[SOLVED] Local Variable Referenced Before Assignment
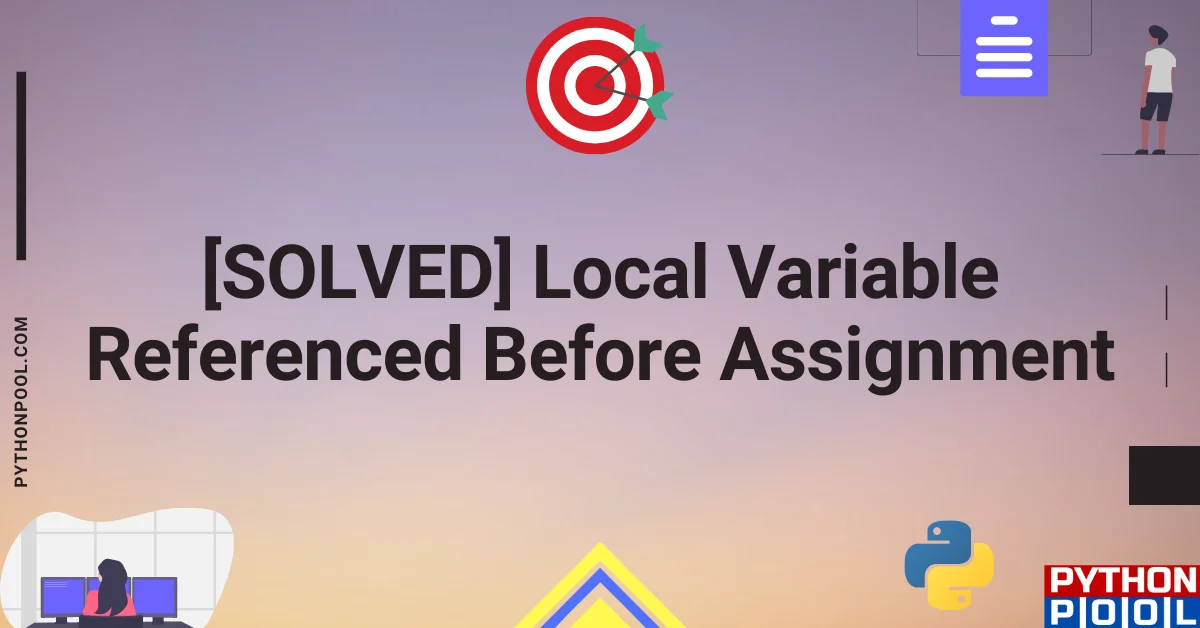
Python treats variables referenced only inside a function as global variables. Any variable assigned to a function’s body is assumed to be a local variable unless explicitly declared as global.

Why Does This Error Occur?
Unboundlocalerror: local variable referenced before assignment occurs when a variable is used before its created. Python does not have the concept of variable declarations. Hence it searches for the variable whenever used. When not found, it throws the error.
Before we hop into the solutions, let’s have a look at what is the global and local variables.
Local Variable Declarations vs. Global Variable Declarations
Local Variables | Global Variables |
---|---|
A variable is declared primarily within a Python function. | Global variables are in the global scope, outside a function. |
A local variable is created when the function is called and destroyed when the execution is finished. | A Variable is created upon execution and exists in memory till the program stops. |
Local Variables can only be accessed within their own function. | All functions of the program can access global variables. |
Local variables are immune to changes in the global scope. Thereby being more secure. | Global Variables are less safer from manipulation as they are accessible in the global scope. |
![unboundlocalerror local variable 'button' referenced before assignment [Fixed] typeerror can’t compare datetime.datetime to datetime.date](https://www.pythonpool.com/wp-content/uploads/2024/01/typeerror-cant-compare-datetime.datetime-to-datetime.date_-300x157.webp)
Local Variable Referenced Before Assignment Error with Explanation
Try these examples yourself using our Online Compiler.
Let’s look at the following function:

Explanation
The variable myVar has been assigned a value twice. Once before the declaration of myFunction and within myFunction itself.
Using Global Variables
Passing the variable as global allows the function to recognize the variable outside the function.
Create Functions that Take in Parameters
Instead of initializing myVar as a global or local variable, it can be passed to the function as a parameter. This removes the need to create a variable in memory.
UnboundLocalError: local variable ‘DISTRO_NAME’
This error may occur when trying to launch the Anaconda Navigator in Linux Systems.
Upon launching Anaconda Navigator, the opening screen freezes and doesn’t proceed to load.
Try and update your Anaconda Navigator with the following command.
If solution one doesn’t work, you have to edit a file located at
After finding and opening the Python file, make the following changes:
In the function on line 159, simply add the line:
DISTRO_NAME = None
Save the file and re-launch Anaconda Navigator.
DJANGO – Local Variable Referenced Before Assignment [Form]
The program takes information from a form filled out by a user. Accordingly, an email is sent using the information.
Upon running you get the following error:
We have created a class myForm that creates instances of Django forms. It extracts the user’s name, email, and message to be sent.
A function GetContact is created to use the information from the Django form and produce an email. It takes one request parameter. Prior to sending the email, the function verifies the validity of the form. Upon True , .get() function is passed to fetch the name, email, and message. Finally, the email sent via the send_mail function
Why does the error occur?
We are initializing form under the if request.method == “POST” condition statement. Using the GET request, our variable form doesn’t get defined.
Local variable Referenced before assignment but it is global
This is a common error that happens when we don’t provide a value to a variable and reference it. This can happen with local variables. Global variables can’t be assigned.
This error message is raised when a variable is referenced before it has been assigned a value within the local scope of a function, even though it is a global variable.
Here’s an example to help illustrate the problem:
In this example, x is a global variable that is defined outside of the function my_func(). However, when we try to print the value of x inside the function, we get a UnboundLocalError with the message “local variable ‘x’ referenced before assignment”.
This is because the += operator implicitly creates a local variable within the function’s scope, which shadows the global variable of the same name. Since we’re trying to access the value of x before it’s been assigned a value within the local scope, the interpreter raises an error.
To fix this, you can use the global keyword to explicitly refer to the global variable within the function’s scope:
However, in the above example, the global keyword tells Python that we want to modify the value of the global variable x, rather than creating a new local variable. This allows us to access and modify the global variable within the function’s scope, without causing any errors.
Local variable ‘version’ referenced before assignment ubuntu-drivers
This error occurs with Ubuntu version drivers. To solve this error, you can re-specify the version information and give a split as 2 –
Here, p_name means package name.
With the help of the threading module, you can avoid using global variables in multi-threading. Make sure you lock and release your threads correctly to avoid the race condition.
When a variable that is created locally is called before assigning, it results in Unbound Local Error in Python. The interpreter can’t track the variable.
Therefore, we have examined the local variable referenced before the assignment Exception in Python. The differences between a local and global variable declaration have been explained, and multiple solutions regarding the issue have been provided.
