16 Assign multiple variables
Using Tuples, it is possible to assign multiple values to multiple variables (either var or val ).
- Solarized Dark
- ◀ Prev
- Next ▶

Table of Contents
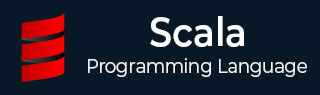
- Scala Tutorial
- Scala - Home
- Scala - Overview
- Scala - Environment Setup
- Scala - Basic Syntax
- Scala - Data Types
Scala - Variables
- Scala - Classes & Objects
- Scala - Access Modifiers
- Scala - Operators
- Scala - IF ELSE
- Scala - Loop Statements
- Scala - Functions
- Scala - Closures
- Scala - Strings
- Scala - Arrays
- Scala - Collections
- Scala - Traits
- Scala - Pattern Matching
- Scala - Regular Expressions
- Scala - Exception Handling
- Scala - Extractors
- Scala - Files I/O
- Scala Useful Resources
- Scala - Quick Guide
- Scala - Useful Resources
- Scala - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Variables are nothing but reserved memory locations to store values. This means that when you create a variable, you reserve some space in memory.
Based on the data type of a variable, the compiler allocates memory and decides what can be stored in the reserved memory. Therefore, by assigning different data types to variables, you can store integers, decimals, or characters in these variables.
Variable Declaration
Scala has a different syntax for declaring variables. They can be defined as value, i.e., constant or a variable. Here, myVar is declared using the keyword var. It is a variable that can change value and this is called mutable variable . Following is the syntax to define a variable using var keyword −
Here, myVal is declared using the keyword val. This means that it is a variable that cannot be changed and this is called immutable variable . Following is the syntax to define a variable using val keyword −
Variable Data Types
The type of a variable is specified after the variable name and before equals sign. You can define any type of Scala variable by mentioning its data type as follows −
If you do not assign any initial value to a variable, then it is valid as follows −
Variable Type Inference
When you assign an initial value to a variable, the Scala compiler can figure out the type of the variable based on the value assigned to it. This is called variable type inference. Therefore, you could write these variable declarations like this −
Here, by default, myVar will be Int type and myVal will become String type variable.
Multiple assignments
Scala supports multiple assignments. If a code block or method returns a Tuple ( Tuple − Holds collection of Objects of different types), the Tuple can be assigned to a val variable. [ Note − We will study Tuples in subsequent chapters.]
And the type inference gets it right −
Example Program
The following is an example program that explains the process of variable declaration in Scala. This program declares four variables — two variables are defined with type declaration and remaining two are without type declaration.
Save the above program in Demo.scala . The following commands are used to compile and execute this program.
Variable Scope
Variables in Scala can have three different scopes depending on the place where they are being used. They can exist as fields, as method parameters and as local variables. Below are the details about each type of scope.
Fields are variables that belong to an object. The fields are accessible from inside every method in the object. Fields can also be accessible outside the object depending on what access modifiers the field is declared with. Object fields can be both mutable and immutable types and can be defined using either var or val .

Method Parameters
Method parameters are variables, which are used to pass the value inside a method, when the method is called. Method parameters are only accessible from inside the method but the objects passed in may be accessible from the outside, if you have a reference to the object from outside the method. Method parameters are always immutable which are defined by val keyword.
Local Variables
Local variables are variables declared inside a method. Local variables are only accessible from inside the method, but the objects you create may escape the method if you return them from the method. Local variables can be both mutable and immutable types and can be defined using either var or val .
To Continue Learning Please Login

How to define Scala methods that return multiple items (tuples)
This is an excerpt from the Scala Cookbook (partially modified for the internet). This is Recipe 5.5, “How to define Scala methods and functions that returns multiple items (tuples).”
You want to return multiple values from a Scala function, but don’t want to wrap those values in an artificial, makeshift class.
Although you can return objects from methods just as in other OOP languages, Scala also lets you return multiple values from a method using tuples . To demonstrate this, first define a method that returns a tuple:
Then call that method and assign variable names to the expected return values, enclosing your variable names in parentheses:
Running this example in the REPL demonstrates how this works:
In Java, when it would be convenient to be able to return multiple values from a method, the typical workaround is to return those values in a one-off “wrapper” class. For instance, you might create a temporary wrapper class like this:
Then you could return an instance of this class from a method, like this:
In Scala you don’t need to create a wrapper like this; you can just return the data as a tuple.
