Java Tutorial
Java methods, java classes, java file handling, java how to, java reference, java examples, java strings.
Strings are used for storing text.
A String variable contains a collection of characters surrounded by double quotes:
Create a variable of type String and assign it a value:
Try it Yourself »

String Length
A String in Java is actually an object, which contain methods that can perform certain operations on strings. For example, the length of a string can be found with the length() method:
More String Methods
There are many string methods available, for example toUpperCase() and toLowerCase() :
Finding a Character in a String
The indexOf() method returns the index (the position) of the first occurrence of a specified text in a string (including whitespace):
Java counts positions from zero. 0 is the first position in a string, 1 is the second, 2 is the third ...
Complete String Reference
For a complete reference of String methods, go to our Java String Methods Reference .
The reference contains descriptions and examples of all string methods.
Test Yourself With Exercises
Fill in the missing part to create a greeting variable of type String and assign it the value Hello .
Start the Exercise
Using the Java Keyword new
You can also create a String and assign values to it with the new keyword:
Strings in Java are objects, and an object in Java is declared with the new keyword. However, instead of using the new keyword, you can easily just write the string text inside double quotes. This is called a "String literal". It is up to you which method you would like to use. You will learn more about objects in a later chapter.

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Learn Java practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn java interactively, java introduction.
- Get Started With Java
- Your First Java Program
- Java Comments
Java Fundamentals
- Java Variables and Literals
- Java Data Types (Primitive)
- Java Operators
- Java Basic Input and Output
- Java Expressions, Statements and Blocks
Java Flow Control
- Java if...else Statement
- Java Ternary Operator
- Java for Loop
- Java for-each Loop
- Java while and do...while Loop
- Java break Statement
- Java continue Statement
- Java switch Statement
- Java Arrays
- Java Multidimensional Arrays
- Java Copy Arrays
Java OOP(I)
- Java Class and Objects
- Java Methods
- Java Method Overloading
- Java Constructors
- Java Static Keyword
Java Strings
- Java Access Modifiers
- Java this Keyword
- Java final keyword
- Java Recursion
- Java instanceof Operator
Java OOP(II)
- Java Inheritance
- Java Method Overriding
- Java Abstract Class and Abstract Methods
- Java Interface
- Java Polymorphism
- Java Encapsulation
Java OOP(III)
- Java Nested and Inner Class
- Java Nested Static Class
- Java Anonymous Class
- Java Singleton Class
- Java enum Constructor
- Java enum Strings
- Java Reflection
- Java Package
- Java Exception Handling
- Java Exceptions
- Java try...catch
- Java throw and throws
- Java catch Multiple Exceptions
- Java try-with-resources
- Java Annotations
- Java Annotation Types
- Java Logging
- Java Assertions
- Java Collections Framework
- Java Collection Interface
- Java ArrayList
- Java Vector
- Java Stack Class
- Java Queue Interface
- Java PriorityQueue
- Java Deque Interface
- Java LinkedList
- Java ArrayDeque
- Java BlockingQueue
- Java ArrayBlockingQueue
- Java LinkedBlockingQueue
- Java Map Interface
- Java HashMap
- Java LinkedHashMap
- Java WeakHashMap
- Java EnumMap
- Java SortedMap Interface
- Java NavigableMap Interface
- Java TreeMap
- Java ConcurrentMap Interface
- Java ConcurrentHashMap
- Java Set Interface
- Java HashSet Class
- Java EnumSet
- Java LinkedHashSet
- Java SortedSet Interface
- Java NavigableSet Interface
- Java TreeSet
- Java Algorithms
- Java Iterator Interface
- Java ListIterator Interface
Java I/o Streams
- Java I/O Streams
- Java InputStream Class
- Java OutputStream Class
- Java FileInputStream Class
- Java FileOutputStream Class
- Java ByteArrayInputStream Class
- Java ByteArrayOutputStream Class
- Java ObjectInputStream Class
- Java ObjectOutputStream Class
- Java BufferedInputStream Class
- Java BufferedOutputStream Class
- Java PrintStream Class
Java Reader/Writer
- Java File Class
- Java Reader Class
- Java Writer Class
- Java InputStreamReader Class
- Java OutputStreamWriter Class
- Java FileReader Class
- Java FileWriter Class
- Java BufferedReader
- Java BufferedWriter Class
- Java StringReader Class
- Java StringWriter Class
- Java PrintWriter Class
Additional Topics
- Java Keywords and Identifiers
- Java Operator Precedence
- Java Bitwise and Shift Operators
- Java Scanner Class
- Java Type Casting
- Java Wrapper Class
- Java autoboxing and unboxing
- Java Lambda Expressions
- Java Generics
- Nested Loop in Java
- Java Command-Line Arguments
Java Tutorials
Java String equals()
Java String intern()
Java String compareTo()
- Java String concat()
Java String equalsIgnoreCase()
- Java String compareToIgnoreCase()
In Java, a string is a sequence of characters. For example, "hello" is a string containing a sequence of characters 'h' , 'e' , 'l' , 'l' , and 'o' .