Trending Python Articles
![unboundlocalerror local variable 'button' referenced before assignment [Fixed] nameerror: name Unicode is not defined](https://www.pythonpool.com/wp-content/uploads/2024/01/Fixed-nameerror-name-Unicode-is-not-defined-300x157.webp)
Local variable referenced before assignment in Python
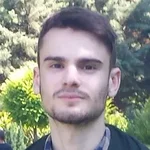
Last updated: Apr 8, 2024 Reading time · 4 min
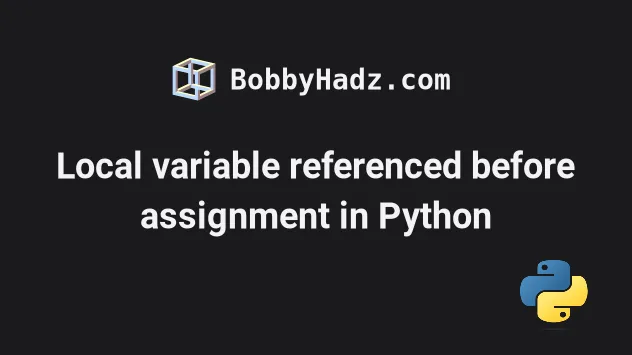
# Local variable referenced before assignment in Python
The Python "UnboundLocalError: Local variable referenced before assignment" occurs when we reference a local variable before assigning a value to it in a function.
To solve the error, mark the variable as global in the function definition, e.g. global my_var .
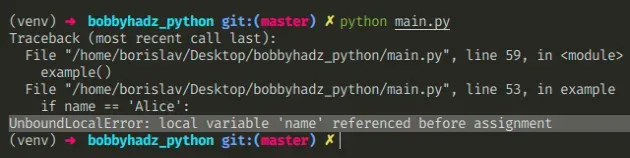
Here is an example of how the error occurs.
We assign a value to the name variable in the function.
# Mark the variable as global to solve the error
To solve the error, mark the variable as global in your function definition.
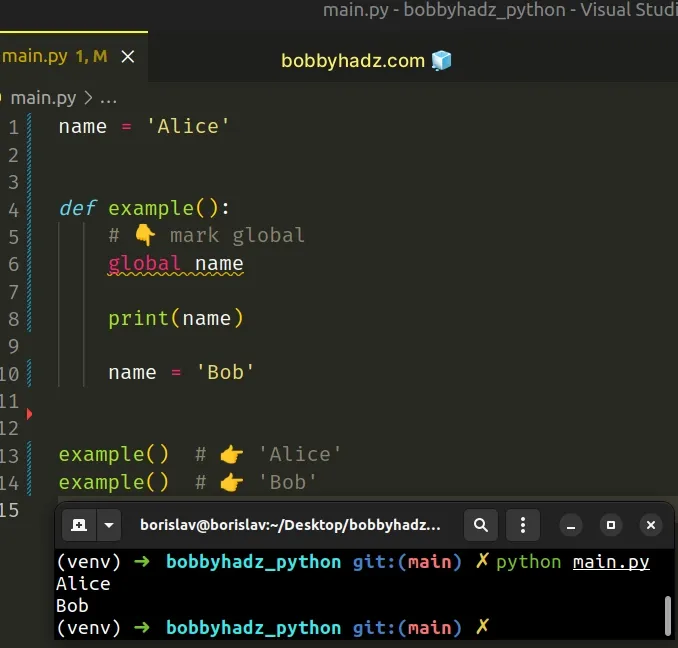
If a variable is assigned a value in a function's body, it is a local variable unless explicitly declared as global .
# Local variables shadow global ones with the same name
You could reference the global name variable from inside the function but if you assign a value to the variable in the function's body, the local variable shadows the global one.
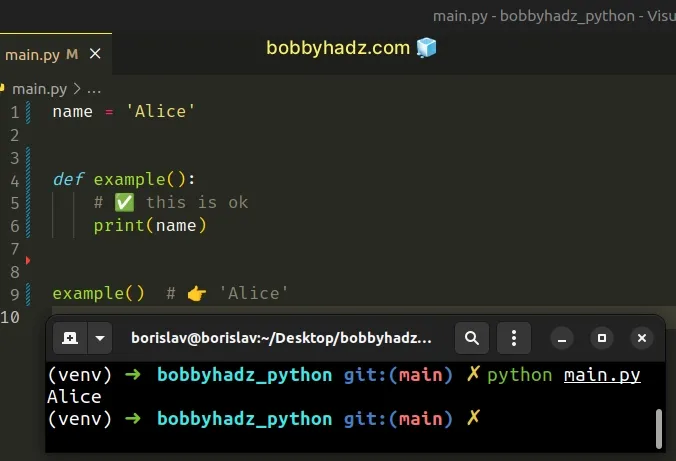
Accessing the name variable in the function is perfectly fine.
On the other hand, variables declared in a function cannot be accessed from the global scope.
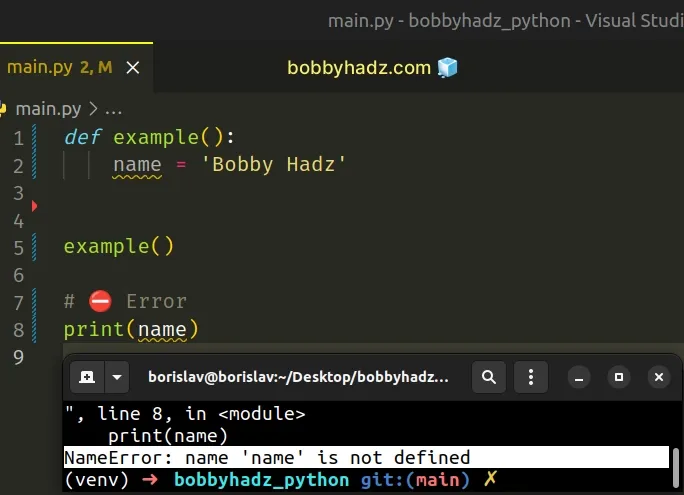
The name variable is declared in the function, so trying to access it from outside causes an error.
Make sure you don't try to access the variable before using the global keyword, otherwise, you'd get the SyntaxError: name 'X' is used prior to global declaration error.
# Returning a value from the function instead
An alternative solution to using the global keyword is to return a value from the function and use the value to reassign the global variable.