Working with tuples
In the example shown in the Solution, the getStockInfo method returned a tuple with three elements, so it is a Tuple3 . Tuples can contain up to 22 variables and are implemented as Tuple1 through Tuple22 classes. As a practical matter, you don’t have to think about those specific classes; just create a new tuple by enclosing elements inside parentheses, as shown.
To demonstrate a Tuple2 , if you wanted to return only two elements from a method, just put two elements in the parentheses:
If you don’t want to assign variable names when calling the method, you can set a variable equal to the tuple the method returns, and then access the tuple values using the following tuple underscore syntax:
As shown, tuple values can be accessed by position as result._1 , result._2 , and so on. Though this approach can be useful in some situations, your code will generally be clearer if you assign variable names to the values:
- The Scala Tuple3 class
- Recipe 10.27, “Scala Tuples, for When You Just Need a Bag of Things” for more tuple examples.
Help keep this website running!
- How to write a Scala function that returns multiple values
- How to add exception annotations to Scala methods so they can be called from Java
- How to use import statements anywhere (methods, blocks) in Scala
- How to rename members on import in Scala
- Scala best practice: Create methods that have no side effects (pure functions)
books by alvin
- ZIO ZLayer: A simple “Hello, world” example (dependency injection, services)
- A dream vacation for the meditator in your life
- Al's Oasis
- Window of the Poet (painting)
- Shinzen Young on meditating in his daily life: arising, disappearing, and The Source, and love

⚠️ Beware of Scams : since Feb 2024, scammers are using fake Scala websites to sell courses , please check you are using an official source.
- Auxiliary Class Constructors
Outdated Notice
This page has a new version.
Info: JavaScript is currently disabled, code tabs will still work, but preferences will not be remembered.
You define auxiliary Scala class constructors by defining methods that are named this . There are only a few rules to know:
- Each auxiliary constructor must have a different signature (different parameter lists)
- Each constructor must call one of the previously defined constructors
Here’s an example of a Pizza class that defines multiple constructors:
With all of those constructors defined, you can create pizza instances in several different ways:
We encourage you to paste that class and those examples into the Scala REPL to see how they work.
There are two important notes to make about this example:
- The DefaultCrustSize and DefaultCrustType variables are not a preferred way to handle this situation, but because we haven’t shown how to handle enumerations yet, we use this approach to keep things simple.
- Auxiliary class constructors are a great feature, but because you can use default values for constructor parameters, you won’t need to use this feature very often. The next lesson demonstrates how using default parameter values like this often makes auxiliary constructors unnecessary:
Contributors to this page:
- Introduction
- Prelude꞉ A Taste of Scala
- Preliminaries
- Scala Features
- Hello, World
- Hello, World - Version 2
- The Scala REPL
- Two Types of Variables
- The Type is Optional
- A Few Built-In Types
- Two Notes About Strings
- Command-Line I/O
- Control Structures
- The if/then/else Construct
- for Expressions
- match Expressions
- try/catch/finally Expressions
- Scala Classes
- Supplying Default Values for Constructor Parameters
- A First Look at Scala Methods
- Enumerations (and a Complete Pizza Class)
- Scala Traits and Abstract Classes
- Using Scala Traits as Interfaces
- Using Scala Traits Like Abstract Classes
- Abstract Classes
- Scala Collections
- The ArrayBuffer Class
- The List Class
- The Vector Class
- The Map Class
- The Set Class
- Anonymous Functions
- Common Sequence Methods
- Common Map Methods
- A Few Miscellaneous Items
- An OOP Example
- sbt and ScalaTest
- The most used scala build tool (sbt)
- Using ScalaTest with sbt
- Writing BDD Style Tests with ScalaTest and sbt
- Functional Programming
- Pure Functions
- Passing Functions Around
- No Null Values
- Companion Objects
- Case Classes
- Case Objects
- Functional Error Handling in Scala
- Concurrency
- Scala Futures
- Where To Go Next
- Using Global Human Resources
Business Title Defaulting for Assignment in Employment Flows
The assignment is identified with the business title and the title is defaulted from the job or position in responsive employment flows using the ORA_PER_EMPL_DEFAULT_BUSINESS_TITLE_FROM profile option code.