We use double quotes to represent a string in Java. For example,
Here, we have created a string variable named type . The variable is initialized with the string Java Programming .
Example: Create a String in Java
In the above example, we have created three strings named first , second , and third .
Here, we are directly creating strings like primitive types .
However, there is another way of creating Java strings (using the new keyword).
We will learn about that later in this tutorial.
Note : Strings in Java are not primitive types (like int , char , etc). Instead, all strings are objects of a predefined class named String .
And all string variables are instances of the String class.
Java String Operations
Java provides various string methods to perform different operations on strings. We will look into some of the commonly used string operations.
1. Get the Length of a String
To find the length of a string, we use the length() method. For example,
In the above example, the length() method calculates the total number of characters in the string and returns it.
To learn more, visit Java String length() .
2. Join Two Java Strings
We can join two strings in Java using the concat() method. For example,
In the above example, we have created two strings named first and second . Notice the statement,
Here, the concat() method joins the second string to the first string and assigns it to the joinedString variable.
We can also join two strings using the + operator in Java.
To learn more, visit Java String concat() .
3. Compare Two Strings
In Java, we can make comparisons between two strings using the equals() method. For example,
In the above example, we have created 3 strings named first , second , and third .
Here, we are using the equal() method to check if one string is equal to another.
The equals() method checks the content of strings while comparing them. To learn more, visit Java String equals() .
Note : We can also compare two strings using the == operator in Java. However, this approach is different than the equals() method. To learn more, visit Java String == vs equals() .
Escape Character in Java Strings
The escape character is used to escape some of the characters present inside a string.
Suppose we need to include double quotes inside a string.
Since strings are represented by double quotes , the compiler will treat "This is the " as the string. Hence, the above code will cause an error.
To solve this issue, we use the escape character \ in Java. For example,
Now escape characters tell the compiler to escape double quotes and read the whole text.
Java Strings are Immutable
In Java, strings are immutable . This means once we create a string, we cannot change that string.
To understand it more thoroughly, consider an example:
Here, we have created a string variable named example . The variable holds the string "Hello! " .
Now, suppose we want to change the string.
Here, we are using the concat() method to add another string "World" to the previous string.
It looks like we are able to change the value of the previous string. However, this is not true .
Let's see what has happened here:
- JVM takes the first string "Hello! "
- creates a new string by adding "World" to the first string
- assigns the new string "Hello! World" to the example variable
- The first string "Hello! " remains unchanged
Creating Strings Using the New Keyword
So far, we have created strings like primitive types in Java.
Since strings in Java are objects, we can create strings using the new keyword as well. For example,
In the above example, we have created a string name using the new keyword.
Here, when we create a string object, the String() constructor is invoked.
To learn more about constructors, visit Java Constructor .
Note : The String class provides various other constructors to create strings. To learn more, visit Java String (official Java documentation) .

Example: Create Java Strings Using the New Keyword
Create string using literals vs. new keyword.
Now that we know how strings are created using string literals and the new keyword, let's see what is the major difference between them.
In Java, the JVM maintains a string pool to store all of its strings inside the memory. The string pool helps in reusing the strings.
1. While creating strings using string literals,
Here, we are directly providing the value of the string ( Java ). Hence, the compiler first checks the string pool to see if the string already exists.
- If the string already exists , the new string is not created. Instead, the new reference, example points to the already existing string ( Java ).
- If the string doesn't exist , a new string ( Java) is created.
2. While creating strings using the new keyword,
Here, the value of the string is not directly provided. Hence, a new "Java" string is created even though "Java" is already present inside the memory pool.
- Methods of Java String
Besides those mentioned above, there are various string methods present in Java. Here are some of those methods:
Table of Contents
- Java String
- Create a String in Java
- Get Length of a String
- Join two Strings
- Compare two Strings
- Escape character in Strings
- Immutable Strings
- Creating strings using the new keyword
- String literals vs new keyword
Sorry about that.
Related Tutorials
Java Library
The Java Tutorials have been written for JDK 8. Examples and practices described in this page don't take advantage of improvements introduced in later releases and might use technology no longer available. See Java Language Changes for a summary of updated language features in Java SE 9 and subsequent releases. See JDK Release Notes for information about new features, enhancements, and removed or deprecated options for all JDK releases.
