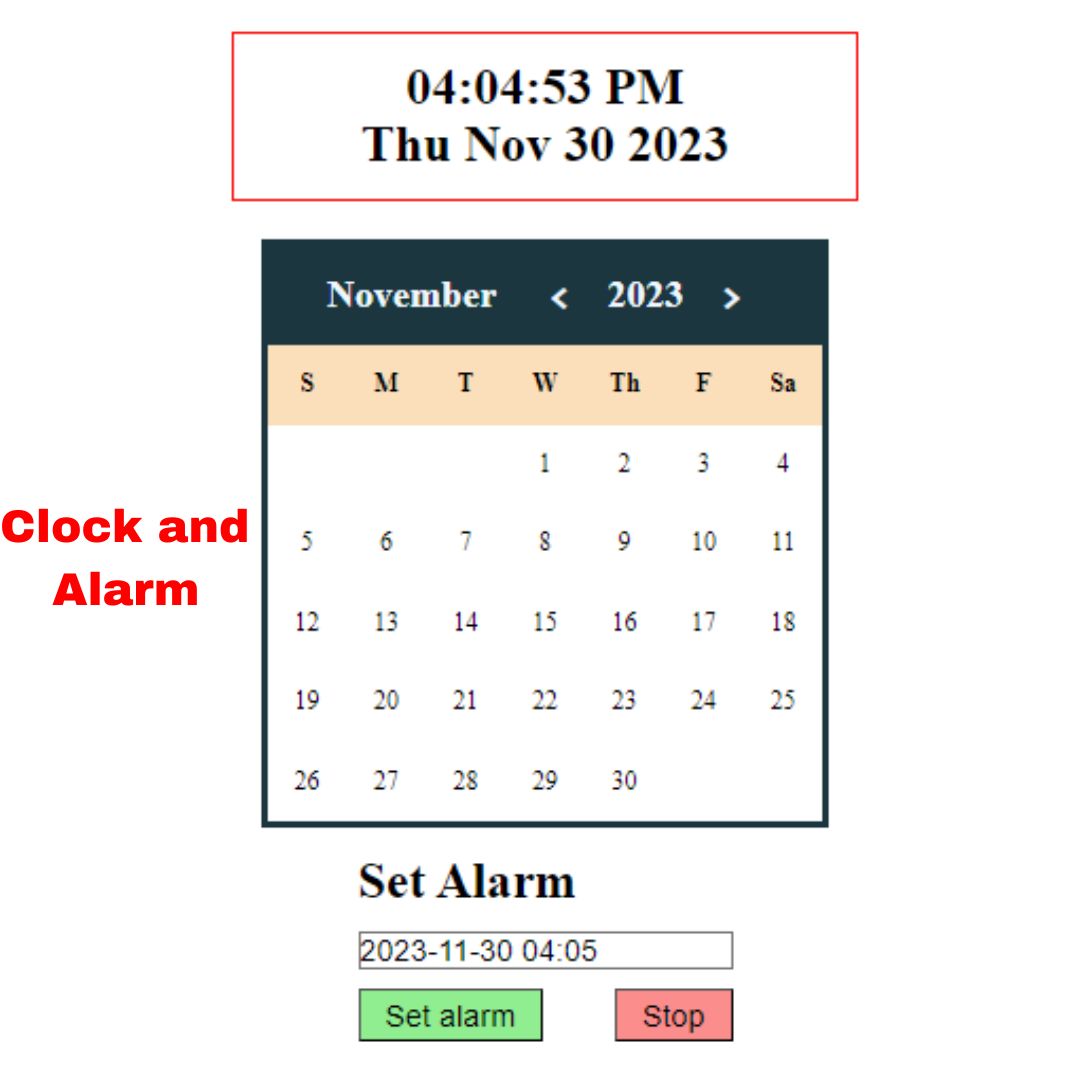
Crafting Custom Alarm and Clock Interfaces using HTML, CSS, and JavaScript
November 30, 2023
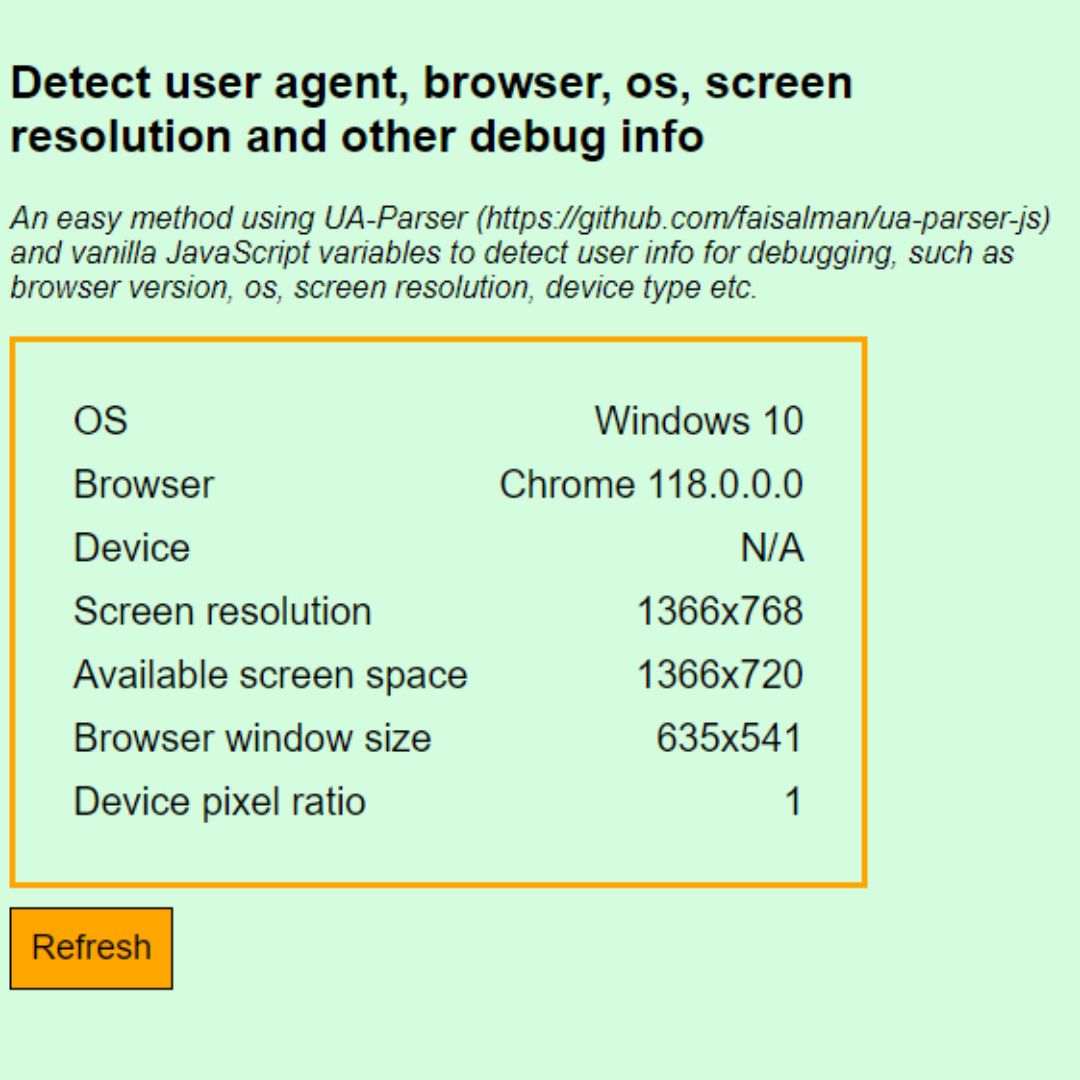
Detect User's Browser, Screen Resolution, OS, and More with JavaScript using UAParser.js Library
October 30, 2023
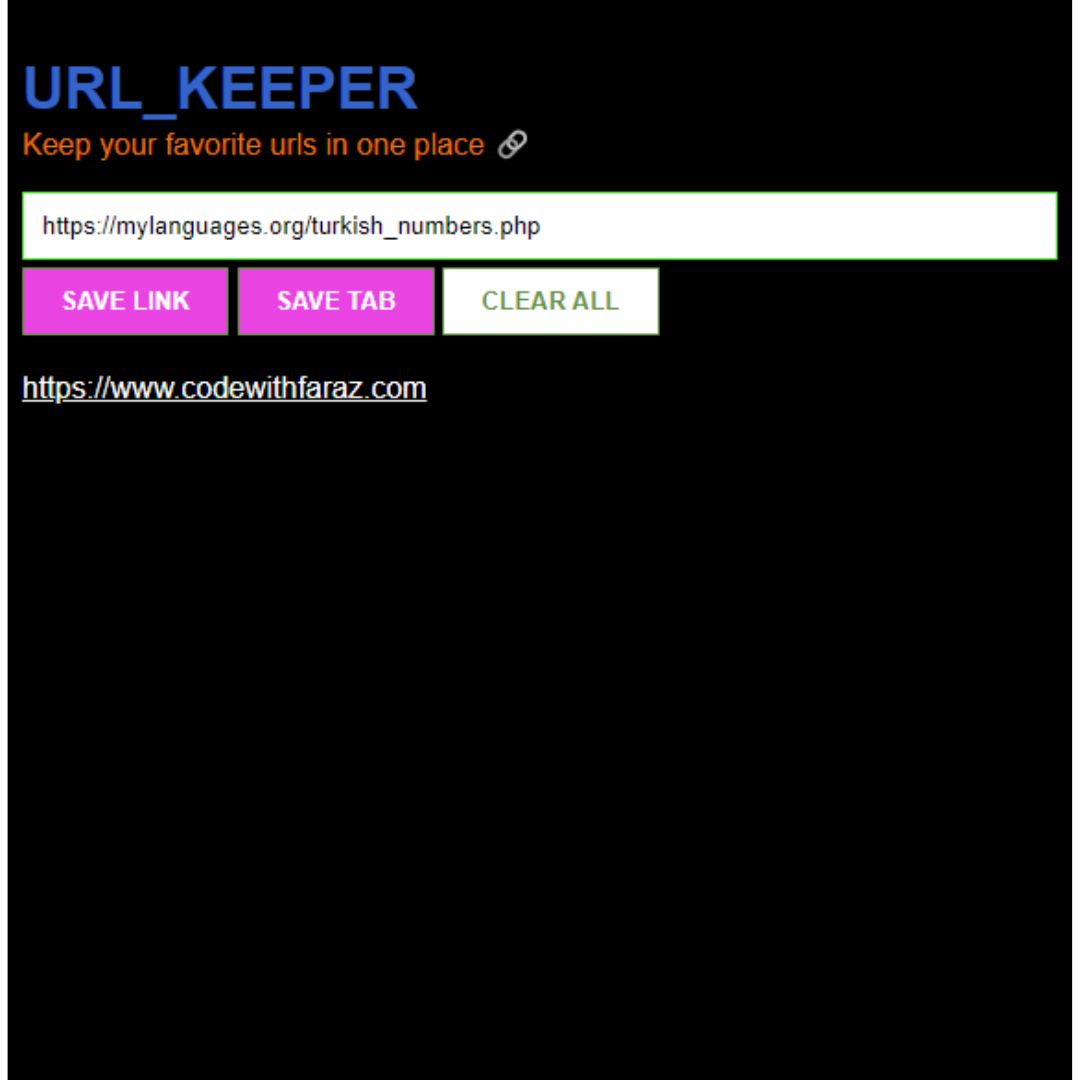
URL Keeper with HTML, CSS, and JavaScript (Source Code)
October 26, 2023
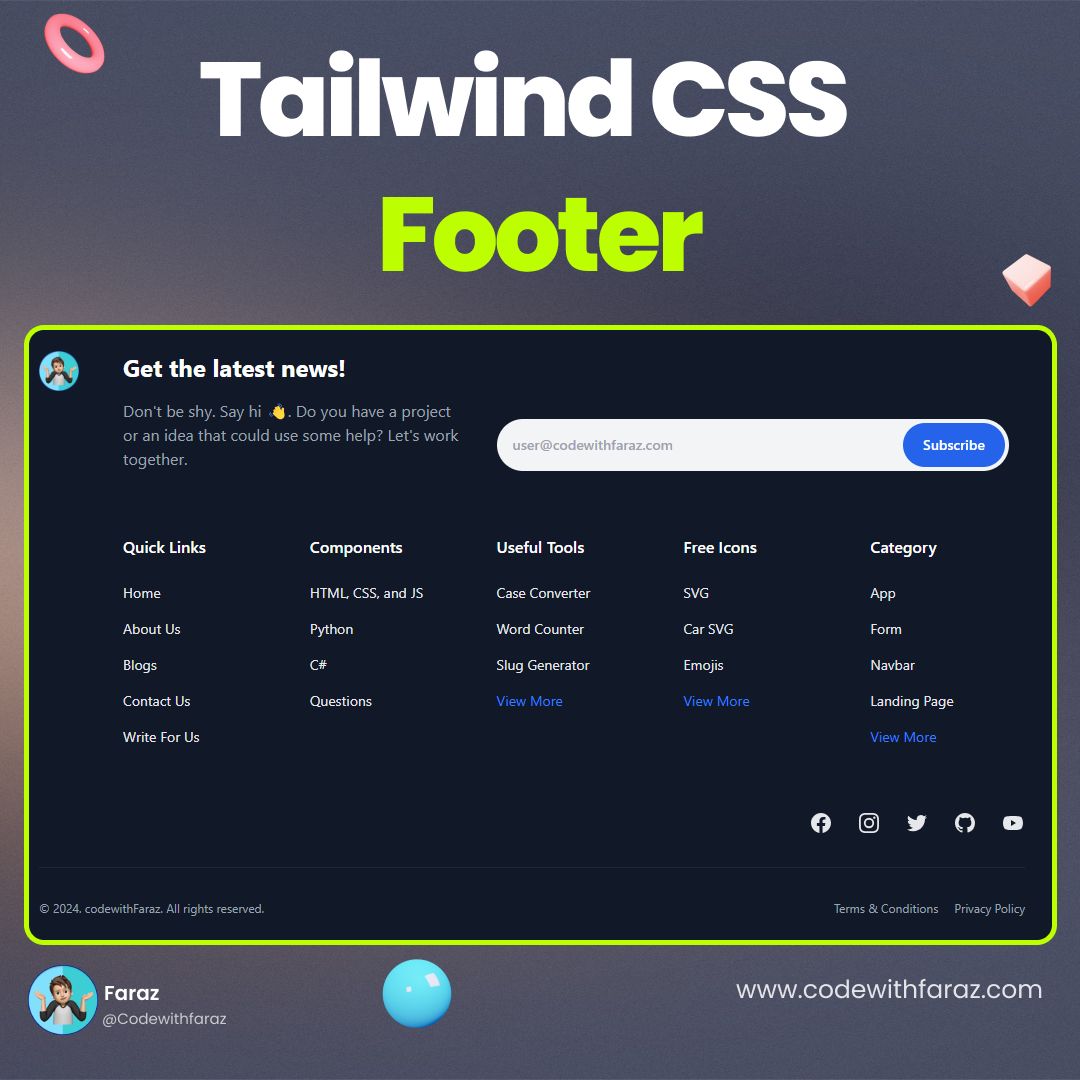
Creating a Responsive Footer with Tailwind CSS (Source Code)
Learn how to design a modern footer for your website using Tailwind CSS with our detailed tutorial. Perfect for beginners in web development.
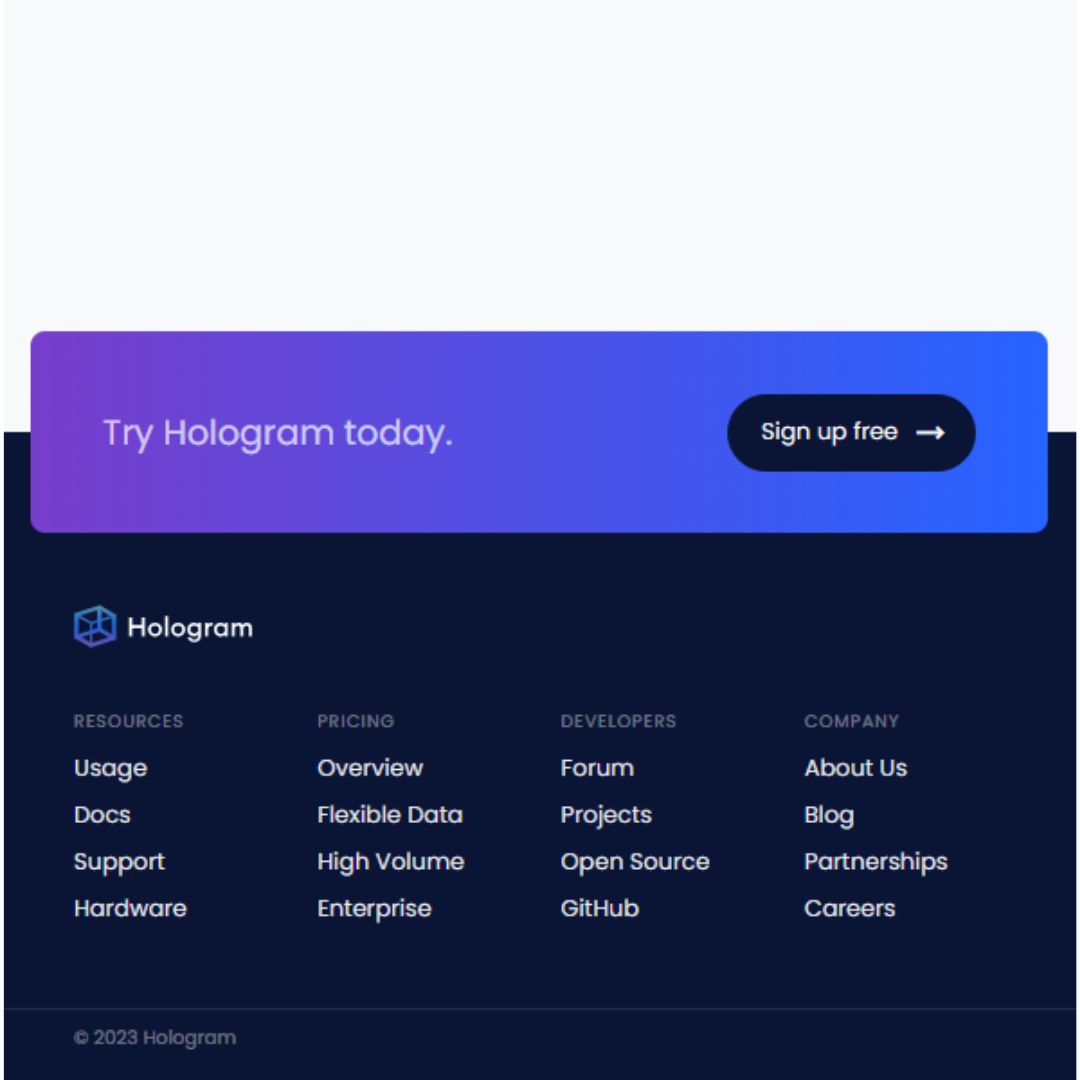
Crafting a Responsive HTML and CSS Footer (Source Code)
November 11, 2023
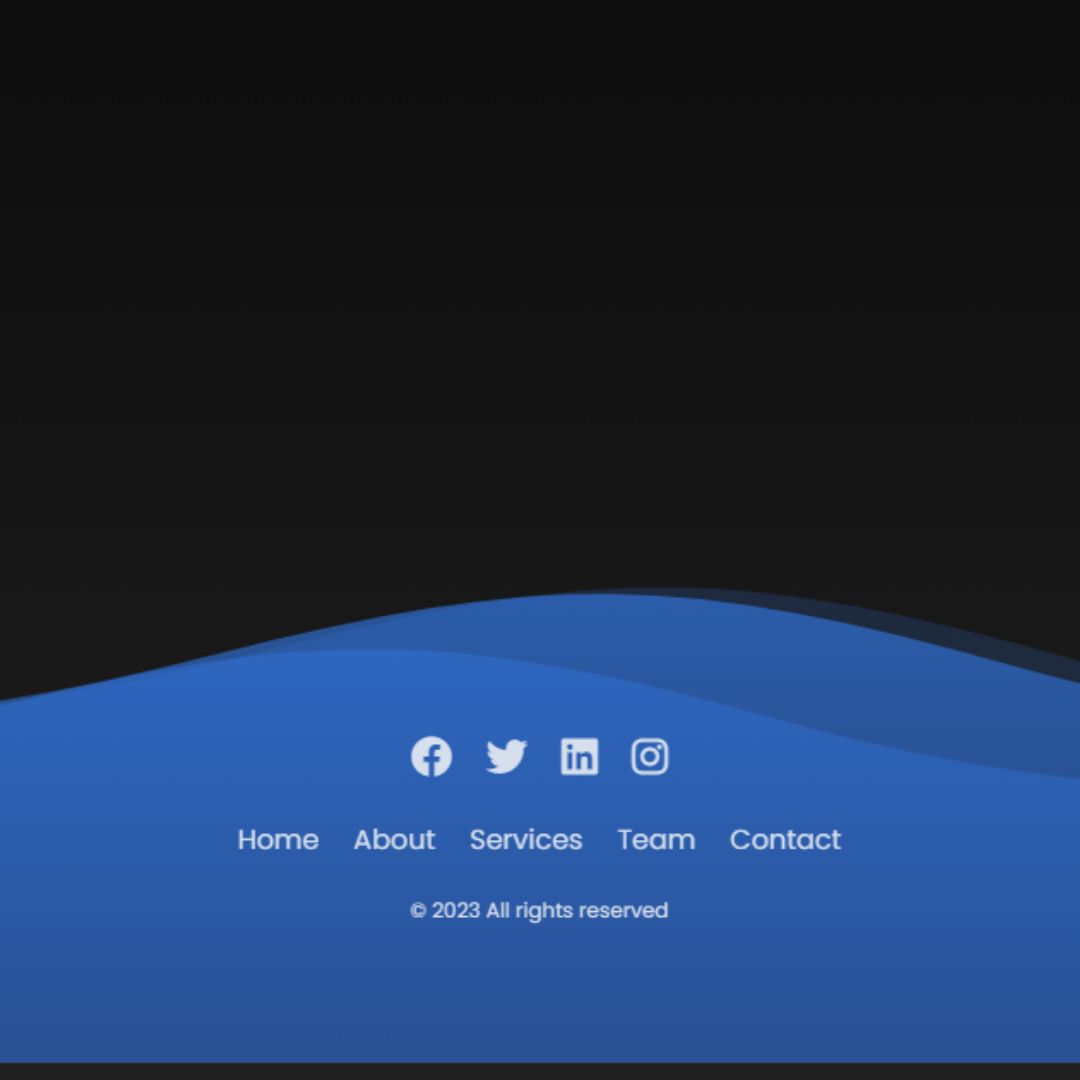
Create an Animated Footer with HTML and CSS (Source Code)
October 17, 2023
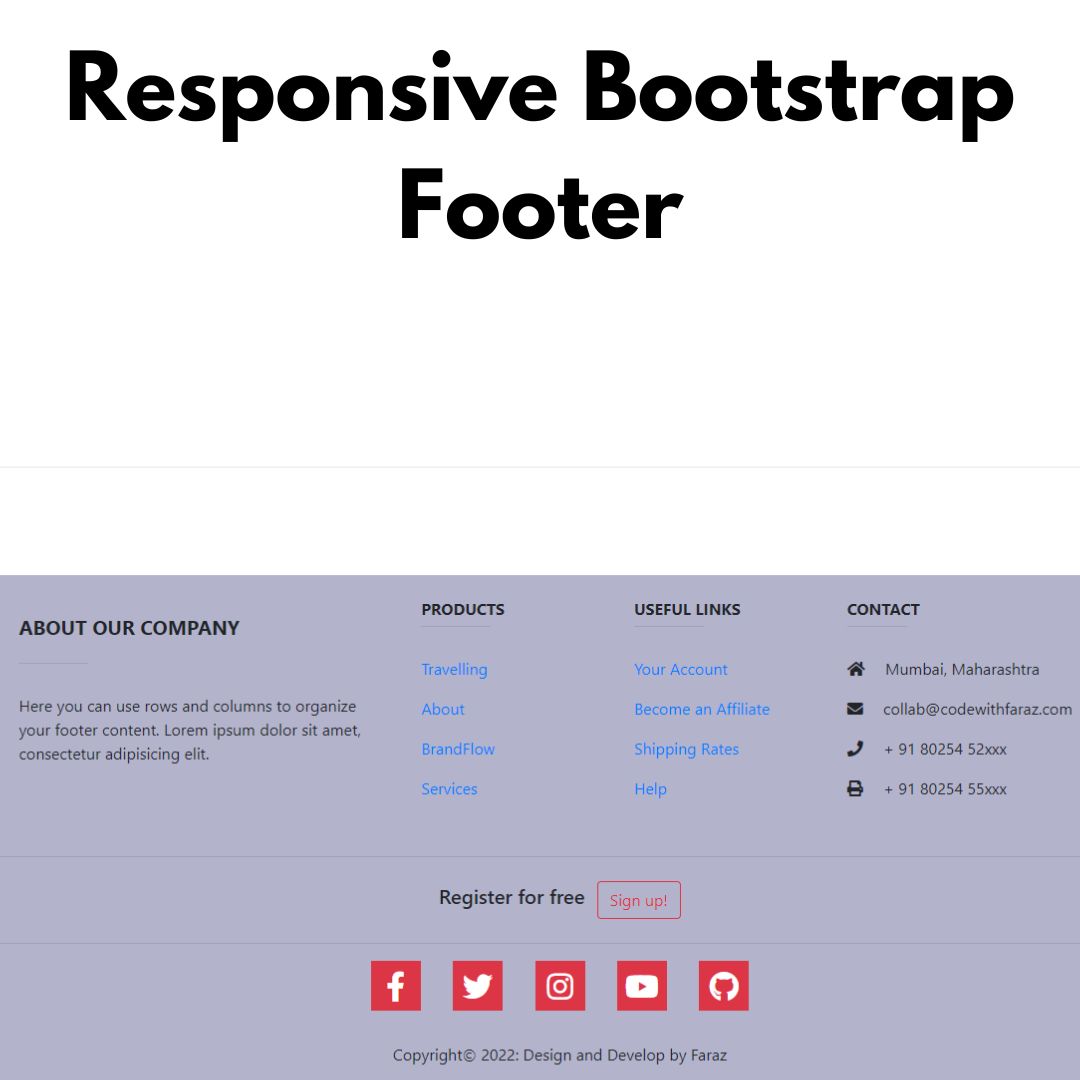
Bootstrap Footer Template for Every Website Style
March 08, 2023
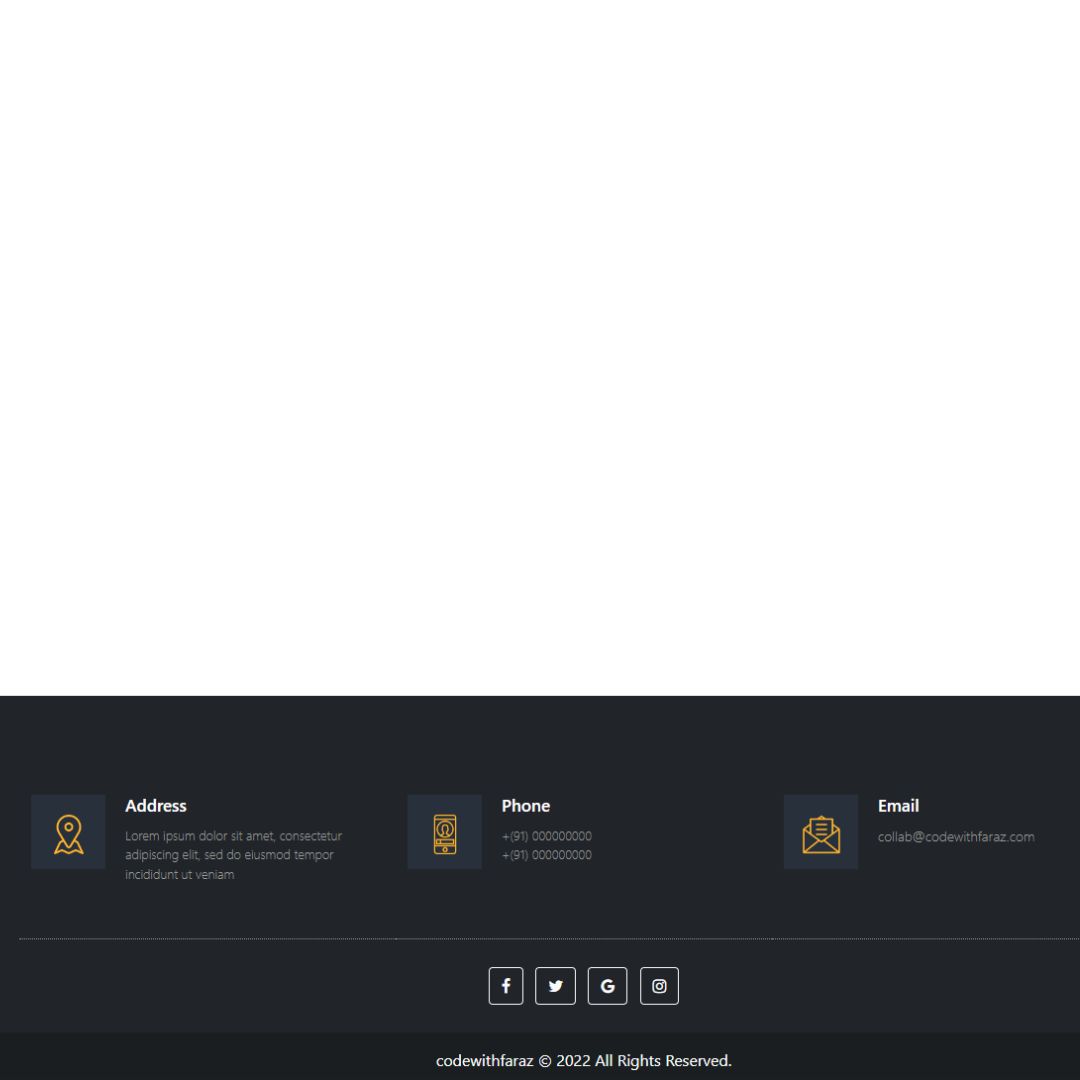
How to Create a Responsive Footer for Your Website with Bootstrap 5
August 19, 2022
Using text to speech APIs with JavaScript
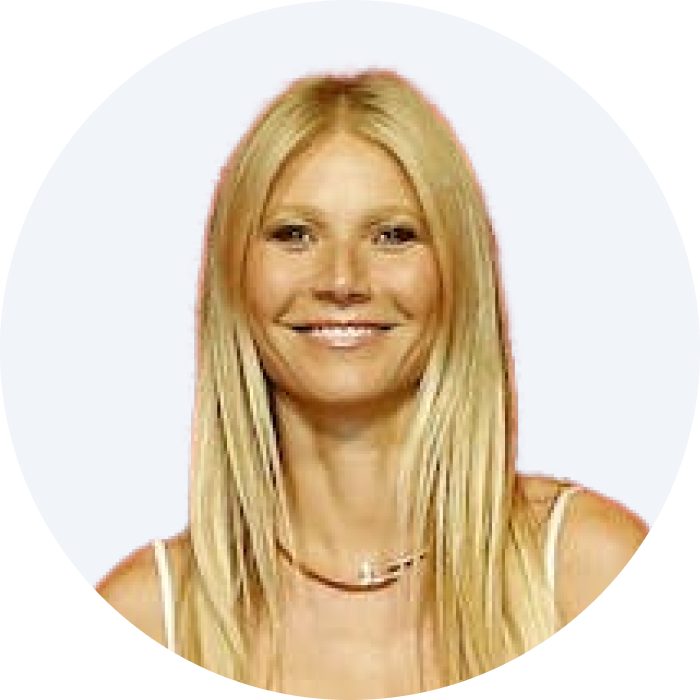
Looking for our Text to Speech Reader ?
Featured In
Table of contents, getting started with javascript and text to speech, seamlessly integrate with speechify.
Using a TTS API with JavaScript enhances web application user experiences. The Web Speech API, supported by Chrome and Firefox, offers speech synthesis and recognition capabilities. This tutorial covers integrating a TTS API, converting text to speech, customizing settings, and utilizing available voices.
Using a text-to-speech (TTS) API with JavaScript can greatly enhance the user experience of web applications. The Web Speech API, supported by modern browsers like Chrome and Firefox, provides a powerful set of tools for speech synthesis and speech recognition. In this tutorial, we will explore how to integrate a TTS API into your JavaScript code, convert text to speech, customize speech settings, and utilize available voices.
To get started, you'll need a basic understanding of HTML, CSS, and JavaScript. Begin by creating an HTML file and linking your JavaScript file using the script src tag. In your JavaScript file, initialize the speech synthesis object and set up an event listener for when the voices are ready. const synth = window.speechSynthesis; // Wait for voices to be loaded synth.onvoiceschanged = () => { const voices = synth.getVoices(); // Do something with the available voices }; Once the voices are loaded, you can access them using the synth.getVoices() method. This will return a list of available voices that you can use for speech synthesis. You can loop through the voices using forEach and display them in your HTML. const voiceSelect = document.getElementById('voice-select'); voices.forEach((voice) => { const option = document.createElement('option'); option.textContent = ${voice.name} (${voice.lang}); option.setAttribute('value', voice.lang); voiceSelect.appendChild(option); }); Next, you can create a function to synthesize speech from the selected voice. This function takes the text input from a textarea element and uses the selected voice to generate speech. const speak = () => { const text = document.getElementById('text-input').value; const voice = voices[voiceSelect.selectedIndex]; const utterance = new SpeechSynthesisUtterance(text); utterance.voice = voice; synth.speak(utterance); }; Add an event listener to the button or form submit to trigger the speak function. const button = document.getElementById('speak-button'); button.addEventListener('click', speak); With these few lines of code, you can convert text to speech in real-time. Customize the speech rate, pitch, and volume by setting properties on the SpeechSynthesisUtterance object. utterance.rate = 0.8; utterance.pitch = 1; utterance.volume = 1; As you continue to explore the Web Speech API, you'll find additional features for speech recognition and controlling speech synthesis events. Remember to consult the official documentation for more details and attributions.
When it comes to using a text-to-speech API with JavaScript, Speechify stands out as the best choice. With its seamless integration with the Web Speech API, Speechify makes it incredibly easy to convert text to speech in real-time. Its comprehensive documentation and user-friendly tutorials provide step-by-step guidance, making it ideal for both novice and experienced developers in web development. With Speechify, you have access to a wide range of available voices and can customize speech settings such as speech rate and pitch. Whether you're a front-end developer or a software developer, Speechify is the perfect tool to enhance your web applications and create engaging user experiences. In conclusion, using a text-to-speech API with JavaScript opens up a world of possibilities for web development. By integrating speech synthesis into your projects, you can create engaging and accessible user experiences. Whether you're a front-end developer or a software developer, learning how to leverage the Web Speech API will enhance your skillset and enable you to build dynamic applications. So why not give it a try and bring your webpages to life with the power of text-to-speech.
Kurzweil vs. Read&Write: A Breakdown
ChatGPT 5 Release Date and What to Expect
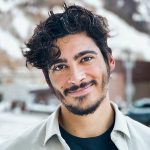
Cliff Weitzman
Cliff Weitzman is a dyslexia advocate and the CEO and founder of Speechify, the #1 text-to-speech app in the world, totaling over 100,000 5-star reviews and ranking first place in the App Store for the News & Magazines category. In 2017, Weitzman was named to the Forbes 30 under 30 list for his work making the internet more accessible to people with learning disabilities. Cliff Weitzman has been featured in EdSurge, Inc., PC Mag, Entrepreneur, Mashable, among other leading outlets.