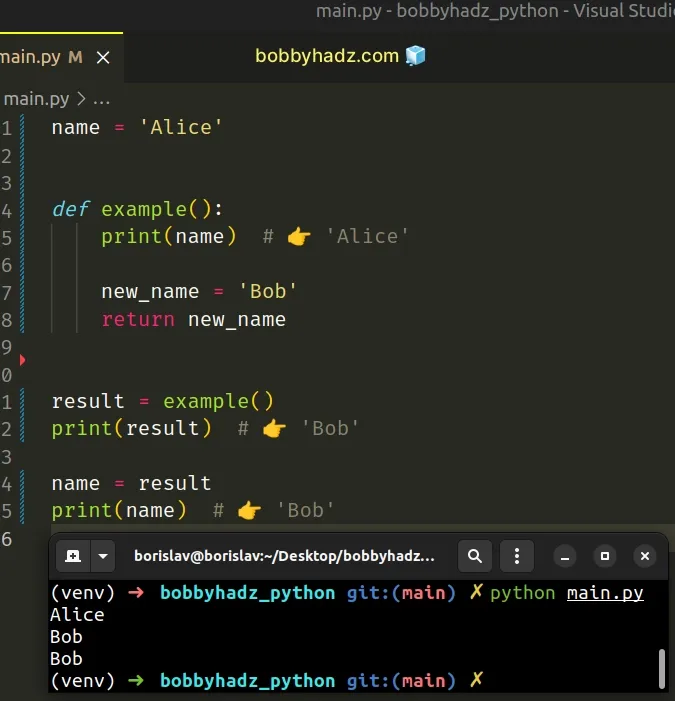
We simply return the value that we eventually use to assign to the name global variable.
# Passing the global variable as an argument to the function
You should also consider passing the global variable as an argument to the function.
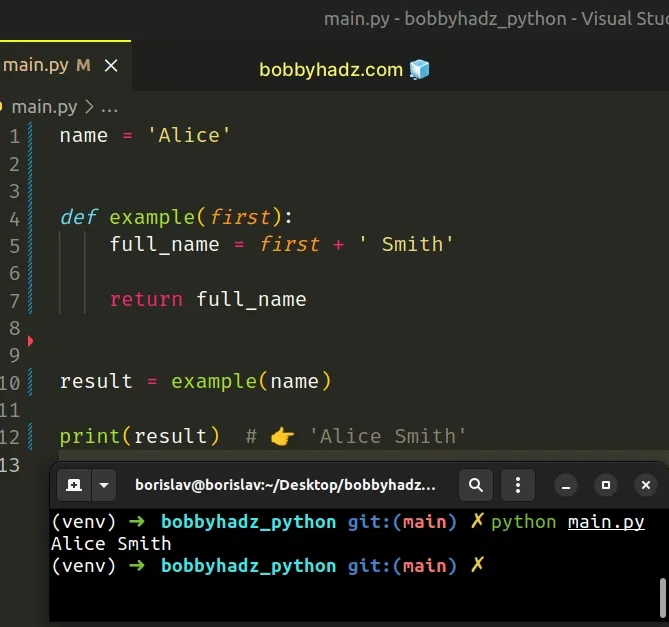
We passed the name global variable as an argument to the function.
If we assign a value to a variable in a function, the variable is assumed to be local unless explicitly declared as global .
# Assigning a value to a local variable from an outer scope
If you have a nested function and are trying to assign a value to the local variables from the outer function, use the nonlocal keyword.
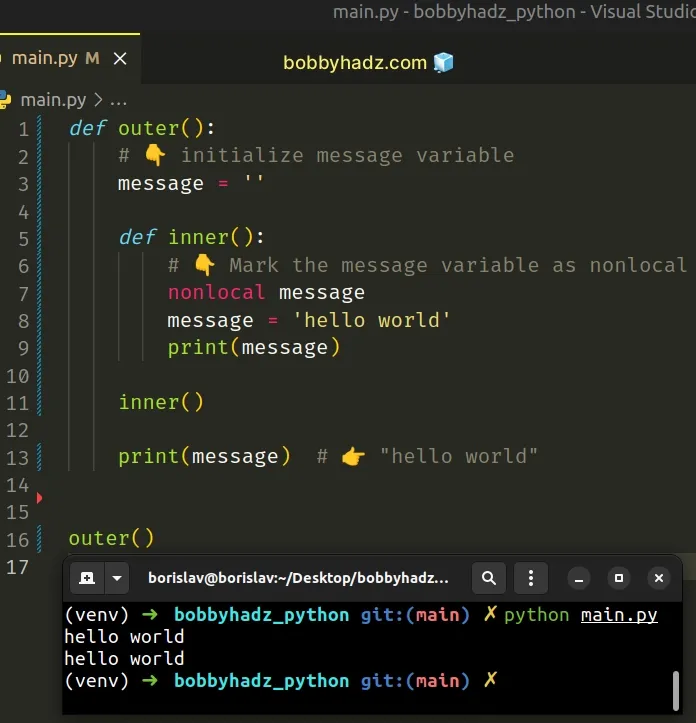
The nonlocal keyword allows us to work with the local variables of enclosing functions.
Had we not used the nonlocal statement, the call to the print() function would have returned an empty string.
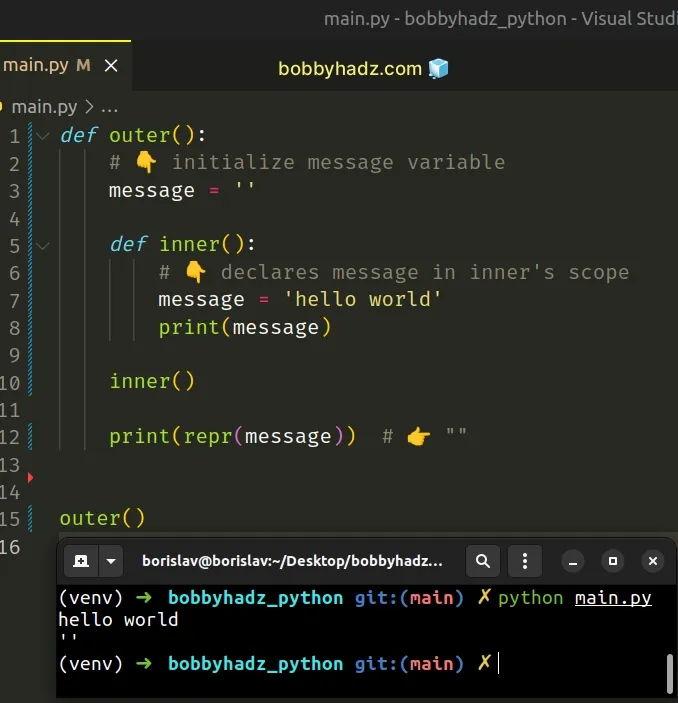
Printing the message variable on the last line of the function shows an empty string because the inner() function has its own scope.
Changing the value of the variable in the inner scope is not possible unless we use the nonlocal keyword.
Instead, the message variable in the inner function simply shadows the variable with the same name from the outer scope.
# Discussion
As shown in this section of the documentation, when you assign a value to a variable inside a function, the variable:
- Becomes local to the scope.
- Shadows any variables from the outer scope that have the same name.
The last line in the example function assigns a value to the name variable, marking it as a local variable and shadowing the name variable from the outer scope.
At the time the print(name) line runs, the name variable is not yet initialized, which causes the error.
The most intuitive way to solve the error is to use the global keyword.