Strings, which are widely used in Java programming, are a sequence of characters. In the Java programming language, strings are objects.
The Java platform provides the String class to create and manipulate strings.
Creating Strings
The most direct way to create a string is to write:
In this case, "Hello world!" is a string literal a series of characters in your code that is enclosed in double quotes. Whenever it encounters a string literal in your code, the compiler creates a String object with its valuein this case, Hello world! .
As with any other object, you can create String objects by using the new keyword and a constructor. The String class has thirteen constructors that allow you to provide the initial value of the string using different sources, such as an array of characters:
The last line of this code snippet displays hello .
String Length
Methods used to obtain information about an object are known as accessor methods . One accessor method that you can use with strings is the length() method, which returns the number of characters contained in the string object. After the following two lines of code have been executed, len equals 17:
A palindrome is a word or sentence that is symmetricit is spelled the same forward and backward, ignoring case and punctuation. Here is a short and inefficient program to reverse a palindrome string. It invokes the String method charAt(i) , which returns the i th character in the string, counting from 0.
Running the program produces this output:
To accomplish the string reversal, the program had to convert the string to an array of characters (first for loop), reverse the array into a second array (second for loop), and then convert back to a string. The String class includes a method, getChars() , to convert a string, or a portion of a string, into an array of characters so we could replace the first for loop in the program above with
Concatenating Strings
The String class includes a method for concatenating two strings:
This returns a new string that is string1 with string2 added to it at the end.
You can also use the concat() method with string literals, as in:
Strings are more commonly concatenated with the + operator, as in
which results in
The + operator is widely used in print statements. For example:
which prints
Such a concatenation can be a mixture of any objects. For each object that is not a String , its toString() method is called to convert it to a String .
Breaking strings between lines using the + concatenation operator is, once again, very common in print statements.
Creating Format Strings
You have seen the use of the printf() and format() methods to print output with formatted numbers. The String class has an equivalent class method, format() , that returns a String object rather than a PrintStream object.
Using String's static format() method allows you to create a formatted string that you can reuse, as opposed to a one-time print statement. For example, instead of
you can write
About Oracle | Contact Us | Legal Notices | Terms of Use | Your Privacy Rights
Copyright © 1995, 2022 Oracle and/or its affiliates. All rights reserved.
- Java Tutorial
- What is Java?
- Installing the Java SDK
- Your First Java App
- Java Main Method
- Java Project Overview, Compilation and Execution
- Java Core Concepts
- Java Syntax
- Java Variables
- Java Data Types
- Java Math Operators and Math Class
- Java Arrays
Java String
- Java Operations
- Java if statements
- Java Ternary Operator
- Java switch Statements
- Java instanceof operator
- Java for Loops
- Java while Loops
- Java Classes
- Java Fields
- Java Methods
- Java Constructors
- Java Packages
- Java Access Modifiers
- Java Inheritance
- Java Nested Classes
- Java Record
- Java Abstract Classes
- Java Interfaces
- Java Interfaces vs. Abstract Classes
- Java Annotations
- Java Lambda Expressions
- Java Modules
- Java Scoped Assignment and Scoped Access
- Java Exercises
Compact Strings
Java string literals, escape characters, string literals as constants or singletons, java text block indentation, string concatenation performance, string length, searching in strings with indexof(), matching a string against a regular expression with matches(), equalsignorecase(), startswith() and endswith(), compareto(), trimming strings with trim(), replacefirst(), replaceall(), splitting strings with split(), converting numbers to strings with valueof(), converting objects to strings, getting characters and bytes, converting to uppercase and lowercase, string formatting, strip indentation, translate escape codes, additional methods.
The Java String data type can contain a sequence (string) of characters, like pearls on a string. Strings are how you work with text in Java. Once a Java String is created you can search inside it, create substrings from it, create new strings based on the first but with some parts replaced, plus many other things.
Internal String Representation
A Java String (before Java 9) is represented internally in the Java VM using bytes, encoded as UTF-16. UTF-16 uses 2 bytes to represent a single character. Thus, the characters of a Java String are represented using a char array.
UTF is a character encoding that can represent characters from a lot of different languages (alphabets). That is why it is necessary to use 2 bytes per character - to be able to represent all these different characters within a single string.
From Java 9 and forward, The Java VM can optimize strings using a new Java feature called compact strings . The compact strings feature lets the Java VM detect if a string only contains ISO-8859-1/Latin-1 characters. If it does, the String will only use 1 byte per character internally. The characters of a compact Java String can thus be represented by a byte array instead of a char array.
Whether a String can be represented as a compact string or not is detected when the string is created. A String is immutable once created - so this is safe to do.