Free Web Design Code & Scripts
Home / Text & Input / Text To Speech using JavaScript
Text To Speech using JavaScript
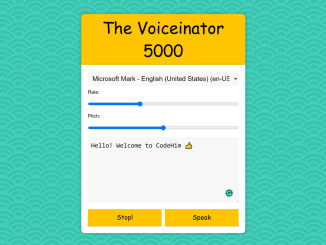
Code Snippet: | Speech Synthesis |
Author: | Aleksandar Sandro Cvetković |
Published: | January 14, 2024 |
Last Updated: | January 22, 2024 |
Downloads: | 732 |
License: | MIT |
Edit Code online: |
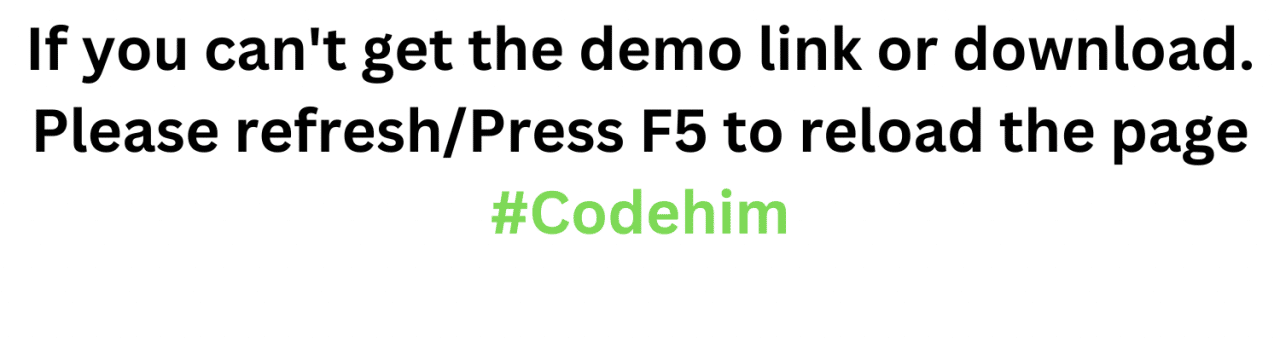
This JavaScript code snippet helps you to create Text-to-Speech functionality on a webpage. It comes with a basic interface with input options for text, voice, pitch, and rate. When you press the “Speak” button, the text you enter is converted to speech using the selected voice, pitch, and rate. The “Stop” button halts speech output. It’s helpful for adding a text-to-speech feature to web applications.
You can use this code to implement text-to-speech functionality on your website. It adds an interactive and engaging feature, making your site more inclusive.
How to Create Text To Speech Functionality Using JavaScript
1. In your HTML file, create a structure for the TTS interface. You can use the following HTML code as a starting point. It includes input fields for text, voice selection, pitch, and rate, along with buttons for speaking and stopping the speech.
2. Apply the following CSS code to style the TTS interface. This will make it visually appealing and user-friendly. You can further customize the CSS to match your website’s design.
3. Finally, add the following JavaScript code to your project. It sets up the SpeechSynthesis API to handle text-to-speech. It also populates the voice selection dropdown with available voices. You can further customize the code to modify voice options or enhance user interactions.
That’s all! hopefully, you have successfully created Text-to-Speech feature using JavaScript. If you have any questions or suggestions, feel free to comment below.
Similar Code Snippets:
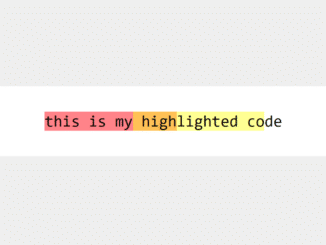
I code and create web elements for amazing people around the world. I like work with new people. New people new Experiences. I truly enjoy what I’m doing, which makes me more passionate about web development and coding. I am always ready to do challenging tasks whether it is about creating a custom CMS from scratch or customizing an existing system.
Leave a Comment Cancel reply
This site uses Akismet to reduce spam. Learn how your comment data is processed .
Free Web Design Code & Scripts - CodeHim is one of the BEST developer websites that provide web designers and developers with a simple way to preview and download a variety of free code & scripts. All codes published on CodeHim are open source, distributed under OSD-compliant license which grants all the rights to use, study, change and share the software in modified and unmodified form. Before publishing, we test and review each code snippet to avoid errors, but we cannot warrant the full correctness of all content. All trademarks, trade names, logos, and icons are the property of their respective owners... find out more...
Please Rel0ad/PressF5 this page if you can't click the download/preview link
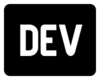
DEV Community