The business title for the Mass Legal Employer Change flow is defaulted from the source assignment irrespective of the profile option.
The business title for the Convert Pending Worker flows (Convert, Quick Convert, and ESS process) is defaulted from the source pending worker assignment irrespective of the profile option.
This table shows how the business title is defaulted based on the profile option:
This table shows the application behavior in the responsive employment flows based on the value configured for the profile option:
Display of Business Title for Multiple Assignments
You can identify and select the appropriate assignment by viewing assignment attributes in the Business Title list of values (LOV) on the Employment Info page. Additionally, you can identify the required assignment in document records by configuring additional assignment attributes to display in the Business Title LoV. This is the order of the attributes available in the Business Title LOV:
Business title
Assignment number
Legal employer
Worker type
Location (shows only if the value exists in the assignment)
HR status (shows only if the assignment is suspended or inactive)
The attributes in the LOV are sorted first based on the HR status (in ascending order of Active, Inactive, or Suspended) followed by the assignment creation date (in descending order of the latest date first).
For example, this is how the attributes look for a worker with an active assignment, suspended assignment, or inactive assignment (with no location):
For an active assignment: Manager Information Systems; E10101010; Vision Corporation; Employee; Alberta, CA
For a suspended assignment: Management Consultant; E10101010-1; Vision Corporation; Employee; Alberta, CA; Suspended - Payroll Eligible
For an inactive assignment: Management Trainer; E10101010-2; Vision Corporation; Employee; Inactive - No Payroll
- Set the ORA_PER_EMPL_ENABLE_WRK_ASG_REST_LOV profile option to Y.
- Use the Search Configuration in HCM Experience Design Studio to configure the Worker Assignment LoV.
- Displayed on the Employment Info page, only when the selected worker has more than 1 assignment.
- Lists assignments which are in any of these HR Statuses: Active, Suspended, Inactive.
- Lists all assignment types except the Offer assignment.
- Supports assignment-level security and lists only those assignments for the selected worker for which the logged in user has access.
- Active primary (Primary_Flag = Y, Primary_Assignment_Flag = Y)
- Active primary (Primary_Flag = N, Primary_Assignment_Flag = Y) Internal order by Assignment Creation Date
- Active non-primary (Primary_Flag = N, Primary_Assignment_Flag = N) Internal order by Assignment Creation Date
- Suspended primary (Primary_Flag = Y, Primary_Assignment_Flag = Y) Internal order by Assignment Creation Date
- Suspended primary (Primary_Flag = N, Primary_Assignment_Flag = Y) Internal order by Assignment Creation Date
- Suspended non-primary (Primary_Flag = N, Primary_Assignment_Flag = N) Internal order by Assignment Creation Date
- Inactive - Internal order by Assignment Creation Date
Related Topics
- Employment Profile Options

IMAGES
VIDEO
COMMENTS
The interpretation of an assignment to a simple variable x = e depends on the definition of x. If x denotes a mutable variable, then the assignment changes the current value of x to be the result of evaluating the expression e. (first, rest) here is a pattern, not a simple variable, so it works in the variable definition but not in the assignment.
If you really want to have some fun, you can take advantage of Scala's extractor functionality to declare fields with different types like this: scala> var (x, y, z) = (0, 1.1, "foo") x: Int = 0. y: Double = 1.1. z: java.lang.String = foo. As you can see from the output, that example creates three var fields of different types in one line of ...
The multiple assignments can be possible in Scala in one line by using 'val' keyword with variable name separated by commas and the "=" sign with the value for the variable. val a, b, c = 1; In the above example, you can see all of the variables a,b, and c get assigned to value 1 as follows.
Scala Tutorials - Basic Scala Tutorial. About; Tweet; 16 Assign multiple variables. Using Tuples, it is possible to assign multiple values to multiple variables (either var or val). Theme Monokai; Solarized Dark; Eclipse; Code editor is using Scalakata.com written by Guillaume Massé ...