Creating a String
Strings in Java are objects. Therefore you need to use the new operator to create a new Java String object. Here is a Java String instantiation (creation) example:
The text inside the quotes is the text the String object will contain.
Java has a shorter way of creating a new String:
Instead of passing the text "Hello World" as a parameter to the String constructor, you can just write the text itself inside the double quote characters. This is called a String literal. The Java compiler will internally figure out how to create a new Java String representing the given text.
Java Strings literals accepts a set of escape characters which are translated into special characters in the created String. These escape characters are:
Here is an example of creating a Java String using escape characters:
This String literal will result in a String that starts with a tab character and ends with a carriage return and a new line character.
If you use the same string (e.g. "Hello World" ) in other String variable declarations, the Java virtual machine may only create a single String instance in memory. The string literal thus becomes a de facto constant or singleton. The various different variables initialized to the same constant string will point to the same String instance in memory. Here is a Java String constant / singleton example:
In this case the Java virtual machine will make both myString1 and myString2 point to the same String object.
More precisely, objects representing Java String literals are obtained from a constant String pool which the Java virtual machine keeps internally. That means, that even classes from different projects compiled separately, but which are used in the same application may share constant String objects. The sharing happens at runtime. It is not a compile time feature.
If you want to be sure that two String variables point to separate String objects, use the new operator like this:
Even though the value (text) of the two Java Strings created is the same, the Java virtual machine will create two different objects in memory to represent them.
Java Text Blocks
Java text blocks , also known as Java multi line strings , is a feature that was added in Java 13 (in preview) which enables you to more easily declare String literals that span multiple lines in your Java code. To explain the Java text block syntax, look at this Java text block example:
Notice the two sets of delimiters ( """ ) on the first line and the last line. These 3 consecutive quote characters tell the Java compiler that this is a Java text block being declared.
Both sets of quote characters should be located on their own lines - above and below the actual text to be included in the text block. Only the text on the lines between the lines with the delimiter characters is being included in the resulting Java String.
In between the quote delimiters you can write a multi line String without the need to escape new line and quote characters. Here is a Java text block example illustrating the use of quote characters inside the text part of the text block declaration:
Notice the quote characters around the word "quotes". In a normal Java String literal you would have had to escape these characters, but this is not necessary inside of a Java text block. Not unless you want to include 3 consecutive quote characters as part of the text in the text block. Then you need to escape at least one of the quote characters, to enable the Java compiler to tell these characters apart from an end-of-text-block delimiter.
In the Java text block examples shown earlier, the text between the two lines with the 3 quote delimiters was indented to be located at the same place horizontally as the delimiters. In other words, the text between the delimiters start at the same horizontal position as the delimiters do. This done purely for code formatting reasons! We may not actually want all those indentation characters (spaces or tabs) to be part of the actual String created from this text block!
What actually happens is, that the Java compiler strips all of the indentation characters out of the String produced by the Java text block declaration. The way the Java compiler knows how many indentation characters to strip out is by looking at the last line of the text block - the line that contains the last 3 delimiter quote characters. The indentation of the quote characters on this last line determines how many indentation characters the Java compiler strips out of the text inside the text block. Here are 3 Java text block examples using different levels of indentation - controlled by the indentation of the last 3 delimiter quote characters:
Notice the different locations of the last 3 quote delimiter characters. In the first Java text block declared the end quote delimiter characters are located at the same indentation position as the text itself. This will result in all indentation characters being stripped out of the resulting Java String.
In the second example the last 3 quote delimiter characters are located 2 characters earlier (horizontally) than the text. This means that the Java compiler will leave 2 characters of indentation in the resulting Java String. The rest of the indentation characters will be stripped out.
In the last example the last 3 quote delimiter characters are located 4 characters earlier (horizontally) than the text inside the text block declaration. This will the Java compiler leave in 4 characters of indentation in the resulting Java String.
Here is the output printed from this Java text block indentation example:
As you can see, the resulting strings have different levels of indentation included.
As you have probably figured out by now - the difference in the start location of the last 3 delimiter characters and the leftmost character of the text inside the text block determines how much indentation is left inside the Java String produced by the Java text block declaration. In other words, the Java compiler will at the indentation of the text compared to the last 3 delimiter quote characters of the text block to determine the indentation to include.
Concatenating Strings
Concatenating Strings means appending one string to another. Strings in Java are immutable meaning they cannot be changed once created. Therefore, when concatenating two Java String objects to each other, the result is actually put into a third String object.
Here is a Java String concatenation example:
The content of the String referenced by the variable three will be Hello World ; The two other Strings objects are untouched.