Posted on Jan 20, 2023
Text To Speech Converter with JavaScript
Converting text to speech using HTML, CSS, and JavaScript can be done using the SpeechSynthesis API.
The SpeechSynthesis API is a built-in JavaScript API that allows you to convert text to speech directly in the browser without the need for any external libraries.
Here is an example of how to use the SpeechSynthesis API to convert text to speech in HTML, CSS, and JavaScript:
JavaScript Code
This example creates a text area and a button in the HTML, styles them using CSS, and then uses JavaScript to add an event listener to the button that converts the text in the text area to speech when the button is clicked.
This is just a basic example and you can customize it further according to your needs by, for example, adding more options to the speech synthesis and also you can use libraries such as responsivevoice.js, meSpeak.js or annyang.js to add more functionalities and features.
Top comments (12)
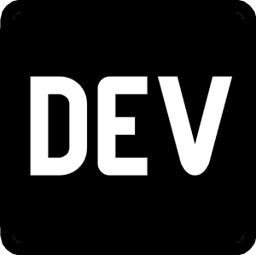
Templates let you quickly answer FAQs or store snippets for re-use.

- Email [email protected]
- Location London
- Education YouTube
- Work Developer
- Joined Nov 17, 2017
Nice post :) And nicely explained, thanks for sharing.
There's loads of project ideas you could make with this!
I made one a while back, that shows real-time analytics based on your speech
Lissy93 / realtime-speech-analytics
🗣️ real-time visual analytics and entity extraction of live speech, 🗣️ real-time speach analytics.
Calculates and renders live speach insights, to help you understand the meaning and tone behind spoken language Useful for generating meeting notes, inteligent subtitles, practicing speaches, language translation, etc
This is a simple node app, which listens for speach (of any type), and in realtime calculates insightful stats from it, then generates a series of visual analytics in D3.js, which are updated live, as speach comes in.
This includes, current sentiment, most commonly used words, pace of speach, key entities that are being mentioned, and more The technology developed can be put into use in areas, such as speach coaching, meeting notes, inteligent subtitles, learning languages, live news analysis, gauging consumer feedback on review videos, sports commentry etc...
Initially developed as a prototype, by myself (Alicia Sykes), and Oliver Poole (in 24 hours!) during AngelHack London 2016.
There is a live demo running here …

- Location Dallas, TX
- Joined Jan 18, 2022
The Fediverse platform I wrote, Quanta, does TTS too. I love it. It's using the javascript API...
Here's the Code

- Joined Apr 26, 2023
This can be useful for people with reading disabilities, as well as for those who want to listen to text instead of reading. You can do anything with a Java script. I find informative speech topics so far, use gradesfixer.com/blog/150-sports-in... for that. Using libraries such as SpeechSynthesis, you can create a script that will convert text to speech and speak it. It just sounds unrealistic, but this future has already arrived and we are living it.

- Joined Jul 3, 2023

- Email [email protected]
- Location India
- Education Gurugram
- Pronouns he/him
- Work Student
- Joined Nov 15, 2021
Small and easy yet amazing project! Thanks for sharing 🙃

- Location india
- Joined May 11, 2021

- Education In a High school in the US
- Joined Nov 29, 2022
A great project idea! :)

- Email [email protected]
- Location Chattogram, Bangladeh
- Education Changzhou University, Jiangsu, China
- Work Employee at Outshade Digital Media Private Limited
- Joined Dec 6, 2022
Beautifully explained with simple plain words. Loved that.

- Work Three.js at Maad
- Joined Jul 3, 2021
Nice share, can download the voice

- Joined May 30, 2023
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse


Mastering React Components
M.Ark - Jun 12

Top 20 Awesome on Github
Antonio | CEO at Litlyx - Jun 12
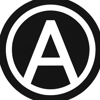
Securing Firebase Connections in Next.js with HTTPS
Antoine - Jun 12

How to start building with new customer accounts in Shopify
Gadget - Jun 11
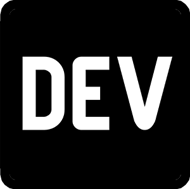
We're a place where coders share, stay up-to-date and grow their careers.
JavaScript Speech Recognition Example (Speech to Text)
With the Web Speech API, we can recognize speech using JavaScript . It is super easy to recognize speech in a browser using JavaScript and then getting the text from the speech to use as user input. We have already covered How to convert Text to Speech in Javascript .
But the support for this API is limited to the Chrome browser only . So if you are viewing this example in some other browser, the live example below might not work.