Scala Variable Data Types. We can specify a variable's type after a colon after its name. Take an example: val x:Int=7 val x:Int Multiple Assignments. How do we fit multiple assignment statements into one statement? We assign a Tuple to a val variable. val (x: Int, y: String) = Pair(7, "Ayushi") val (x, y) = Pair(7, "Ayushi") Scala Variable Scope
1) In Scala, multiple parameters and multiple parameter lists are specified and implemented directly, as part of the language, rather being derived from single-parameter functions. 2) There is danger of confusion with the Scala standard library's curried and uncurried methods, which don't involve multiple parameter lists at all. Regardless ...
Everything is an object in Scala, so we can assign a function to a value: val inc = (number: Int) => number + 1. Copy. Value inc now contains a function. We can use this value everywhere we need to call the unit of code defined in function: scala> println(inc( 10 )) 11. Copy.
Multiple assignments. Scala supports multiple assignments. If a code block or method returns a Tuple (Tuple − Holds collection of Objects of different types), the Tuple can be assigned to a val variable. [Note − We will study Tuples in subsequent chapters.] Syntax val (myVar1: Int, myVar2: String) = Pair(40, "Foo")
Scala supports multiple assignments. If a code block or method returns a Tuple (Tuple − Holds collection of Objects of different types), the Tuple can be assigned to a val variable. [Note − We will study Tuples in subsequent chapters.] Syntax val (myVar1: Int, myVar2: String) = Pair(40, "Foo")
if expressions always return a result. A great thing about the Scala if construct is that it always returns a result. You can ignore the result as we did in the previous examples, but a more common approach — especially in functional programming — is to assign the result to a variable:
As shown, with a match expression you write a number of case statements that you use to match possible values. In this example we match the integer values 1 through 12.Any other value falls down to the _ case, which is the catch-all, default case.. match expressions are nice because they also return values, so rather than directly printing a string as in that example, you can assign the string ...
Introduction This proposal discusses the syntax and semantics of a construct to assign multiple variables with a single expression. This feature would simplify the implementation of operations expressed in terms of relationships between multiple variables, such as std::swap in C++. Motivation It happens that one has to assign multiple variables "at once" in an algorithm. For example, let ...
This is an excerpt from the Scala Cookbook (partially modified for the internet). This is Recipe 5.5, "How to define Scala methods and functions that returns multiple items (tuples)." Problem. You want to return multiple values from a Scala function, but don't want to wrap those values in an artificial, makeshift class.. Solution
1. Overview. Pattern matching is a powerful feature of the Scala language. It allows for more concise and readable code while at the same time providing the ability to match elements against complex patterns. In this tutorial, we'll discover how to use pattern matching in general and how we can benefit from it.
Just to clarify, when I say multiple assigment, parallel assignment, destructuring bind I mean the following pattern matching gem scala> val (x,y) = Tuple2("one",1) x: java.lang.String = one y: Int = 1
Multiple assignment of non-tuples in scala . Arrays Just to clarify, when I say multiple assigment, parallel assignment, destructuring bind I mean the following pattern matching gem scala> val (x,y) = Tuple2("one",1)x: java.lang.String = oney: Int = 1. which assigns "one" to x and 1 to y.
Here's an example of a Pizza class that defines multiple constructors: val DefaultCrustSize = 12 val DefaultCrustType = "THIN" // the primary constructor class Pizza (var crustSize: ... We encourage you to paste that class and those examples into the Scala REPL to see how they work. Notes.
Multiple assignment in Scala without using Array? 3. Assignments in Scala. 1. Function assignment to value/variable in Scala. 1. Multiple constants assignment in Scala. 5. Assign same value to multiple variables in Scala. Hot Network Questions Live-action Norwegian movie about man whose job is to monitor troll migration
Let's see some examples of assignments that need multiple managers. Matrix Management in an Engineering Company. An engineering company uses a matrix management structure. An engineer reports to the lead engineer for everyday functional guidance and to the operational manager for project assignment and tracking.
Multiple assignment of non-tuples in scala. 6. Working with tuples in Scala. 9. scala multiple assignment efficiency. 0. multi-assignment on a Seq[Tuple2] 1. Scala nested collection assignment. 1. Collection of variable-length Tuples AND map with multiple values TO weighted combinations. 0.
Display of Business Title for Multiple Assignments. You can identify and select the appropriate assignment by viewing assignment attributes in the Business Title list of values (LOV) on the Employment Info page. Additionally, you can identify the required assignment in document records by configuring additional assignment attributes to display ...