When concatenating Strings you have to watch out for possible performance problems. Concatenating two Strings in Java will be translated by the Java compiler to something like this:
As you can see, a new StringBuilder is created, passing along the first String to its constructor, and the second String to its append() method, before finally calling the toString() method. This code actually creates two objects: A StringBuilder instance and a new String instance returned from the toString() method.
When executed by itself as a single statement, this extra object creation overhead is insignificant. When executed inside a loop, however, it is a different story.
Here is a loop containing the above type of String concatenation:
This code will be compiled into something similar to this:
Now, for every iteration in this loop a new StringBuilder is created. Additionally, a String object is created by the toString() method. This results in a small object instantiation overhead per iteration: One StringBuilder object and one String object. This by itself is not the real performance killer though. But something else related to the creation of these objects is.
Every time the new StringBuilder(result) code is executed, the StringBuilder constructor copies all characters from the result String into the StringBuilder . The more iterations the loop has, the bigger the result String grows. The bigger the result String grows, the longer it takes to copy the characters from it into a new StringBuilder , and again copy the characters from the StringBuilder into the temporary String created by the toString() method. In other words, the more iterations the slower each iteration becomes.
The fastest way of concatenating Strings is to create a StringBuilder once, and reuse the same instance inside the loop. Here is how that looks:
This code avoids both the StringBuilder and String object instantiations inside the loop, and therefore also avoids the two times copying of the characters, first into the StringBuilder and then into a String again.
You can obtain the length of a String using the length() method. The length of a String is the number of characters the String contains - not the number of bytes used to represent the String. Here is an example:
You can extract a part of a String. This is called a substring. You do so using the substring() method of the String class. Here is an example:
After this code is executed the substring variable will contain the string Hello .
The substring() method takes two parameters. The first is the character index of the first character to be included in the substring. The second is the index of the character after the last character to be included in the substring. Remember that. The parameters mean "from - including, to - excluding ". This can be a little confusing until you memorize it.
The first character in a String has index 0, the second character has index 1 etc. The last character in the string has has the index String.length() - 1 .
You can search for substrings in Strings using the indexOf() method. Here is an example:
The index variable will contain the value 6 after this code is executed. The indexOf() method returns the index of where the first character in the first matching substring is found. In this case the W of the matched substring World was found at index 6 .
If the substring is not found within the string, the indexOf() method returns -1 ;
There is a version of the indexOf() method that takes an index from which the search is to start. That way you can search through a string to find more than one occurrence of a substring. Here is an example:
This code searches through the string " is this good or is this bad? " for occurrences of the substring " is ". It does so using the indexOf(substring, index) method. The index parameter tells what character index in the String to start the search from. In this example the search is to start 1 character after the index where the previous occurrence was found. This makes sure that you do not just keep finding the same occurrence.
The output printed from this code would be:
The substring " is " is found in four places. Two times in the words "is", and two times inside the word " this ".
The Java String class also has a lastIndexOf() method which finds the last occurrence of a substring. Here is an example:
The output printed from this code would be 21 which is the index of the last occurrence of the substring " is ".
The Java String matches() method takes a regular expression as parameter, and returns true if the regular expression matches the string, and false if not.
Here is a matches() example:
Comparing Strings
Java Strings also have a set of methods used to compare Strings. These methods are:
- startsWith()
The equals() method tests if two Strings are exactly equal to each other. If they are, the equals() method returns true . If not, it returns false . Here is an example:
The two strings one and three are equal, but one is not equal to two or to four . The case of the characters must match exactly too, so lowercase characters are not equal to uppercase characters.
The output printed from the code above would be:
The String class also has a method called equalsIgnoreCase() which compares two strings but ignores the case of the characters. Thus, uppercase characters are considered to be equal to their lowercase equivalents.
The startsWith() and endsWith() methods check if the String starts with a certain substring. Here are a few examples:
This example creates a String and checks if it starts and ends with various substrings.
The first line (after the String declaration) checks if the String starts with the substring " This ". Since it does, the startsWith() method returns true.
The second line checks if the String starts with the substring " This " when starting the comparison from the character with index 5. The result is false, since the character at index 5 is " i ".
The third line checks if the String ends with the substring " code ". Since it does, the endsWith() method returns true .
The fourth line checks if the String ends with the substring " shower ". Since it does not, the endsWith() method returns false.
The compareTo() method compares the String to another String and returns an int telling whether this String is smaller, equal to or larger than the other String. If the String is earlier in sorting order than the other String, compareTo() returns a negative number. If the String is equal in sorting order to the other String, compareTo() returns 0. If the String is after the other String in sorting order, the compareTo() metod returns a positive number.
Here is an example:
This example compares the one String to two other Strings. The output printed from this code would be:
The numbers are negative because the one String is earlier in sorting order than the two other Strings.