The global keyword is used to indicate to Python that we are actually modifying the value of the name variable from the outer scope.
- If a variable is only referenced inside a function, it is implicitly global.
- If a variable is assigned a value inside a function's body, it is assumed to be local, unless explicitly marked as global .
If you want to read more about why this error occurs, check out [this section] ( this section ) of the docs.
# Additional Resources
You can learn more about the related topics by checking out the following tutorials:
- SyntaxError: name 'X' is used prior to global declaration
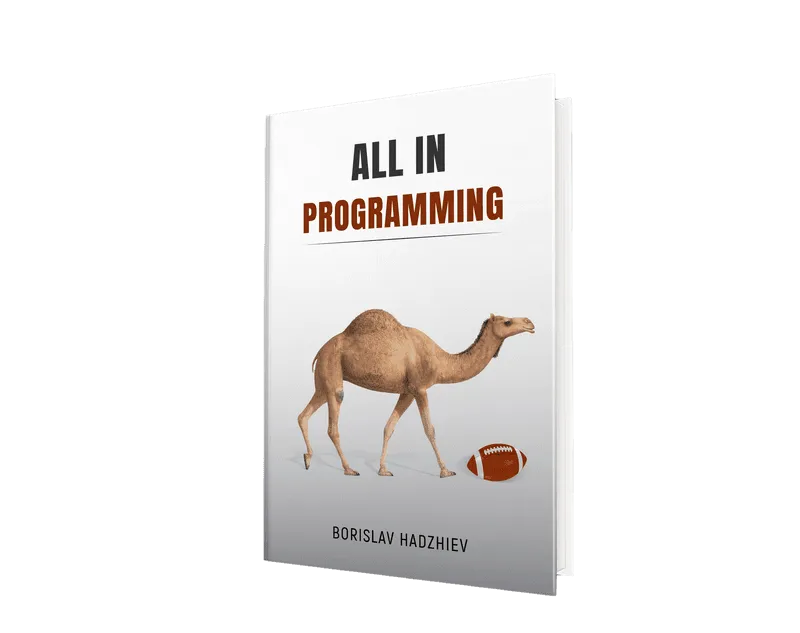
Borislav Hadzhiev
Web Developer
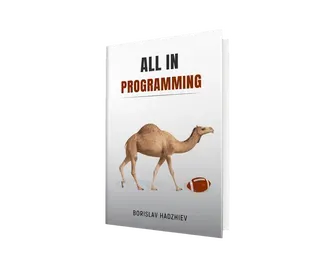
Copyright © 2024 Borislav Hadzhiev
How to fix UnboundLocalError: local variable 'x' referenced before assignment in Python
You could also see this error when you forget to pass the variable as an argument to your function.
How to reproduce this error
How to fix this error.
I hope this tutorial is useful. See you in other tutorials.
Take your skills to the next level ⚡️
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
UnboundLocalError Local variable Referenced Before Assignment in Python
- How to Fix - UnboundLocalError: Local variable Referenced Before Assignment in Python
- Python | Accessing variable value from code scope
- Access environment variable values in Python
- Get Variable Name As String In Python
- How to use Pickle to save and load Variables in Python?
- __file__ (A Special variable) in Python
- Undefined Variable Nameerror In Python
- Variables under the hood in Python
- How to Reference Elements in an Array in Python
- Difference between Local Variable and Global variable
- Unused local variable in Python
- Unused variable in for loop in Python
- Assign Function to a Variable in Python
- JavaScript ReferenceError - Can't access lexical declaration`variable' before initialization
- Global and Local Variables in Python
- Pass by reference vs value in Python
- __name__ (A Special variable) in Python
- PYTHONPATH Environment Variable in Python
- Julia local Keyword | Creating a local variable in Julia
Handling errors is an integral part of writing robust and reliable Python code. One common stumbling block that developers often encounter is the “UnboundLocalError” raised within a try-except block. This error can be perplexing for those unfamiliar with its nuances but fear not – in this article, we will delve into the intricacies of the UnboundLocalError and provide a comprehensive guide on how to effectively use try-except statements to resolve it.
What is UnboundLocalError Local variable Referenced Before Assignment in Python?
The UnboundLocalError occurs when a local variable is referenced before it has been assigned a value within a function or method. This error typically surfaces when utilizing try-except blocks to handle exceptions, creating a puzzle for developers trying to comprehend its origins and find a solution.
Why does UnboundLocalError: Local variable Referenced Before Assignment Occur?
below, are the reasons of occurring “Unboundlocalerror: Try Except Statements” in Python :
Variable Assignment Inside Try Block
Reassigning a global variable inside except block.
- Accessing a Variable Defined Inside an If Block
In the below code, example_function attempts to execute some_operation within a try-except block. If an exception occurs, it prints an error message. However, if no exception occurs, it prints the value of the variable result outside the try block, leading to an UnboundLocalError since result might not be defined if an exception was caught.
In below code , modify_global function attempts to increment the global variable global_var within a try block, but it raises an UnboundLocalError. This error occurs because the function treats global_var as a local variable due to the assignment operation within the try block.
Solution for UnboundLocalError Local variable Referenced Before Assignment
Below, are the approaches to solve “Unboundlocalerror: Try Except Statements”.
Initialize Variables Outside the Try Block
Avoid reassignment of global variables.
In modification to the example_function is correct. Initializing the variable result before the try block ensures that it exists even if an exception occurs within the try block. This helps prevent UnboundLocalError when trying to access result in the print statement outside the try block.
Below, code calculates a new value ( local_var ) based on the global variable and then prints both the local and global variables separately. It demonstrates that the global variable is accessed directly without being reassigned within the function.
In conclusion , To fix “UnboundLocalError” related to try-except statements, ensure that variables used within the try block are initialized before the try block starts. This can be achieved by declaring the variables with default values or assigning them None outside the try block. Additionally, when modifying global variables within a try block, use the `global` keyword to explicitly declare them.
Please Login to comment...
Similar reads.