This tutorial will cover a basic example where we will cover speech to text. We will ask the user to speak something and we will use the SpeechRecognition object to convert the speech into text and then display the text on the screen.
The Web Speech API of Javascript can be used for multiple other use cases. We can provide a list of rules for words or sentences as grammar using the SpeechGrammarList object, which will be used to recognize and validate user input from speech.
For example, consider that you have a webpage on which you show a Quiz, with a question and 4 available options and the user has to select the correct option. In this, we can set the grammar for speech recognition with only the options for the question, hence whatever the user speaks, if it is not one of the 4 options, it will not be recognized.
We can use grammar, to define rules for speech recognition, configuring what our app understands and what it doesn't understand.
JavaScript Speech to Text
In the code example below, we will use the SpeechRecognition object. We haven't used too many properties and are relying on the default values. We have a simple HTML webpage in the example, where we have a button to initiate the speech recognition.
The main JavaScript code which is listening to what user speaks and then converting it to text is this:
In the above code, we have used:
recognition.start() method is used to start the speech recognition.
Once we begin speech recognition, the onstart event handler can be used to inform the user that speech recognition has started and they should speak into the mocrophone.
When the user is done speaking, the onresult event handler will have the result. The SpeechRecognitionEvent results property returns a SpeechRecognitionResultList object. The SpeechRecognitionResultList object contains SpeechRecognitionResult objects. It has a getter so it can be accessed like an array. The first [0] returns the SpeechRecognitionResult at the last position. Each SpeechRecognitionResult object contains SpeechRecognitionAlternative objects that contain individual results. These also have getters so they can be accessed like arrays. The second [0] returns the SpeechRecognitionAlternative at position 0 . We then return the transcript property of the SpeechRecognitionAlternative object.
Same is done for the confidence property to get the accuracy of the result as evaluated by the API.
We have many event handlers, to handle the events surrounding the speech recognition process. One such event is onspeechend , which we have used in our code to call the stop() method of the SpeechRecognition object to stop the recognition process.
Now let's see the running code:
When you will run the code, the browser will ask for permission to use your Microphone , so please click on Allow and then speak anything to see the script in action.
Conclusion:
So in this tutorial we learned how we can use Javascript to write our own small application for converting speech into text and then displaying the text output on screen. We also made the whole process more interactive by using the various event handlers available in the SpeechRecognition interface. In future I will try to cover some simple web application ideas using this feature of Javascript to help you usnderstand where we can use this feature.
If you face any issue running the above script, post in the comment section below. Remember, only Chrome browser supports it .
You may also like:
- JavaScript Window Object
- JavaScript Number Object
- JavaScript Functions
- JavaScript Document Object
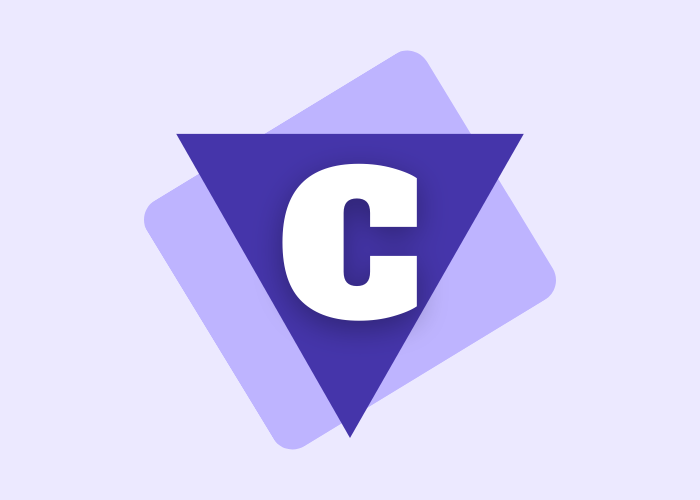
IF YOU LIKE IT, THEN SHARE IT
Related posts.
- HTML Tutorial
- HTML Exercises
- HTML Attributes
- Global Attributes
- Event Attributes
- HTML Interview Questions
- DOM Audio/Video
- HTML Examples
- Color Picker
- A to Z Guide
- HTML Formatter
How to convert speech into text using JavaScript ?
- Build a Text to Speech Converter using HTML, CSS & Javascript
- How to convert JSON results into a date using JavaScript ?
- How to make a text italic using JavaScript ?
- How to align text content into center using JavaScript ?
- How to convert text to speech in Node.js ?
- How to make a word count in textarea using JavaScript ?
- How to convert an object to string using JavaScript ?
- How to convert image into base64 string using JavaScript ?
- How to Convert JSON to string in JavaScript ?
- How to Convert a String to Uppercase in JavaScript ?
- How to change the shape of a textarea using JavaScript ?
- How to Convert Char to String in JavaScript ?
- How to Convert String of Objects to Array in JavaScript ?
- How to convert string into float in JavaScript?
- How to create Text Editor using Javascript and HTML ?
- Converting Text to Speech in Java
- How to Convert Text to Speech in Android using Kotlin?
- Convert Text to Speech in Python
- Text to speech GUI convertor using Tkinter in Python
In this article, we will learn to convert speech into text using HTML and JavaScript.
Approach: We added a content editable “div” by which we make any HTML element editable.
We use the SpeechRecognition object to convert the speech into text and then display the text on the screen.
We also added WebKit Speech Recognition to perform speech recognition in Google chrome and Apple safari.
InterimResults results should be returned true and the default value of this is false. So set interimResults= true
Use appendChild() method to append a node as the last child of a node.
Add eventListener, in this event listener, map() method is used to create a new array with the results of calling a function for every array element.
Note: This method does not change the original array.
Use join() method to return array as a string.
Final Code:
Output:
If the user tells “Hello World” after running the file, it shows the following on the screen.
Please Login to comment...
Similar reads.
- JavaScript-Misc
- Technical Scripter 2020
- Technical Scripter
- Web Technologies
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Stack Overflow Public questions & answers
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Talent Build your employer brand
- Advertising Reach developers & technologists worldwide
- Labs The future of collective knowledge sharing
- About the company
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Using Speech Synthesis and Web Audio API to visualize text-to-speech
I am trying to create an audio visualizer that takes the text-to-speech and visualizes as it is being spoken. I was able to use this as a baseline to start with. I have sucessfully implemented a text to speech feature in the top left corner but now I am struggling to connect the two. This is the code that I started with:
This is what I have for text-to-speech.
Have been reading on Speech Synthesis and Web Audio API but at a loss for how the nodes are connected. The mp3 is read in as one continous thing while the tts splices everything to be successfully read.
- text-to-speech
- web-audio-api
Know someone who can answer? Share a link to this question via email , Twitter , or Facebook .
Your answer.
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Browse other questions tagged audio three.js text-to-speech web-audio-api or ask your own question .
- Featured on Meta
- Upcoming sign-up experiments related to tags
- Policy: Generative AI (e.g., ChatGPT) is banned
- The return of Staging Ground to Stack Overflow
- The 2024 Developer Survey Is Live
Hot Network Questions
- Looking at buying house with mechanical septic system. What questions should I be asking?
- What type of aircraft is this?
- Falling damage based on creature size
- Quote identification: Schindler war ein kleiner Rabe
- Is a Poisson minus a constant still a Poisson?
- Expected Value Chi Square distribution
- How is the progress field of Bitcoin Core's synchronisation/Initial Block Download debug logs calculated?
- Do we need a risk premium for the assets in the binomial option pricing model?
- What is the most efficient way to fillna multiple columns with values from other columns in a way that they can be paired with a suffix?
- Applying an IES texture to mesh light
- Who was responsible for adding words into John 1:18?
- Is there any position where getting checkmated by En Passant is a brilliant move on Chess.com?
- I will not raise my voice to him ever again
- Is it known that the the sequence 7n+1 would diverge starting with 7?
- TV-L 13 Classification (stufe) for PhD with Prior Industry Experience
- Found possible instance of plagiarism in joint review paper and PhD thesis of high profile collaborator, what to do?
- Is there anyway to estimate the time average power without knowing the power factor?
- Can you make a logo very similar to an existing trademark?
- How does the IC direct supplement work on NS / Dutch railways?
- How to scan "ac veluti magno in populo ..."?
- Help me understand what happened and how the machine left works
- Network devices cannot ping subnetwork devices
- I buy retrocomputing devices, but they set my allergies off. Any advice on sanitizing new acquisitions?
- How interrupt one of two crossing curved paths
Hello World! Online Javascript playground for free.
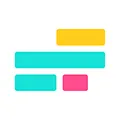
TEXT TO SPEECH VIDEO MAKER
Discover a variety of state-of-the-art voices powered by AI. Try out different voices with a built-in audio library of realistic, premium TTS voices.
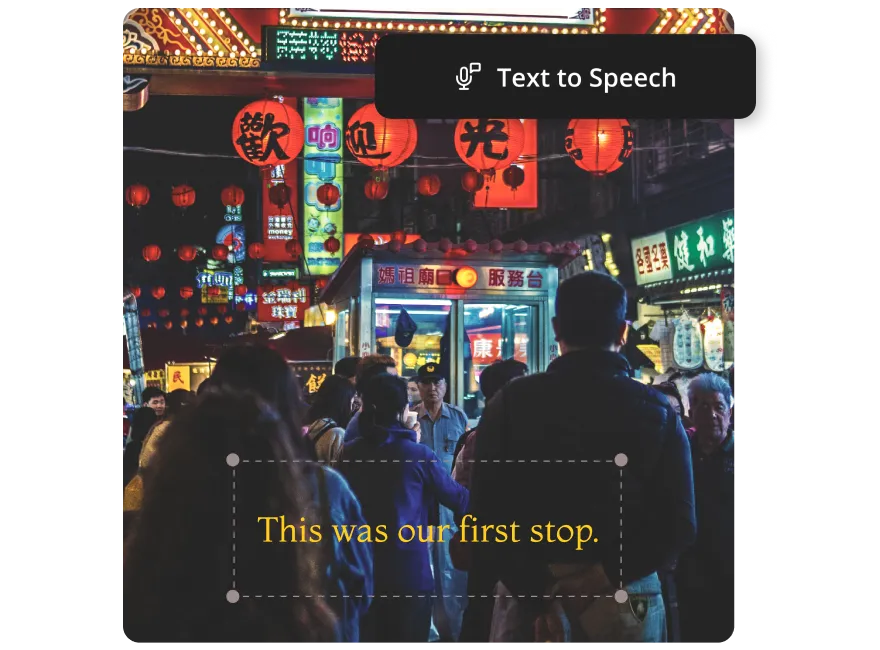
Turn written text into spoken word with text to speech videos
Explore a variety of premium male and female voices.
Seeking out natural sounding voice overs can be time-consuming. Discover realistic, human-like AI voices with Kapwing's built-in audio library making it super easy to try different types of voice overs.
Cut costs in half and convert text to voice in-house
It can be overwhelming to search for the right agency or partner to convert text to voice for every video project, let alone handling introduction calls to get to know the partner better.
Empower your own team to create text to speech videos themselves. With an all-in-one platform for video editing, creation, and collaboration, your team is well-equipped to convert text to speech—all without having to outsource a video editing professional.
Translate text into different languages
Growing your audience is an achievement, until you find most of your new audience's primary language is not the same as your own. Reach a wider audience by translating your text to speech videos into multiple languages such as Spanish, Arabic, German, and much more.
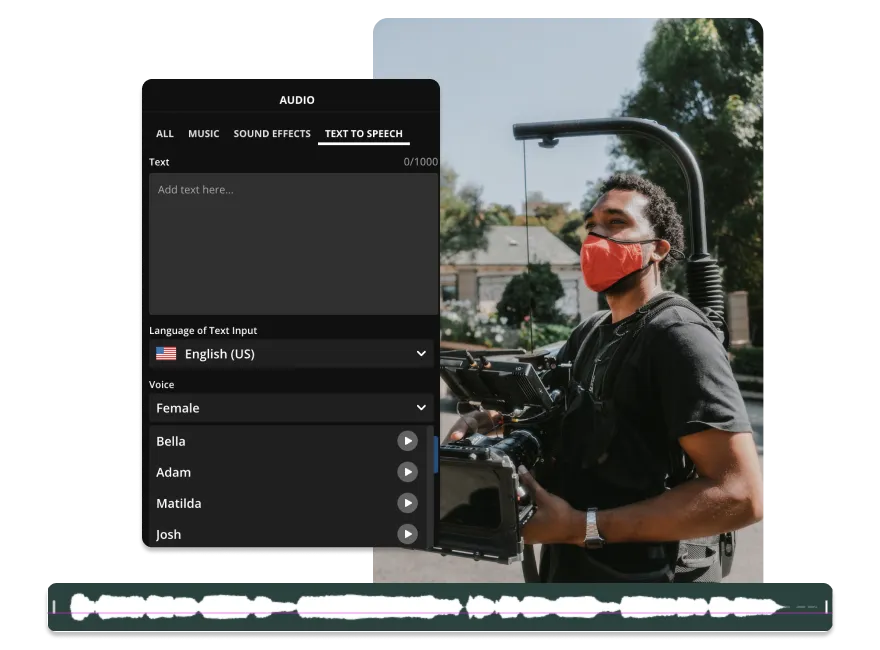
How to Make Text to Speech Videos
Start a new video project by opening a blank canvas in Kapwing. Upload a video file directly from your device, or paste a video URL link.
Open the "Text" tab in the left-hand sidebar and add text to video. With a text layer selected, open the "Effects" tab in the right-hand sidebar and select "Text to Speech." Choose the output language and an accent. (TIP): If you already have a voice over (VO) audio, generate subtitles and turn all text to speech automatically.
Make any additional edits and add transitions, Click “Export project” and your final text to speech video will be ready for you to download in seconds. Share with anyone online on all social media platforms.
Upgrade your video content with premium TTS voices
What is text to speech.
Text-to-Speech (TTS) is a type of assistive technology that reads digital text aloud, so the user can understand and enjoy the content they’re watching regardless of any visual impairments. In short, this process takes text and turns it into an audio file to add in video clips.
Promote accessibility with visual and auditory aids
Cover all grounds of assistive tech to support viewers who need visual or auditory support. Text to Speech provides visual learners with text to follow along with while also tending to auditory learners with audio tracks.
Explore a wide range of video editing tools
Record your own voice or screen on just one platform. With Kapwing, you can add narration or a voiceover to a screen recording and edit your video all in one place.
Simplify the video creation process with AI
It can be overwhelming to create videos in a crowded video editor with advanced features. Speed up your content creation process with Kapwing's AI Video Editor powered by more user-friendly tools to polish and create professional looking videos for any goal.
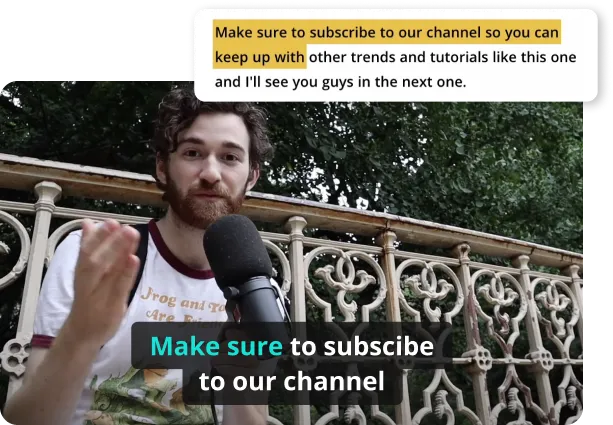
Frequently Asked Questions
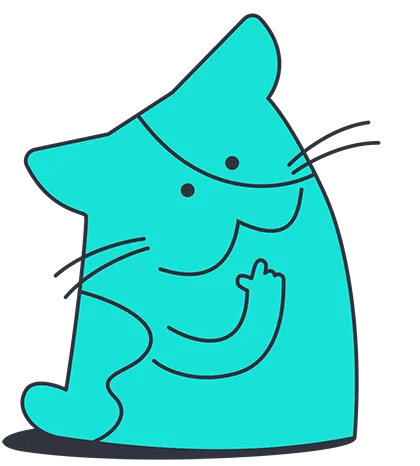
How do I use text to speech on a video?
You can add text to speech to video by using a text-to-speech generator or a video editor that offers a text-to-speech feature. Kapwing has a Text-to-Speech Video Maker that you can use easily online. Because of its intuitive interface, you can add text to speech to your video in just a few clicks.