The compareTo() method actually belongs to the Comparable interface. This interface is described in more detail in my tutorial about Sorting .
You should be aware that the compareTo() method may not work correctly for Strings in different languages than English. To sort Strings correctly in a specific language, use a Collator .
The Java String class contains a method called trim() which can trim a string object. By trim is meant to remove white space characters at the beginning and end of the string. White space characters include space, tab and new lines. Here is a Java String trim() example:
After executing this code the trimmed variable will point to a String instance with the value
The white space characters at the beginning and end of the String object have been removed. The white space character inside the String have not been touched. By inside is meant between the first and last non-white-space character.
The trim() method does not modify the String instance. Instead it returns a new Java String object which is equal to the String object it was created from, but with the white space in the beginning and end of the String removed.
The trim() method can be very useful to trim text typed into input fields by a user. For instance, the user may type in his or her name and accidentally put an extra space after the last word, or before the first word. The trim() method is an easy way to remove such extra white space characters.
Replacing Characters in Strings With replace()
The Java String class contains a method named replace() which can replace characters in a String. The replace() method does not actually replace characters in the existing String. Rather, it returns a new String instance which is equal to the String instance it was created from, but with the given characters replaced. Here is a Java String replace() example:
After executing this code the replaced variable will point to a String with the text:
The replace() method will replace all character matching the character passed as first parameter to the method, with the second character passed as parameter to the replace() method.
The Java String replaceFirst() method returns a new String with the first match of the regular expression passed as first parameter with the string value of the second parameter.
Here is a replaceFirst() example:
This example will return the string "one five three two one".
The Java String replaceAll() method returns a new String with all matches of the regular expression passed as first parameter with the string value of the second parameter.
Here is a replaceAll() example:
This example will return the string "one five three five one".
The Java String class contains a split() method which can be used to split a String into an array of String objects. Here is a Java String split() example:
After executing this Java code the occurrences array would contain the String instances:
The source String has been split on the a characters. The Strings returned do not contain the a characters. The a characters are considered delimiters to split the String by, and the delimiters are not returned in the resulting String array.
The parameter passed to the split() method is actually a Java regular expression . Regular expressions can be quite advanced. The regular expression above just matched all a characters. It even only matched lowercase a characters.
The String split() method exists in a version that takes a limit as a second parameter. Here is a Java String split() example using the limit parameter:
The limit parameter sets the maximum number of elements that can be in the returned array. If there are more matches of the regular expression in the String than the given limit , then the array will contain limit - 1 matches, and the last element will be the rest of the String from the last of the limit - 1 matches. So, in the example above the returned array would contain these two Strings:
The first String is a match of the a regular expression. The second String is the rest of the String after the first match.
Running the example with a limit of 3 instead of 2 would result in these Strings being returned in the resulting String array:
Notice how the last String still contains the a character in the middle. That is because this String represents the rest of the String after the last match (the a after ' n drove with ').
Running the example above with a limit of 4 or higher would result in only the Split strings being returned, since there are only 4 matches of the regular expression a in the String.
The Java String class contains a set of overloaded static methods named valueOf() which can be used to convert a number to a String. Here are some simple Java String valueOf() examples:
The Object class contains a method named toString() . Since all Java classes extends (inherits from) the Object class, all objects have a toString() method. This method can be used to create a String representation of the given object. Here is a Java toString() example:
Note: For the toString() method to return a sane String representation of the given object, the class of the object must have overridden the toString() method. If not, the default toString() method (inherited from the Object class) will get called. The default toString() method does not provide that much useful information. Many built-in Java classes have a sensible toString() method already.
It is possible to get a character at a certain index in a String using the charAt() method. Here is an example:
This code will print out:
since these are the characters located at index 0 and 3 in the String.
You can also get the byte representation of the String method using the getBytes() method. Here are two examples:
The first getBytes() call return a byte representation of the String using the default character set encoding on the machine. What the default character set is depends on the machine on which the code is executed. Therefore it is generally better to explicitly specify a character set to use to create the byte representation (as in the next line).
The second getBytes() call return a UTF-8 byte representation of the String.
You can convert Strings to uppercase and lowercase using the methods toUpperCase() and toLowerCase() . Here are two examples:
From Java 13 the Java String class got a new method named formatted() which can be used to return a formatted version of the String formatted() is called on. The formatted() method is only a preview feature that was added together with Java Text Blocks in Java 13, so we do not yet know if it will stay in. Here is an example of using the Java String formatted() method:
This example will first print out "Hello World" and then "Hello Jakob". The parameter values passed to formatted() will be inserted into the returned String at the %s location of the input String.