- Python Errors
- Python Programs
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
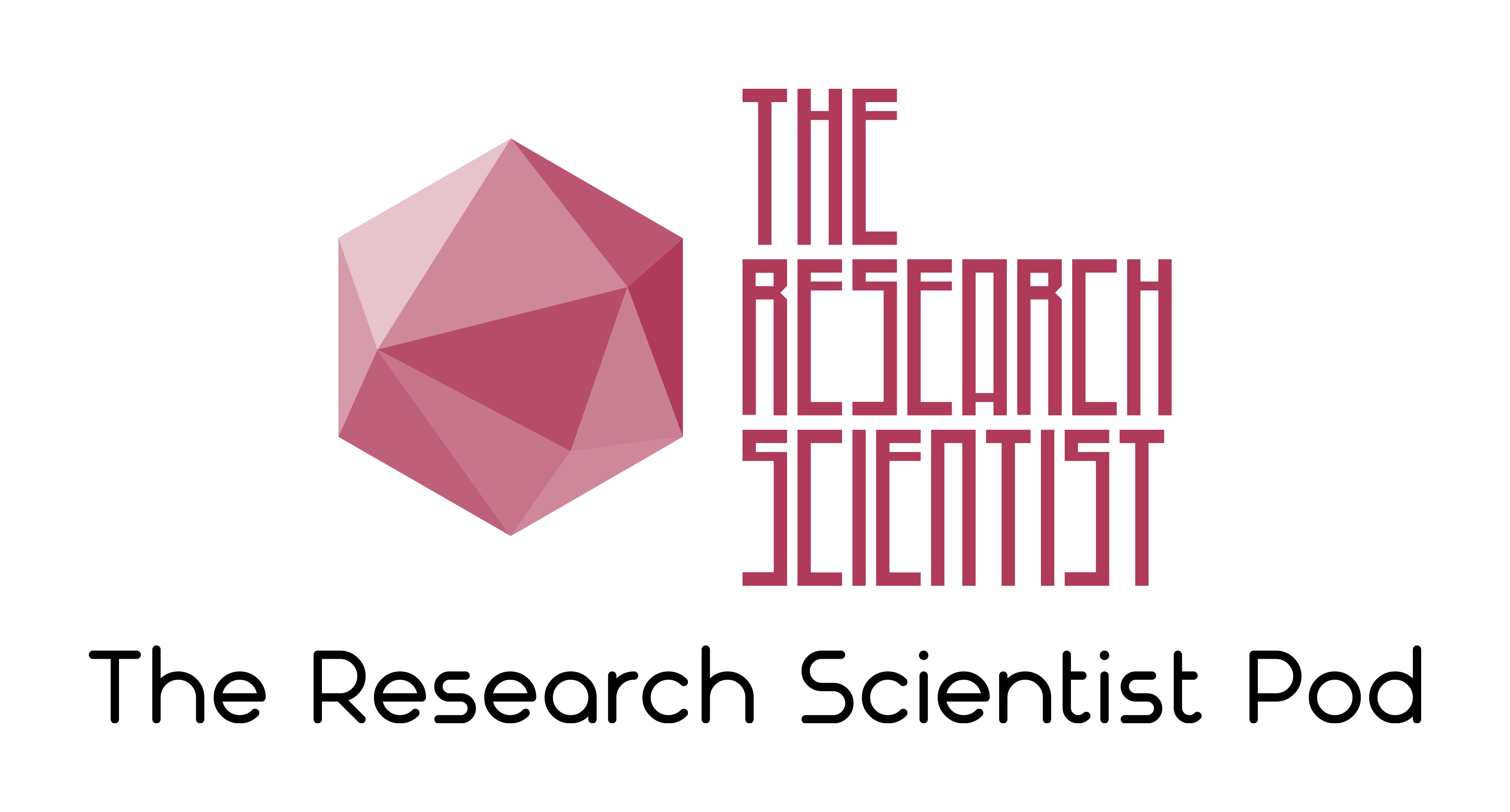
Python UnboundLocalError: local variable referenced before assignment
by Suf | Programming , Python , Tips
If you try to reference a local variable before assigning a value to it within the body of a function, you will encounter the UnboundLocalError: local variable referenced before assignment.
The preferable way to solve this error is to pass parameters to your function, for example:
Alternatively, you can declare the variable as global to access it while inside a function. For example,
This tutorial will go through the error in detail and how to solve it with code examples .

Table of contents
What is scope in python, unboundlocalerror: local variable referenced before assignment, solution #1: passing parameters to the function, solution #2: use global keyword, solution #1: include else statement, solution #2: use global keyword.
Scope refers to a variable being only available inside the region where it was created. A variable created inside a function belongs to the local scope of that function, and we can only use that variable inside that function.
A variable created in the main body of the Python code is a global variable and belongs to the global scope. Global variables are available within any scope, global and local.
UnboundLocalError occurs when we try to modify a variable defined as local before creating it. If we only need to read a variable within a function, we can do so without using the global keyword. Consider the following example that demonstrates a variable var created with global scope and accessed from test_func :
If we try to assign a value to var within test_func , the Python interpreter will raise the UnboundLocalError:
This error occurs because when we make an assignment to a variable in a scope, that variable becomes local to that scope and overrides any variable with the same name in the global or outer scope.
var +=1 is similar to var = var + 1 , therefore the Python interpreter should first read var , perform the addition and assign the value back to var .
var is a variable local to test_func , so the variable is read or referenced before we have assigned it. As a result, the Python interpreter raises the UnboundLocalError.
Example #1: Accessing a Local Variable
Let’s look at an example where we define a global variable number. We will use the increment_func to increase the numerical value of number by 1.
Let’s run the code to see what happens:
The error occurs because we tried to read a local variable before assigning a value to it.
We can solve this error by passing a parameter to increment_func . This solution is the preferred approach. Typically Python developers avoid declaring global variables unless they are necessary. Let’s look at the revised code:
We have assigned a value to number and passed it to the increment_func , which will resolve the UnboundLocalError. Let’s run the code to see the result:
We successfully printed the value to the console.
We also can solve this error by using the global keyword. The global statement tells the Python interpreter that inside increment_func , the variable number is a global variable even if we assign to it in increment_func . Let’s look at the revised code:
Let’s run the code to see the result:
Example #2: Function with if-elif statements
Let’s look at an example where we collect a score from a player of a game to rank their level of expertise. The variable we will use is called score and the calculate_level function takes in score as a parameter and returns a string containing the player’s level .
In the above code, we have a series of if-elif statements for assigning a string to the level variable. Let’s run the code to see what happens:
The error occurs because we input a score equal to 40 . The conditional statements in the function do not account for a value below 55 , therefore when we call the calculate_level function, Python will attempt to return level without any value assigned to it.
We can solve this error by completing the set of conditions with an else statement. The else statement will provide an assignment to level for all scores lower than 55 . Let’s look at the revised code:
In the above code, all scores below 55 are given the beginner level. Let’s run the code to see what happens:
We can also create a global variable level and then use the global keyword inside calculate_level . Using the global keyword will ensure that the variable is available in the local scope of the calculate_level function. Let’s look at the revised code.
In the above code, we put the global statement inside the function and at the beginning. Note that the “default” value of level is beginner and we do not include the else statement in the function. Let’s run the code to see the result:
Congratulations on reading to the end of this tutorial! The UnboundLocalError: local variable referenced before assignment occurs when you try to reference a local variable before assigning a value to it. Preferably, you can solve this error by passing parameters to your function. Alternatively, you can use the global keyword.
If you have if-elif statements in your code where you assign a value to a local variable and do not account for all outcomes, you may encounter this error. In which case, you must include an else statement to account for the missing outcome.
For further reading on Python code blocks and structure, go to the article: How to Solve Python IndentationError: unindent does not match any outer indentation level .
Go to the online courses page on Python to learn more about Python for data science and machine learning.