What’s the best free text to speech software for YouTube videos?
You can easily use text-to-speech voices for your YouTube videos by adding the audio files to your video during the editing process. Kapwing is an online video editor that allows you to generate text-to-speech and add it to your video in one place. Once you’re finished editing in Kapwing, you can post the video to social platforms like Facebook, Twitter, and TikTok.
What's different about Kapwing?
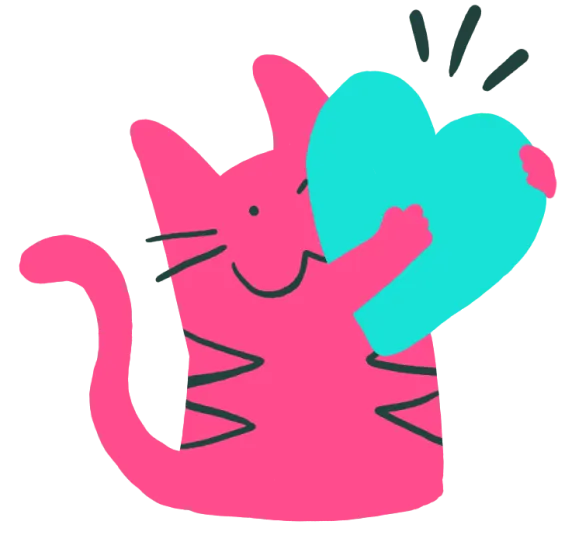
Kapwing is free to use for teams of any size. We also offer paid plans with additional features, storage, and support.
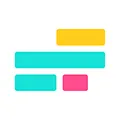
Easy Way to Learn Speech Recognition in Java With a Speech-To-Text API
Rev › Blog › Resources › Other Resources › Speech-to-Text APIs › Easy Way to Learn Speech Recognition in Java With a Speech-To-Text API
Here we explain show how to use a speech-to-text API with two Java examples.
We will be using the Rev AI API ( free for your first 5 hours ) that has two different speech-to-text API’s:
- Asynchronous API – For pre-recorded audio or video
- Streaming API – For live (streaming) audio or video
Asynchronous Rev AI API Java Code Example
We will use the Rev AI Java SDK located here . We use this short audio , on the exciting topic of HR recruiting.
First, sign up for Rev AI for free and get an access token.
Create a Java project with whatever editor you normally use. Then add this dependency to the Maven pom.xml manifest:
The code sample below is here . We explain it and show the output.
Submit the job from a URL:
Most of the Rev AI options are self-explanatory, for the most part. You can use the callback to kick off downloading the transcription in another program that is on standby, listening on http, if you don’t want to use the polling method we use in this example.
Put the program in a loop and check the job status. Download the transcription when it is done.
The SDK returns captions as well as text.
Here is the complete code:
It responds:
You can get the transcript with Java.
Or go get it later with curl, noting the job id from stdout above.
This returns the transcription in JSON format:
Streaming Rev AI API Java Code Example
A stream is a websocket connection from your video or audio server to the Rev AI audio-to-text entire.
We can emulate this connection by streaming a .raw file from the local hard drive to Rev AI.
One Ubuntu run:
Download the audio then convert it to .raw format as shown below. Converted it from wav to raw with the following ffmpeg command:
As you run that is gives key information about the audio file:
To explain, first we set a websocket connection and start streaming the file:
The important items to set here are the sampling rate (not bit rate) and format. We match this information from ffmpeg: Audio: pcm_f32le, 48000 Hz ,
After the client connects, the onConnected event sends a message. We can get the jobid from there. This will let us download the transcription later if we don’t want to get it in real-time.
To get the transcription in real time, listen for the onHypothesis event:
Here is what the output looks like:
What is the Best Speech Recognition API for Java?
Accuracy is what you want in a speech-to-text API, and Rev AI is a one-of-a-kind speech-to-text API in that regard.
You might ask, “So what? Siri and Alexa already do speech-to-text, and Google has a speech cloud API.”
That’s true. But there’s one game-changing difference:
The data that powers Rev AI is manually collected and carefully edited . Rev pays 50,000 freelancers to transcribe audio & caption videos for its 99% accurate transcription & captioning services . Rev AI is trained with this human-sourced data, and this produces transcripts that are far more accurate than those compiled simply by collecting audio, as Siri and Alexa do.
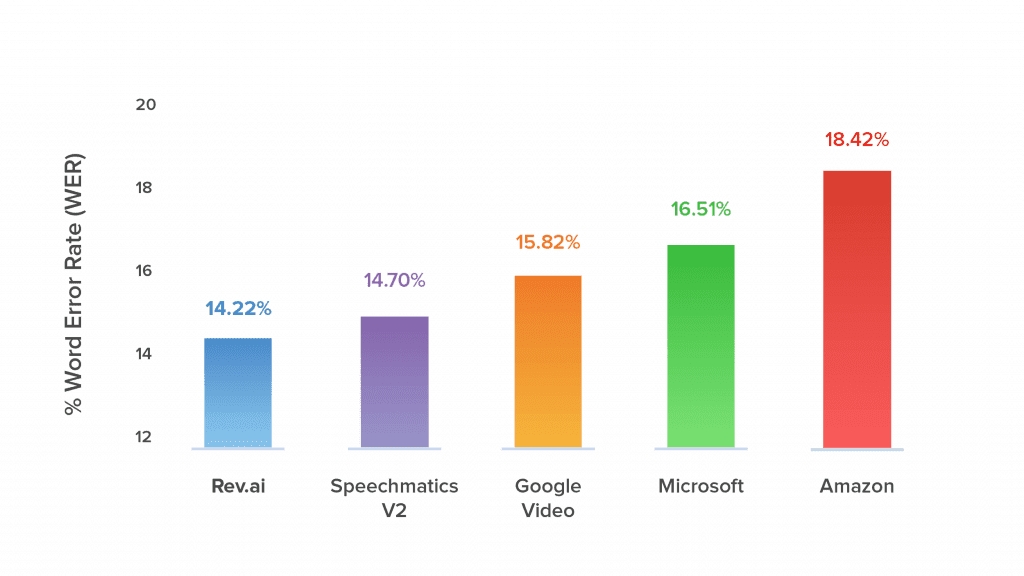
Rev AI’s accuracy is also snowballing, in a sense. Rev’s speech recognition system and API is constantly improving its accuracy rates as its dataset grows and the world-class engineers constantly improve the product.
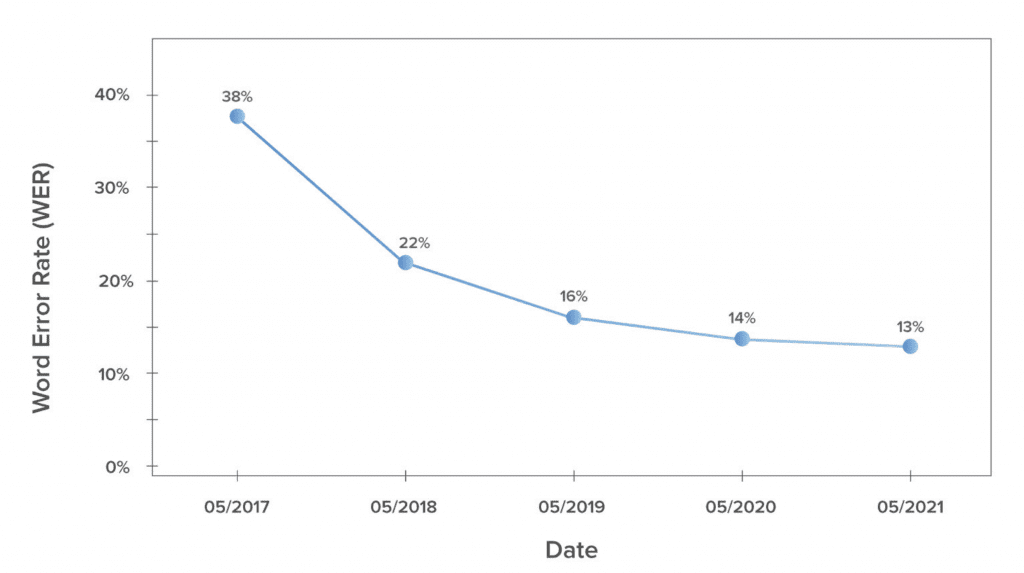
Labelled Data and Machine Learning
Why is human transcription important?
If you are familiar with machine learning then you know that converting audio to text is a classification problem.
To train the computer to transcribe audio ML programmers feed feature-label data into their model. This data is called a training set .
Features (sound) are input and labels (the corresponding letter) are output, calculated by the classification algorithm.
Alexa and Siri vacuum up this data all day long. So you would think they would have the largest and therefore most accurate training data.
But that’s only half of the equation. It takes many hours of manual work to type in the labels that correspond to the audio. In other words, a human must listen to the audio and type the corresponding letter and word.
This is what Rev AI has done.
It’s a business model that has taken off, because it fills a very specific need.
For example, look at closed captioning on YouTube. YouTube can automatically add captions to it’s audio. But it’s not always clear. You will notice that some of what it says is nonsense. It’s just like Google Translate: it works most of the time, but not all of the time.
The giant tech companies use statistical analysis, like the frequency distribution of words, to help their models.
But they are consistently outperformed by manually trained audio-to-voice training models.
More Caption & Subtitle Articles
Everybody’s Favorite Speech-to-Text Blog
We combine AI and a huge community of freelancers to make speech-to-text greatness every day. Wanna hear more about it?
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Português (do Brasil)
Text formatting
This chapter introduces how to work with strings and text in JavaScript.
JavaScript's String type is used to represent textual data. It is a set of "elements" of 16-bit unsigned integer values (UTF-16 code units). Each element in the String occupies a position in the String. The first element is at index 0, the next at index 1, and so on. The length of a String is the number of elements in it. You can create strings using string literals or string objects.
String literals
You can create simple strings using either single or double quotes:
More advanced strings can be created using escape sequences:
Hexadecimal escape sequences
The number after \x is interpreted as a hexadecimal number.
Unicode escape sequences
The Unicode escape sequences require at least four hexadecimal digits following \u .
Unicode code point escapes
With Unicode code point escapes, any character can be escaped using hexadecimal numbers so that it is possible to use Unicode code points up to 0x10FFFF . With simple Unicode escapes it is often necessary to write the surrogate halves separately to achieve the same result.
See also String.fromCodePoint() or String.prototype.codePointAt() .
String objects
The String object is a wrapper around the string primitive data type.
You can call any of the methods of the String object on a string literal value—JavaScript automatically converts the string literal to a temporary String object, calls the method, then discards the temporary String object. You can also use the length property with a string literal.
You should use string literals unless you specifically need to use a String object, because String objects can have counterintuitive behavior. For example:
A String object has one property, length , that indicates the number of UTF-16 code units in the string. For example, the following code assigns helloLength the value 13, because "Hello, World!" has 13 characters, each represented by one UTF-16 code unit. You can access each code unit using an array bracket style. You can't change individual characters because strings are immutable array-like objects:
Characters whose Unicode scalar values are greater than U+FFFF (such as some rare Chinese/Japanese/Korean/Vietnamese characters and some emoji) are stored in UTF-16 with two surrogate code units each. For example, a string containing the single character U+1F600 "Emoji grinning face" will have length 2. Accessing the individual code units in such a string using square brackets may have undesirable consequences such as the formation of strings with unmatched surrogate code units, in violation of the Unicode standard. (Examples should be added to this page after MDN bug 857438 is fixed.) See also String.fromCodePoint() or String.prototype.codePointAt() .
A String object has a variety of methods: for example those that return a variation on the string itself, such as substring and toUpperCase .
The following table summarizes the methods of String objects.
Method | Description |
---|---|
, , | Return the character or character code at the specified position in string. |
, | Return the position of specified substring in the string or last position of specified substring, respectively. |
, , | Returns whether or not the string starts, ends or contains a specified string. |
Combines the text of two strings and returns a new string. | |
Splits a object into an array of strings by separating the string into substrings. | |
Extracts a section of a string and returns a new string. | |
, | Return the specified subset of the string, either by specifying the start and end indexes or the start index and a length. |
, , , , | Work with regular expressions. |
, | Return the string in all lowercase or all uppercase, respectively. |
Returns the Unicode Normalization Form of the calling string value. | |
Returns a string consisting of the elements of the object repeated the given times. | |
Trims whitespace from the beginning and end of the string. |
Multi-line template literals
Template literals are string literals allowing embedded expressions. You can use multi-line strings and string interpolation features with them.
Template literals are enclosed by backtick ( grave accent ) characters ( ` ) instead of double or single quotes. Template literals can contain placeholders. These are indicated by the dollar sign and curly braces ( ${expression} ).
Multi-lines
Any new line characters inserted in the source are part of the template literal. Using normal strings, you would have to use the following syntax in order to get multi-line strings:
To get the same effect with multi-line strings, you can now write:
Embedded expressions
In order to embed expressions within normal strings, you would use the following syntax:
Now, with template literals, you are able to make use of the syntactic sugar making substitutions like this more readable:
For more information, read about Template literals in the JavaScript reference .
Internationalization
The Intl object is the namespace for the ECMAScript Internationalization API, which provides language sensitive string comparison, number formatting, and date and time formatting. The constructors for Intl.Collator , Intl.NumberFormat , and Intl.DateTimeFormat objects are properties of the Intl object.
Date and time formatting
The Intl.DateTimeFormat object is useful for formatting date and time. The following formats a date for English as used in the United States. (The result is different in another time zone.)
Number formatting
The Intl.NumberFormat object is useful for formatting numbers, for example currencies.
The Intl.Collator object is useful for comparing and sorting strings.
For example, there are actually two different sort orders in German, phonebook and dictionary . Phonebook sort emphasizes sound, and it's as if "ä", "ö", and so on were expanded to "ae", "oe", and so on prior to sorting.
Some German words conjugate with extra umlauts, so in dictionaries it's sensible to order ignoring umlauts (except when ordering words differing only by umlauts: schon before schön ).
For more information about the Intl API, see also Introducing the JavaScript Internationalization API .
Best text-to-speech software of 2024
Boosting accessibility and productivity
- Best overall
- Best realism
- Best for developers
- Best for podcasting
- How we test
The best text-to-speech software makes it simple and easy to convert text to voice for accessibility or for productivity applications.
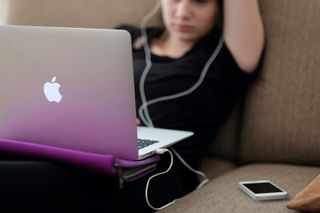
1. Best overall 2. Best realism 3. Best for developers 4. Best for podcasting 5. Best for developers 6. FAQs 7. How we test
Finding the best text-to-speech software is key for anyone looking to transform written text into spoken words, whether for accessibility purposes, productivity enhancement, or creative applications like voice-overs in videos.