From Java 13 the Java String class got a new method named stripIndent() which can be used to strip out indentation, similarly to how indentation is stripped out of Java Text Blocks . The stripIndent() method is a preview feature, so we don't know if it will stay in Java yet. Here is an example of using the new Java String stripIndent() method:
The output printed from this example will be:
Notice how the first 3 characters of indentation on each line have been stripped out.
If the indentation is different on each line, the shortest indentation will be stripped out from each line. If, e.g. the last line in the input String was only indented 1 character, only 1 character would be strippe from the indentation of the other lines.
From Java 13 the Java String class got a new method called translateEscapes() which can translate escape codes that exist inside a String in the same way the Java compiler translates them. For now, the translateEscapes() is a preview feature, so it is not yet sure that it will stay in Java. Here is an example of using the Java String translateEscapes() method:
The escape character \\ is interpreted to mean a single \ character by the Java compiler, so the input String ends up containing a \n as 2 text characters, not a line break.
When calling the translateEscapes() method the \n part of the text will now be interpreted as a line break escape code.
The output printed from the above code will be:
Notice how the first line printed shows the \n as text, where as the second line interprets it as a line break.
The String class has several other useful methods than the ones described in this tutorial. You can find them all in the String JavaDoc.
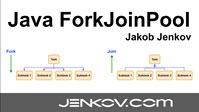
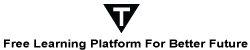
Java String
Java string methods.
- Why are String objects immutable?
- How to create an immutable class?
- What is string constant pool?
- What code is written by the compiler if you concatenate any string by + (string concatenation operator)?
- What is the difference between StringBuffer and StringBuilder class?
- Concept of String
- Immutable String
- String Comparison
- String Concatenation
- Concept of Substring
- String class methods and its usage
- StringBuffer class
- StringBuilder class
- Creating Immutable class
- toString() method
- StringTokenizer class

- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot
String Arrays in Java
- String class in Java
- Arrays in Java
- Strings in Java
- Set to Array in Java
- Array to Stream in Java
- C# | Arrays of Strings
- Array Literals in Java
- ArrayList in Java
- Arrays stream() method in Java
- Array of Strings in C
- Java | Arrays | Question 2
- Java | Arrays | Question 7
- Java | Arrays | Question 6
- Java | Arrays | Question 3
- Java | Arrays | Question 1
- Java | Arrays | Question 5
- Java | Arrays | Question 4
- Java | Arrays | Question 8
In programming, an array is a collection of the homogeneous types of data stored in a consecutive memory location and each data can be accessed using its index.
In the Java programming language, we have a String data type. The string is nothing but an object representing a sequence of char values. Strings are immutable in java. Immutable means strings cannot be modified in java.
When we create an array of type String in Java, it is called String Array in Java.
To use a String array, first, we need to declare and initialize it. There is more than one way available to do so.
Declaration:
The String array can be declared in the program without size or with size. Below is the code for the same –
In the above code, we have declared one String array (myString0) without the size and another one(myString1) with a size of 4. We can use both of these ways for the declaration of our String array in java.
Initialization:
In the first method , we are declaring the values at the same line. A second method is a short form of the first method and in the last method first, we are creating the String array with size after that we are storing data into it.
To iterate through a String array we can use a looping statement.
Time Complexity: O(N), where N is length of array. Auxiliary Space: O(1)
So generally we are having three ways to iterate over a string array. The first method is to use a for-each loop. The second method is using a simple for loop and the third method is to use a while loop. You can read more about iterating over array from Iterating over Arrays in Java
To find an element from the String Array we can use a simple linear search algorithm. Here is the implementation for the same –
In the above code, we have a String array that contains three elements Apple, Banana & Orange. Now we are searching for the Banana. Banana is present at index location 1 and that is our output.
Sorting of String array means to sort the elements in ascending or descending lexicographic order.
We can use the built-in sort() method to do so and we can also write our own sorting algorithm from scratch but for the simplicity of this article, we are using the built-in method.
Here our String array is in unsorted order, so after the sort operation the array is sorted in the same fashion we used to see on a dictionary or we can say in lexicographic order.
String array to String:
To convert from String array to String, we can use a toString() method.
Here the String array is converted into a string and it is stored into a string type variable but one thing to note here is that comma(,) and brackets are also present in the string. To create a string from a string array without them, we can use the below code snippet.
In the above code, we are having an object of the StringBuilder class. We are appending that for every element of the string array (myarr). After that, we are storing the content of the StringBuilder object as a string using the toString() method.
Please Login to comment...
Similar reads.