Have fun and happy researching!
Share this:
- Click to share on Facebook (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to share on Pinterest (Opens in new window)
- Click to share on Telegram (Opens in new window)
- Click to share on WhatsApp (Opens in new window)
- Click to share on Twitter (Opens in new window)
- Click to share on Tumblr (Opens in new window)
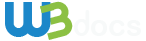
- Password Generator
- HTML Editor
- HTML Encoder
- JSON Beautifier
- CSS Beautifier
- Markdown Convertor
- Find the Closest Tailwind CSS Color
- Phrase encrypt / decrypt
- Browser Feature Detection
- Number convertor
- CSS Maker text shadow
- CSS Maker Text Rotation
- CSS Maker Out Line
- CSS Maker RGB Shadow
- CSS Maker Transform
- CSS Maker Font Face
- Color Picker
- Colors CMYK
- Color mixer
- Color Converter
- Color Contrast Analyzer
- Color Gradient
- String Length Calculator
- MD5 Hash Generator
- Sha256 Hash Generator
- String Reverse
- URL Encoder
- URL Decoder
- Base 64 Encoder
- Base 64 Decoder
- Extra Spaces Remover
- String to Lowercase
- String to Uppercase
- Word Count Calculator
- Empty Lines Remover
- HTML Tags Remover
- Binary to Hex
- Hex to Binary
- Rot13 Transform on a String
- String to Binary
- Duplicate Lines Remover
Python 3: UnboundLocalError: local variable referenced before assignment
This error occurs when you are trying to access a variable before it has been assigned a value. Here is an example of a code snippet that would raise this error:
Watch a video course Python - The Practical Guide
The error message will be:
In this example, the variable x is being accessed before it is assigned a value, which is causing the error. To fix this, you can either move the assignment of the variable x before the print statement, or give it an initial value before the print statement.
Both will work without any error.
Related Resources
- Using global variables in a function
- "Least Astonishment" and the Mutable Default Argument
- Why is "1000000000000000 in range(1000000000000001)" so fast in Python 3?
- HTML Basics
- Javascript Basics
- TypeScript Basics
- React Basics
- Angular Basics
- Sass Basics
- Vue.js Basics
- Python Basics
- Java Basics
- NodeJS Basics
Get the Reddit app
Subreddit for posting questions and asking for general advice about your python code.
having a problem with error code: UnboundLocalError: local variable 'player' referenced before assignment
hi hoping someone here can actually help me. I asked this question on stack overflow and they closed it and redirected to similar previously asked questions. none of which actually helped me to understand how to correct the error code.
I am following a video tutorial series for learning to code with python/pygame. and i have checked my coding aginst the source material and they are the same, because i typed it as was told to in the video.
here's the full tracebackback including the error:
File "/home/dev/PycharmProjects/game7_meteor_game/game7_meteor_game.py", line 160, in <module>
File "/home/dev/PycharmProjects/game7_meteor_game/game7_meteor_game.py", line 152, in main
GameInterface(num_player=1, screen=screen)
File "/home/dev/PycharmProjects/game7_meteor_game/game7_meteor_game.py", line 82, in GameInterface
if player.cooling_time > 0:
UnboundLocalError: local variable 'player' referenced before assignment.
here's the actual code:
if player.cooling_time > 0: player.cooling_time -= 1
UndboundLocalError: local variable referenced before assignment
Hello all, I’m using PsychoPy 2023.2.3 Win 10 x64bits

What I’m trying to do? The experiment will show in the middle of the screen an abstracted stimuli (B1 or B2), and after valid click on it, the stimulus will remain on the middle of the screen and three more stimuli will appear in the cornor of the screen.
I’m having this erro (attached above), a simple error, but I can not see where the error is. Also the experiment isn’t working proberly and is the old version (I don’t know but someone are having troubles with this version of PscyhoPy)? ba_training_block.xlsx (13.8 KB) SMTS.psyexp (91.6 KB) stimuli, instructions and parameters.xlsx (12.8 KB)
You have a routine called sample but you also use that name for your image file in sample_box .
I changed the name of the routine for ‘stimulus_sample’ and manteined the image file in sample_box as ‘sample’. But, the error still remain. But it do not happen all the time, this is very interesting…
Can u give it a look again? (I made some minor changes here)

Here the exp file ba_training_block.xlsx (13.7 KB) SMTS.psyexp (89.7 KB) stimuli, instructions and parameters.xlsx (12.8 KB)
Thanks again
Please could you confirm/show the new error message? Is it definitely still related to sample?

I think you have blank rows in your spreadsheet. The loop claims that there are 19 conditions but I think you only want 12. Without a value for sample_category sample doesn’t get set. With random presentation this will happen at a random point.
Related Topics
Topic | Replies | Views | Activity | |
---|---|---|---|---|
Builder | 10 | 4129 | February 9, 2024 | |
Builder | 2 | 175 | April 22, 2024 | |
Builder | 1 | 182 | October 17, 2023 | |
Builder | 2 | 518 | February 1, 2023 | |
Builder | 1 | 51 | June 6, 2024 |
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
UnboundLocalError: local variable referenced before assignment
I have following simple function to get percent values for different cover types from a raster. It gives me following error: UnboundLocalError: local variable 'a' referenced before assignment
which isn't clear to me. Any suggestions?
- arcgis-10.1
- unboundlocalerror
- 1 Because if row.getValue("Value") == 1 might be false and so a never gets assigned. – Nathan W Commented May 20, 2013 at 2:39
- It has value and do gets assigned. I checked it in arcmap interactive python window but can't get it to work in a stand alone script. – Ibe Commented May 20, 2013 at 2:44
- 1 your loop will also only give you the values of the last loop iteration as you are returning out of the loop and not doing anything with each value. – Nathan W Commented May 20, 2013 at 2:49
- You could use 3 x elif and an else to see if any values other than 1-4 are encountered. – PolyGeo ♦ Commented May 20, 2013 at 3:44
- I tried that way as well but still hung up with error. – Ibe Commented May 20, 2013 at 4:15
This error is pretty much explained here and it helped me to get assignments and return values for all variables.
Your Answer
Sign up or log in, post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged arcpy arcgis-10.1 unboundlocalerror or ask your own question .
- Featured on Meta
- Upcoming sign-up experiments related to tags
Hot Network Questions
- Short story about a group of astronauts/scientist that find a sentient planet that seems friendly but is not
- Meaning of あんたの今 here
- What does "the dogs of prescriptivism" mean?
- What is a "general" relation algebra?
- Visiting every digit
- The connection between determinants and eigenvalues
- What is the meaning of "Wa’al"?
- Medical - Must use corrective lens(es)…
- Binary Slashes Display
- How can I enable read only mode in microSD card
- if people are bred like dogs, what can be achieved?
- Scammed applying for ESTA, looking for travel options
- Is teaching how to solve recurrence relations using generating functions too much for a first year discrete maths course?