Text-to-speech (TTS) technology relies on sophisticated algorithms to model natural language to bring written words to life, making it easier to catch typos or nuances in written content when it's read aloud. So, unlike the best speech-to-text apps and best dictation software , which focus on converting spoken words into text, TTS software specializes in the reverse process: turning text documents into audio. This technology is not only efficient but also comes with a variety of tools and features. For those creating content for platforms like YouTube , the ability to download audio files is a particularly valuable feature of the best text-to-speech software.
While some standard office programs like Microsoft Word and Google Docs offer basic TTS tools, they often lack the comprehensive functionalities found in dedicated TTS software. These basic tools may provide decent accuracy and basic options like different accents and languages, but they fall short in delivering the full spectrum of capabilities available in specialized TTS software.
To help you find the best text-to-speech software for your specific needs, TechRadar Pro has rigorously tested various software options, evaluating them based on user experience, performance, output quality, and pricing. This includes examining the best free text-to-speech software as well, since many free options are perfect for most users. We've brought together our picks below to help you choose the most suitable tool for your specific needs, whether for personal use, professional projects, or accessibility requirements.
The best text-to-speech software of 2024 in full:
Why you can trust TechRadar We spend hours testing every product or service we review, so you can be sure you’re buying the best. Find out more about how we test.
Below you'll find full write-ups for each of the entries on our best text-to-speech software list. We've tested each one extensively, so you can be sure that our recommendations can be trusted.
The best text-to-speech software overall
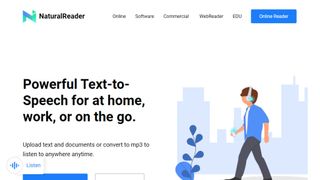
1. NaturalReader
Our expert review:
Reasons to buy
Reasons to avoid.
If you’re looking for a cloud-based speech synthesis application, you should definitely check out NaturalReader. Aimed more at personal use, the solution allows you to convert written text such as Word and PDF documents, ebooks and web pages into human-like speech.
Because the software is underpinned by cloud technology, you’re able to access it from wherever you go via a smartphone, tablet or computer. And just like Capti Voice, you can upload documents from cloud storage lockers such as Google Drive, Dropbox and OneDrive.
Currently, you can access 56 natural-sounding voices in nine different languages, including American English, British English, French, Spanish, German, Swedish, Italian, Portuguese and Dutch. The software supports PDF, TXT, DOC(X), ODT, PNG, JPG, plus non-DRM EPUB files and much more, along with MP3 audio streams.
There are three different products: online, software, and commercial. Both the online and software products have a free tier.
Read our full NaturalReader review .
- ^ Back to the top
The best text-to-speech software for realistic voices
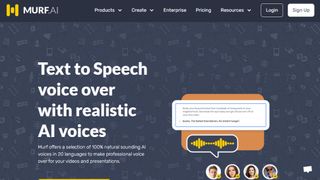
Specializing in voice synthesis technology, Murf uses AI to generate realistic voiceovers for a range of uses, from e-learning to corporate presentations.
Murf comes with a comprehensive suite of AI tools that are easy to use and straightforward to locate and access. There's even a Voice Changer feature that allows you to record something before it is transformed into an AI-generated voice- perfect if you don't think you have the right tone or accent for a piece of audio content but would rather not enlist the help of a voice actor. Other features include Voice Editing, Time Syncing, and a Grammar Assistant.
The solution comes with three pricing plans to choose from: Basic, Pro and Enterprise. The latter of these options may be pricey but some with added collaboration and account management features that larger companies may need access to. The Basic plan starts at around $19 / £17 / AU$28 per month but if you set up a yearly plan that will drop to around $13 / £12 / AU$20 per month. You can also try the service out for free for up to 10 minutes, without downloads.
The best text-to-speech software for developers
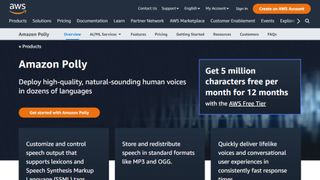
3. Amazon Polly
Alexa isn’t the only artificial intelligence tool created by tech giant Amazon as it also offers an intelligent text-to-speech system called Amazon Polly. Employing advanced deep learning techniques, the software turns text into lifelike speech. Developers can use the software to create speech-enabled products and apps.
It sports an API that lets you easily integrate speech synthesis capabilities into ebooks, articles and other media. What’s great is that Polly is so easy to use. To get text converted into speech, you just have to send it through the API, and it’ll send an audio stream straight back to your application.
You can also store audio streams as MP3, Vorbis and PCM file formats, and there’s support for a range of international languages and dialects. These include British English, American English, Australian English, French, German, Italian, Spanish, Dutch, Danish and Russian.
Polly is available as an API on its own, as well as a feature of the AWS Management Console and command-line interface. In terms of pricing, you’re charged based on the number of text characters you convert into speech. This is charged at approximately $16 per1 million characters , but there is a free tier for the first year.
The best text-to-speech software for podcasting
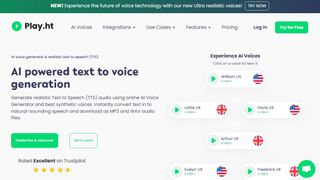
In terms of its library of voice options, it's hard to beat Play.ht as one of the best text-to-speech software tools. With almost 600 AI-generated voices available in over 60 languages, it's likely you'll be able to find a voice to suit your needs.
Although the platform isn't the easiest to use, there is a detailed video tutorial to help users if they encounter any difficulties. All the usual features are available, including Voice Generation and Audio Analytics.
In terms of pricing, Play.ht comes with four plans: Personal, Professional, Growth, and Business. These range widely in price, but it depends if you need things like commercial rights and affects the number of words you can generate each month.
The best text-to-speech software for Mac and iOS
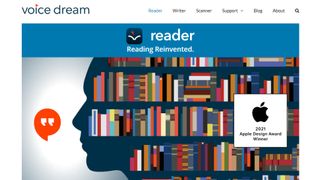
5. Voice Dream Reader
There are also plenty of great text-to-speech applications available for mobile devices, and Voice Dream Reader is an excellent example. It can convert documents, web articles and ebooks into natural-sounding speech.
The app comes with 186 built-in voices across 30 languages, including English, Arabic, Bulgarian, Catalan, Croatian, Czech, Danish, Dutch, Finnish, French, German, Greek, Hebrew, Hungarian, Italian, Japanese and Korean.
You can get the software to read a list of articles while you drive, work or exercise, and there are auto-scrolling, full-screen and distraction-free modes to help you focus. Voice Dream Reader can be used with cloud solutions like Dropbox, Google Drive, iCloud Drive, Pocket, Instapaper and Evernote.
The best text-to-speech software: FAQs
What is the best text-to-speech software for youtube.
If you're looking for the best text-to-speech software for YouTube videos or other social media platforms, you need a tool that lets you extract the audio file once your text document has been processed. Thankfully, that's most of them. So, the real trick is to select a TTS app that features a bountiful choice of natural-sounding voices that match the personality of your channel.
What’s the difference between web TTS services and TTS software?
Web TTS services are hosted on a company or developer website. You’ll only be able to access the service if the service remains available at the whim of a provider or isn’t facing an outage.
TTS software refers to downloadable desktop applications that typically won’t rely on connection to a server, meaning that so long as you preserve the installer, you should be able to use the software long after it stops being provided.
Do I need a text-to-speech subscription?
Subscriptions are by far the most common pricing model for top text-to-speech software. By offering subscription models for, companies and developers benefit from a more sustainable revenue stream than they do from simply offering a one-time purchase model. Subscription models are also attractive to text-to-speech software providers as they tend to be more effective at defeating piracy.
Free software options are very rarely absolutely free. In some cases, individual voices may be priced and sold individually once the application has been installed or an account has been created on the web service.
How can I incorporate text-to-speech as part of my business tech stack?
Some of the text-to-speech software that we’ve chosen come with business plans, offering features such as additional usage allowances and the ability to have a shared workspace for documents. Other than that, services such as Amazon Polly are available as an API for more direct integration with business workflows.
Small businesses may find consumer-level subscription plans for text-to-speech software to be adequate, but it’s worth mentioning that only business plans usually come with the universal right to use any files or audio created for commercial use.
How to choose the best text-to-speech software
When deciding which text-to-speech software is best for you, it depends on a number of factors and preferences. For example, whether you’re happy to join the ecosystem of big companies like Amazon in exchange for quality assurance, if you prefer realistic voices, and how much budget you’re playing with. It’s worth noting that the paid services we recommend, while reliable, are often subscription services, with software hosted via websites, rather than one-time purchase desktop apps.
Also, remember that the latest versions of Microsoft Word and Google Docs feature basic text-to-speech as standard, as well as most popular browsers. So, if you have access to that software and all you’re looking for is a quick fix, that may suit your needs well enough.
How we test the best text-to-speech software
We test for various use cases, including suitability for use with accessibility issues, such as visual impairment, and for multi-tasking. Both of these require easy access and near instantaneous processing. Where possible, we look for integration across the entirety of an operating system , and for fair usage allowances across free and paid subscription models.
At a minimum, we expect an intuitive interface and intuitive software. We like bells and whistles such as realistic voices, but we also appreciate that there is a place for products that simply get the job done. Here, the question that we ask can be as simple as “does this piece of software do what it's expected to do when asked?”
Read more on how we test, rate, and review products on TechRadar .
Get in touch
- Want to find out about commercial or marketing opportunities? Click here
- Out of date info, errors, complaints or broken links? Give us a nudge
- Got a suggestion for a product or service provider? Message us directly
- You've reached the end of the page. Jump back up to the top ^
Are you a pro? Subscribe to our newsletter
Sign up to the TechRadar Pro newsletter to get all the top news, opinion, features and guidance your business needs to succeed!
John (He/Him) is the Components Editor here at TechRadar and he is also a programmer, gamer, activist, and Brooklyn College alum currently living in Brooklyn, NY.
Named by the CTA as a CES 2020 Media Trailblazer for his science and technology reporting, John specializes in all areas of computer science, including industry news, hardware reviews, PC gaming, as well as general science writing and the social impact of the tech industry.
You can find him online on Threads @johnloeffler.
Currently playing: Baldur's Gate 3 (just like everyone else).
- Luke Hughes Staff Writer
- Steve Clark B2B Editor - Creative & Hardware
Copy My Data review: quickly transfer your content between devices
IDX data removal service review
You can now turn the Apple Vision Pro into a full-on film camera
Most Popular
- 2 Beelink GTi Ultra Mini PC is powered by an Intel 12th to 14th Gen Core CPU with support for external GPUs
- 3 Farewell, Nvidia GeForce Experience – you were a terrible app and I hated you, but at least something better is on the way
- 4 Trying to walk 10,000 steps a day? These three top tips from a walking expert will help you cross the finish line
- 5 How to unblock Netflix with a Smart TV
- 2 Blizzard saved a fortune by selling old WoW servers to fans
- 3 Intel reveals pricing for Gaudi 3 and Gaudi 2 AI accelerators will massively undercut Nvidia H100 GPU
- 4 Beelink GTi Ultra Mini PC is powered by an Intel 12th to 14th Gen Core CPU with support for external GPUs
- 5 If Apple adds this to Vision Pro, I might finally embrace it for good