- Java-Strings
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

IMAGES
VIDEO
COMMENTS
String Length. A String in Java is actually an object, which contain methods that can perform certain operations on strings. For example, the length of a string can be found with the length() method: ... Fill in the missing part to create a greeting variable of type String and assign it the value Hello.
Ways of Creating a String. There are two ways to create a string in Java: String Literal. Using new Keyword. Syntax: <String_Type> <string_variable> = "<sequence_of_string>"; . 1. String literal. To make Java more memory efficient (because no new objects are created if it exists already in the string constant pool).
Note: The compound assignment operator in Java performs implicit type casting. Let's consider a scenario where x is an int variable with a value of 5. int x = 5; If you want to add the double value 4.5 to the integer variable x and print its value, there are two methods to achieve this: Method 1: x = x + 4.5. Method 2: x += 4.5.
You can also combine the arithmetic operators with the simple assignment operator to create compound assignments. For example, x+=1; and x=x+1; both increment the value of x by 1. The + operator can also be used for concatenating (joining) two strings together, as shown in the following ConcatDemo program:
In Java, a string is a sequence of characters. For example, "hello" is a string containing a sequence of characters 'h', 'e', 'l', 'l', and 'o'. We use double quotes to represent a string in Java. For example, // create a string String type = "Java programming"; Here, we have created a string variable named type.The variable is initialized with the string Java Programming.
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
Let's now create three empty Strings: String emptyLiteral = ""; String emptyNewString = new String(""); String emptyNewStringTwo = new String(); As we know by now, the emptyLiteral will be added to the String pool, while the other two go directly onto the heap. Although these won't be the same objects, all of them will have the same value:
Creating Strings. The most direct way to create a string is to write: String greeting = "Hello world!"; In this case, "Hello world!" is a string literal —a series of characters in your code that is enclosed in double quotes. Whenever it encounters a string literal in your code, the compiler creates a String object with its value—in this ...
Compound Assignment Operators. Sometime we need to modify the same variable value and reassigned it to a same reference variable. Java allows you to combine assignment and addition operators using a shorthand operator. For example, the preceding statement can be written as: i +=8; //This is same as i = i+8; The += is called the addition ...
The assignment operator is used to assign values to string objects. The size of the resulting string object will be the number of characters in the string value being assigned. The size of the resulting string object will be the number of characters in the string value being assigned.
To assign a value to a variable, use the basic assignment operator (=). It is the most fundamental assignment operator in Java. It assigns the value on the right side of the operator to the variable on the left side. Example: int x = 10; int x = 10; In the above example, the variable x is assigned the value 10.
In Java 7 that's in the Heap with other objects, but prior to that it was created in the Perm-Gen. String s = "hi"; String s1 = new String("hi"); new String("hi") creates a new String object on the heap, separate from the existing one. s and s1 are references to the two separate objects. References themselves actually live on the stack, even ...
The Java String matches() method takes a regular expression as parameter, and returns true if the regular expression matches the string, and false if not. Here is a matches() example: String text = "one two three two one"; boolean matches = text.matches(".*two.*"); Comparing Strings. Java Strings also have a set of methods used to compare Strings.
Learn different ways to concatenate Strings in Java. First up is the humble StringBuilder. This class provides an array of String-building utilities that makes easy work of String manipulation. Let's build a quick example of String concatenation using the StringBuilder class: StringBuilder stringBuilder = new StringBuilder(100); stringBuilder.append("Baeldung"); stringBuilder.append(" is ...
Java String literal is created by using double quotes. For Example: Each time you create a string literal, the JVM checks the "string constant pool" first. If the string already exists in the pool, a reference to the pooled instance is returned. If the string doesn't exist in the pool, a new string instance is created and placed in the pool.
This is Java doing typecasting to add the two numbers. String Concatenation. The += operator also works for string mutation. String a = "Hello"; a += "World"; System. out. println (a); Output. The string "Hello" has been mutated and the string "World" has been concatenated to it. Conclusion. The += is an important assignment operator ...
To use a String array, first, we need to declare and initialize it. There is more than one way available to do so. Declaration: The String array can be declared in the program without size or with size. Below is the code for the same -. String[] myString0; // without size. String[] myString1=new String[4]; //with size.
5. Write a Java program to compare two strings lexicographically. Two strings are lexicographically equal if they are the same length and contain the same characters in the same positions. Sample Output: String 1: This is Exercise 1 String 2: This is Exercise 2 "This is Exercise 1" is less than "This is Exercise 2".
I believe. :) FYI: alternatively, for unmodifiable list rather than simple array, List<String> list = List.of( "foo" , "bar" ) ; as a single line or two lines. normally you have to use new String[] { ...} (this was an always must in earlier versions of Java), but, as a syntactic sugar, the compiler accepts just { ... } in a field declaration or ...