- Rewarding the finding of zeroes of a hash function
- Would a PhD from Europe, Canada, Australia, or New Zealand be accepted in the US?
- What should the next step be in this Kakuro puzzle?
- Why a battery has a limit usage of current in amps?
- Can commercial aircraft be equipped with ECM and ECCM equipment?
- A Boy Named Sue
- My 5-year-old is stealing food and lying
- Numerical approximation of the integral by using data
- How does MPPT maintain a constant voltage?
- HTTP: how likely are you to be compromised by using it just once?
- What is the purpose of the M1 pin on a Z80
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
UnboundLocalError: local variable 'Normalizer' referenced before assignment #173
wdyyyyyy commented Jun 1, 2024
|
The text was updated successfully, but these errors were encountered: |
jianchang512 commented Jun 1, 2024 • edited
查看 或 |
Sorry, something went wrong.
osahenr commented Jun 1, 2024
More light about this post please! |
wasimar commented Jun 1, 2024
fixed in (tested locally and Google Colab) |
lesonky commented Jun 3, 2024
Normalizer 有些问题,可以在调用 infer 的时候把 do_text_normalization=False 加进去,跳过 normalizer 这一步,等后续修复吧 |
- 👍 5 reactions
wdyyyyyy commented Jun 3, 2024
我现在可以跑了(系统是win10) |
我报错的原因是库没有装全,之后下载了pynini、WeTextProcessing等库就可以运行了。 |
- 👍 3 reactions
xiehuiqi220 commented Jun 7, 2024
(tested locally and Google Colab) no effect |
jianchang512 commented Jun 7, 2024
windows上只可使用 conda 方式才能正确安装,其他报错,最简单方式就是 chat.infer里传入 do_text_normalization=False |
wdyyyyyy commented Jun 8, 2024
是的,用pip显示安装失败,用conda就可以下载了 |
cucdengjunli commented Jun 17, 2024
mark |
Successfully merging a pull request may close this issue.
【Python】成功解决Python报错 UnboundLocalError: local variable ‘xxx‘ referenced before assignment问题
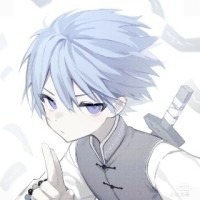
😎 作者介绍:我是程序员洲洲,一个热爱写作的非著名程序员。CSDN全栈优质领域创作者、华为云博客社区云享专家、阿里云博客社区专家博主。 🤓 同时欢迎大家关注其他专栏,我将分享Web前后端开发、人工智能、机器学习、深度学习从0到1系列文章。
在Python编程中,UnboundLocalError是一个运行时错误,它发生在尝试访问一个在当前作用域内未被绑定(即未被赋值)的局部变量时。 错误信息UnboundLocalError: local variable ‘xxx’ referenced before assignment指出变量xxx在赋值之前就被引用了。 这种情况通常发生在函数内部,尤其是在使用循环或条件语句时,变量的赋值逻辑可能因为某些条件未满足而未能执行,导致在后续的代码中访问了未初始化的变量。
我们来看看粉丝跟我说的具体的报错情况:
运行后会显示报错:UnboundLocalError: local variable ‘xxx’ referenced before assignment
把变量声明称global,global sum_score。
- 条件语句中未初始化变量
- 循环中变量初始化位置错误
- 循环的退出条件导致变量未初始化
- 确保变量在使用前被初始化
- 调整循环中变量的作用域
- 检查循环退出条件,确保变量被初始化
- 明确变量作用域:理解Python中变量的作用域,确保在变量的作用域内使用前已经初始化。
- 使用初始化值:为变量提供一个初始值,特别是在不确定变量是否会被赋值的情况下。
- 条件语句的使用:在条件语句中使用变量前,确保变量已经在所有分支中被初始化。
- 循环逻辑检查:在循环中使用变量前,确保循环的逻辑允许变量被正确初始化。
- 代码审查:定期进行代码审查,检查变量的使用是否符合预期,特别是变量初始化的逻辑。
- 编写测试:编写单元测试来验证函数或方法在所有预期的使用情况下都能正确处理变量初始化。
本文分享自 作者个人站点/博客 前往查看
如有侵权,请联系 [email protected] 删除。
本文参与 腾讯云自媒体同步曝光计划 ,欢迎热爱写作的你一起参与!
Copyright © 2013 - 2024 Tencent Cloud. All Rights Reserved. 腾讯云 版权所有
深圳市腾讯计算机系统有限公司 ICP备案/许可证号: 粤B2-20090059 深公网安备号 44030502008569
腾讯云计算(北京)有限责任公司 京ICP证150476号 | 京ICP备11018762号 | 京公网安备号11010802020287
Copyright © 2013 - 2024 Tencent Cloud.
All Rights Reserved. 腾讯云 版权所有
- Stack Overflow Public questions & answers
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Talent Build your employer brand
- Advertising Reach developers & technologists worldwide
- Labs The future of collective knowledge sharing
- About the company
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Python, Tkinter - UnboundLocalError: local variable 'Label' referenced before assignment?
I have just started to learn python & tkinter and during developing simple program, I'm receiving UnboundLocalError: local variable 'Label' referenced before assignment , while pressing Team Button. Individual button works as planned.
I don't understand how to solve this problem. My code:

- In the line Label = Label(root, text = "Entering as team or individual") what is the Label on the RHS of = sign? – Ritwik G Commented Mar 23, 2021 at 12:27
- 1 change Label = Label(...) to my_label = Label(...) – TheLizzard Commented Mar 23, 2021 at 12:32
- @RitwikG its the tkinter Label class imported on the line: from tkinter import * – TheLizzard Commented Mar 23, 2021 at 12:33
- Okay. I think the error could be since he is changing the Label variable as return value of Label(root, text = "Entering as team or individual") . @TheLizzard as you suggested making change should resolve the issue. @Student anyway try to add the error trace as it will clarify where exactly this error is thrown. – Ritwik G Commented Mar 23, 2021 at 12:37
- Your code is way too unpythonic (I tried and failed fixing it). You keep referencing Label (as a tkinter.Label object) before defining it. You have widgets overlapping. You have imports scattered around your program. You don't even use consistent white spacing. Your variable names aren't helpful at all. Why do you have global Tk ? – TheLizzard Commented Mar 23, 2021 at 12:48
When you do Label = Label(...) , python sees the Label = part and deduces that Label is a local variable since you didn't declare it global. Then, when python tries to do Label(...) , it still thinks Label is a local variable, but it hasn't been initialized yet so it throws local variable 'Label' referenced before assignment error.
The root of the problem is that you're using Label for a variable name, but Label has already been imported as a class name.
The fix is simple: don't create a variable named Label . Give it some other name.

Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged python python-3.x tkinter or ask your own question .