IMAGES
VIDEO
COMMENTS
Yes, the Stone Age of the Internet is long over. Javascript has a native speechSynthesis text-to-speech API, and it will work so long as the browser and operating system support it. var msg = new SpeechSynthesisUtterance("MESSAGE"); speechSynthesis.speak(msg); That covers the quick basics, read on for more examples!
Step 1 - Setting Up The App. First, we set up a very basic application using a simple HTML file called index.html and a JavaScript file called script.js . We'll also use a CSS file called style.css to add some margins and to center things, but it's entirely up to you if you want to include this styling file.
Using the Web Speech API. The Web Speech API provides two distinct areas of functionality — speech recognition, and speech synthesis (also known as text to speech, or tts) — which open up interesting new possibilities for accessibility, and control mechanisms. This article provides a simple introduction to both areas, along with demos.
Text-to-Speech (TTS) technology enables computers to convert written text into spoken words. In the context of JavaScript, TTS allows developers to integrate speech synthesis capabilities directly into web applications. With TTS, users can interact with websites and applications using voice commands and receive audible feedback.
Line 1: We created a variable msg, and the value assigned to it is a new instance of the speechSynthesis class. Line 2: The .text property is used to specify the text we want to convert to speech. And finally, the code on the 3rd (last) line is what actually make our browser talks.
speech.lang = "en"; Text: The text property gets and sets the text that will be synthesized when the utterance is spoken. The text can be provided as plain text. In our case, the text property must be set when the start button is clicked. Let's add a click listener to the button.
The Speech Synthesis API is a JavaScript API that allows you to integrate text-to-speech (TTS) capabilities into web applications. It provides control over the voice, pitch, rate, and volume of the synthesized speech, offering flexibility in how the spoken output sounds. This API is supported in modern browsers like Chrome, Firefox, and Edge.
We will now start building our text-to-speech application. Before we begin, ensure that you have Node and npm installed on your machine. Run the following commands on your terminal to set up a project for the app and install the dependencies. Create a new project directory: mkdir web-speech-app.
This will begin the process of transforming the text into speech. Before calling this function, the text property must be set. If you start another text-to-speech instance when one is already running, the new one will be queued behind the current one. document.querySelector("#start").addEventListener("click", () => {.
Every article consists of text, which means that we can actually get our browser to read the text for us out loud by doing a bit of JavaScript. Let's dive into it. Text to speech with JavaScript. Before we can get this started, we need to have a webpage. For this basic example, we will create a simple HTML file:
Inside the inputForm.onsubmit handler, we stop the form submitting with preventDefault(), create a new SpeechSynthesisUtterance instance containing the text from the text <input>, set the utterance's voice to the voice selected in the <select> element, and start the utterance speaking via the SpeechSynthesis.speak() method.
This snippet is excerpted from our Speech synthesizer demo ( see it live ). When a form containing the text we want to speak is submitted, we (amongst other things) create a new utterance containing this text, then speak it by passing it into speak() as a parameter. const synth = window.speechSynthesis; // ...
You can use the SpeechSynthesisUtterance with a function like say:. function say(m) { var msg = new SpeechSynthesisUtterance(); var voices = window.speechSynthesis ...
Step 2 (CSS Code): Once the basic HTML structure of the text to speech converter is in place, the next step is to add styling to the text to speech converter using CSS. Next, we will create our CSS file. In this file, we will use some basic CSS rules to create our text to speech converter. The first section, * {}, is a universal selector that ...
Build a Text to Speech Converter using HTML, CSS & Javascript. A text-to-speech converter is an application that is used to convert the text content entered by the user into speech with a click of a button. A text-to-speech converter should have a text area at the top so that, the user can enter a long text to be converted into speech followed ...
Text-to-Speech (TTS) systems convert normal language text into speech. From now on we'll create a simple Text-to-Speech application using JavaScript, specifically using the Speech Synthesis interface of the Web Speech API. This interface is supported in almost all modern browsers and it's perfect for our application.
Begin by creating an HTML file and linking your JavaScript file using the script src tag. In your JavaScript file, initialize the speech synthesis object and set up an event listener for when the voices are ready. const synth = window.speechSynthesis; // Wait for voices to be loaded synth.onvoiceschanged = () => { const voices = synth.getVoices ...
This JavaScript code snippet helps you to create Text-to-Speech functionality on a webpage. It comes with a basic interface with input options for text, voice, pitch, and rate. When you press the "Speak" button, the text you enter is converted to speech using the selected voice, pitch, and rate. The "Stop" button halts speech output.
In this video, I have demonstrated with a code example how the Web Speech Api of JavaScript can be used to convert text to speech in web sites and web pages....
JavaScript Code. // Get the text area and speak button elements let textArea = document.getElementById("text"); let speakButton = document.getElementById("speak-button"); // Add an event listener to the speak button speakButton.addEventListener("click", function() { // Get the text from the text area let text = textArea.value; // Create a new ...
With the Web Speech API, we can recognize speech using JavaScript. It is super easy to recognize speech in a browser using JavaScript and then getting the text from the speech to use as user input. We have already covered How to convert Text to Speech in Javascript. But the support for this API is limited to the Chrome browser only. So if you ...
A text-to-speech converter is an application that is used to convert the text content entered by the user into speech with a click of a button. A text-to-speech converter should have a text area at the top so that, the user can enter a long text to be converted into speech followed by a button that converts the entered text into speech and plays th
I am trying to create an audio visualizer that takes the text-to-speech and visualizes as it is being spoken. I was able to use this as a baseline to start with. I have sucessfully implemented a text to speech feature in the top left corner but now I am struggling to connect the two. This is the code that I started with:
Opt out personal dataAgree and proceed. <!--. Online HTML, CSS and JavaScript editor to run code online. Write, edit and run HTML, CSS and JavaScript code online. Our HTML editor updates the webview automatically in real-time as you write code. Write and run HTML, CSS and JavaScript code using our online editor.
Open the "Text" tab in the left-hand sidebar and add text to video. With a text layer selected, open the "Effects" tab in the right-hand sidebar and select "Text to Speech." Choose the output language and an accent. (TIP): If you already have a voice over (VO) audio, generate subtitles and turn all text to speech automatically. Edit and export.
Here we explain show how to use a speech-to-text API with two Java examples. We will be using the Rev AI API ( free for your first 5 hours) that has two different speech-to-text API's: Asynchronous API - For pre-recorded audio or video. Streaming API - For live (streaming) audio or video. Find the Full Java SDK for the Rev AI API Here.
Using normal strings, you would have to use the following syntax in order to get multi-line strings: js. console.log( "string text line 1\n\. string text line 2", ); // "string text line 1 // string text line 2". To get the same effect with multi-line strings, you can now write: js. console.log(`string text line 1.
Dev focus. Alexa isn't the only artificial intelligence tool created by tech giant Amazon as it also offers an intelligent text-to-speech system called Amazon Polly. Employing advanced deep ...