- Featured on Meta
- Upcoming sign-up experiments related to tags
- The return of Staging Ground to Stack Overflow
- Policy: Generative AI (e.g., ChatGPT) is banned
Hot Network Questions
- Episode of a sci-fi series about a salesman who loses the ability to understand English
- I did not contest a wrong but inconsequential accusation of plagiarism – can this come back to haunt me?
- What are some plausible means for increasing the atmospheric pressure on a post-apocalyptic earth?
- Cohen-Lenstra heuristics for ideal class groups over non-abelian extensions
- Is there some sort of kitchen utensil/device like a cylinder with a strainer?
- What rights does an employee retain, if any, who does not consent to being monitored on a work IT system?
- What is the explicit list of the situations that require RAII?
- Mechanistic view of the universe
- Bulls and Cows meet Sudoku!
- Vertical alignment of array inside array
- Travel from India to USA with close passport expiry
- Rewarding the finding of zeroes of a hash function
- HTTP: how likely are you to be compromised by using it just once?
- Numerical approximation of the integral by using data
- Chain slipping when under pressure
- Scammed applying for ESTA, looking for travel options
- Looking for a caveman discovers fire short story that is a pun on "nuclear" power
- Does Bluetooth not work on devices without GPS?
- Is the atomic sentence “There is something” the starting point for all possible human inquiry about anything at all?
- Meaning of あんたの今 here
- Are there substantive differences between the different approaches to "size issues" in category theory?
- Would a PhD from Europe, Canada, Australia, or New Zealand be accepted in the US?
- How to make Region from Spline curve
- if people are bred like dogs, what can be achieved?

IMAGES
VIDEO
COMMENTS
File "weird.py", line 5, in main. print f(3) UnboundLocalError: local variable 'f' referenced before assignment. Python sees the f is used as a local variable in [f for f in [1, 2, 3]], and decides that it is also a local variable in f(3). You could add a global f statement: def f(x): return x. def main():
Output. Hangup (SIGHUP) Traceback (most recent call last): File "Solution.py", line 7, in <module> example_function() File "Solution.py", line 4, in example_function x += 1 # Trying to modify global variable 'x' without declaring it as global UnboundLocalError: local variable 'x' referenced before assignment Solution for Local variable Referenced Before Assignment in Python
I can see that, but that variable can not be referenced before being set to some value ie. a dictionary or another object. Somewhere there needs to exist something like this rootent = to_something then you can use, well only if that object has function called get()
Therefore, we have examined the local variable referenced before the assignment Exception in Python. The differences between a local and global variable declaration have been explained, and multiple solutions regarding the issue have been provided.
The Python "UnboundLocalError: Local variable referenced before assignment" occurs when we reference a local variable before assigning a value to it in a function. To solve the error, mark the variable as global in the function definition, e.g. global my_var .
The UnboundLocalError: local variable 'x' referenced before assignment occurs when you reference a variable inside a function before declaring that variable. To resolve this error, you need to use a different variable name when referencing the existing variable, or you can also specify a parameter for the function. I hope this tutorial is useful.
Avoid Reassignment of Global Variables. Below, code calculates a new value (local_var) based on the global variable and then prints both the local and global variables separately.It demonstrates that the global variable is accessed directly without being reassigned within the function.
UnboundLocalError: local variable referenced before assignment. Example #1: Accessing a Local Variable. Solution #1: Passing Parameters to the Function. Solution #2: Use Global Keyword. Example #2: Function with if-elif statements. Solution #1: Include else statement. Solution #2: Use global keyword. Summary.
To fix this, you can either move the assignment of the variable x before the print statement, or give it an initial value before the print statement. def example (): x = 5 print (x) example()
The Unboundlocalerror: local variable referenced before assignment is raised when you try to use a variable before it has been assigned in the local context. Python doesn't have variable declarations , so it has to figure out the scope of variables itself. It does so by a simple rule: If there is an assignment to a variable inside a function ...
UnboundLocalError: local variable 'player' referenced before assignment. here's the actual code: if player.cooling_time > 0: player.cooling_time -= 1 Share Sort by: Best. Open comment sort options ... UnboundLocalError: local variable 'player' referenced before assignment Reply reply
UnboundLocalError: local variable 'a' referenced before assignment The text was updated successfully, but these errors were encountered: 👍 17 callensm, bendesign55, nulladdict, rohanbanerjee, balovbohdan, drewszurko, hellotuitu, rribani, jeromesteve202, egmaziero, and 7 more reacted with thumbs up emoji
And still are the same error: 1366×768 58.8 KB. wakecarter February 29, 2024, 8:54pm 6. I think you have blank rows in your spreadsheet. The loop claims that there are 19 conditions but I think you only want 12. Without a value for sample_category sample doesn't get set. With random presentation this will happen at a random point.
I have following simple function to get percent values for different cover types from a raster. It gives me following error: UnboundLocalError: local variable 'a' referenced before assignment whic...
In order for you to modify test1 while inside a function you will need to do define test1 as a global variable, for example: test1 = 0. def test_func(): global test1. test1 += 1. test_func() However, if you only need to read the global variable you can print it without using the keyword global, like so: test1 = 0.
UnboundLocalError: tokenizer_one referenced before assignment in train_dreambooth_lora_sd3.py #8594 Closed C0nsumption opened this issue Jun 16, 2024 · 3 comments · Fixed by #8600
UnboundLocalError: local variable 'all_files' referenced before assignment #691. HenryZhuHR opened this issue Apr 27, 2024 · 1 comment Comments. Copy link ... request_exception UnboundLocalError: local variable ' all_files ' referenced before assignment ...
UnboundLocalError: local variable 'Normalizer' referenced before assignment #173. Closed ... Closed UnboundLocalError: local variable 'Normalizer' referenced before assignment #173. wdyyyyyy opened this issue Jun 1, 2024 · 10 comments · Fixed by #420. Labels. bug Something isn't working. Comments. Copy link wdyyyyyy commented Jun 1, 2024.
I am trying to plot using seaborn boxplot, however I get the UnboundLocalError: local variable 'boxprops' referenced before assignment. These codes were executing fine last week. I have tried updat...
错误信息UnboundLocalError: local variable 'xxx' referenced before assignment指出变量xxx在赋值之前就被引用了。 这种情况通常发生在函数内部,尤其是在使用循环或条件语句时,变量的赋值逻辑可能因为某些条件未满足而未能执行,导致在后续的代码中访问了未初始化的 ...
So, you need to do this for the global variable a since you modify it inside addNumbers: def addNumbers(e): global a. # This is the same as: a = a + b. a += b. # You don't need str here. label1.configure(text=a) Here is a reference on the global keyword. Also, I would like to point out that your code can be improved if you use the command ...
UnboundLocalError: local variable referenced before assignment # Hot Network Questions Is there a fully combinatorial version of MLTT without lambda abstraction?
I have just started to learn python & tkinter and during developing simple program, I'm receiving UnboundLocalError: local variable 'Label' referenced before assignment, while pressing Team Button. Individual button works as planned. I don't understand how to solve this problem. My code: